The Difference Between Operators is and as in Kotlin
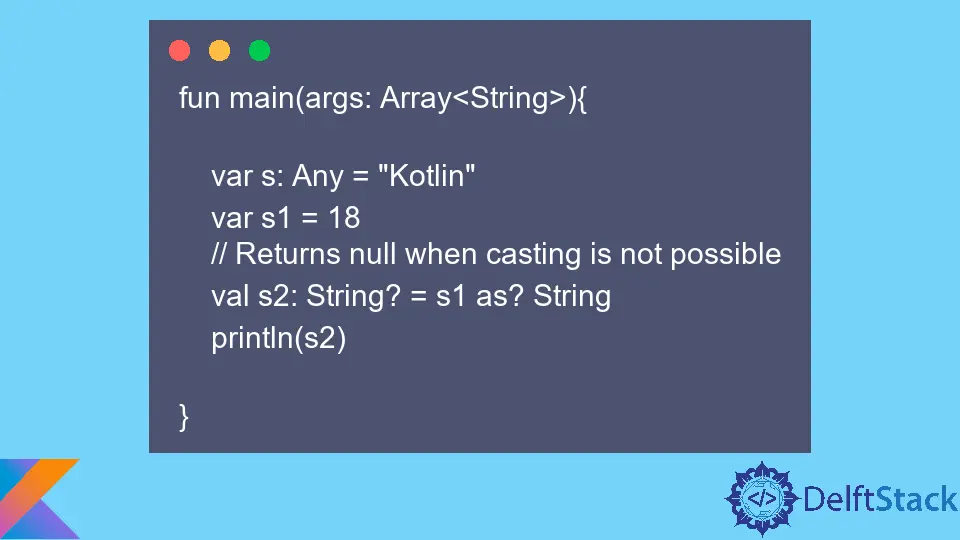
This article introduces the difference between the is
and as
operators in Kotlin. While the use of these operators might seem similar, they are different.
Kotlin is
Operator
The Kotlin is
operator is used for type checking. Suppose we have multiple classes with different variables in an interface, and we want to use the public variables interchangeably with different methods.
In that case, we will have to check whether the data type of the parameters required in both methods is similar. The code will throw an error if the data type is not similar.
For instance, if one method requires a parameter of data type String
, we need to check the data type of the variable we are passing. Kotlin is
operator allows us to do this.
It returns a Boolean value, and if both the operands of the is
operator has the same data type, it returns true
. Otherwise, it returns false
.
The Kotlin is
operator is a type checking operator. Hence, we can use it to check if the data type of a variable is what we think it is.
Code Example:
fun main(args: Array<String>) {
var s: Any = "Hey, this is Kotlin programming!"
val r = s is String
println("The variable s is a String: " + r)
}
Output:
The variable s is a String: true
The Kotlin is
operator is equivalent to Java’s instanceof
operator. Hence, we can use it to check a class’s object.
Code Example:
class exampleClass {
var v: String = "Kotlin Programming!"
fun exampleFunc() {
println("Official Android language is - " + v)
}
}
fun main(args: Array<String>) {
val obj = exampleClass() // Creating an object
obj.exampleFunc()
val result = obj is exampleClass // Using the Kotlin is operator
println("The object obj is an instance of the class exampleClass: " + result)
}
Output:
Official Android language is - Kotlin Programming!
The object obj is an instance of the class exampleClass: true
Kotlin as
Operator
The Kotlin as
operator is used for explicit type casting. The Kotlin as
is not a run-time safe operator.
If we pass a wrong object accidentally, the as
operator will lead to an error. Hence, it is always advised to use Kotlin’s safe cast operator as?
.
The as?
operator will return the casted value if possible. Otherwise, it will return a null
.
Use Unsafe Cast Operator as
The unsafe cast operator as
will work fine until we pass an operand that can be converted to the target type.
Code Example:
fun main(args: Array<String>){
val s: String = "Convertible"
val s1: String = s as String
println(s1)
}
Convertible
But when we try to convert something incompatible with the target type, it will throw an error.
Code Example:
fun main(args: Array<String>){
val s: String = 18
val s1: String = s as String
println(s1)
}
Error Output:
The safe cast operator as?
is best to avoid the error.
Use Safe Cast Operator As?
As mentioned earlier, the safe cast operator will return null
when the type casting is impossible.
fun main(args: Array<String>){
var s: Any = "Kotlin"
var s1 = 18
// Returns null when casting is not possible
val s2: String? = s1 as? String
println(s2)
}
Output:
null
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn