How to Difference Between !! And ? Operators in Kotlin
- the Concept of Null Safety
-
Null Safety Operator
?
in Kotlin -
Not-Null Assertion
!!
Operator in Kotlin
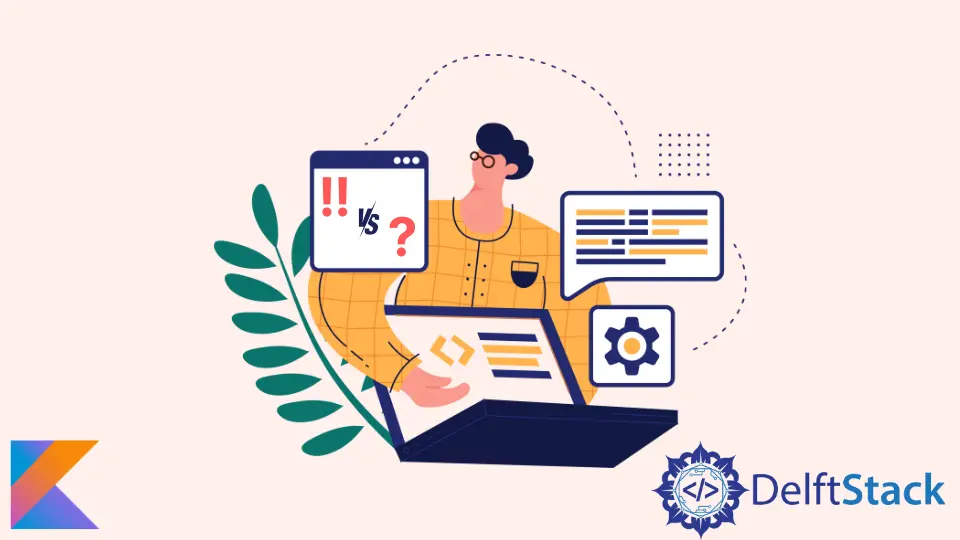
In Kotlin, the assertion operator !!
and the safe call operator ?
work with null safety.
This article introduces the concept of null safety. We will also go through how !!
and ?
in Kotlin helps with null safety.
the Concept of Null Safety
Kotlin’s type system aims to remove the null reference problem. The issue is that in many programming languages with the null concept, accessing a null reference member leads to an exception.
In Kotlin, this exception is called the NullPointerException
, or NPE. The type system in Kotlin aims to overcome the null reference problem through null safety.
The system distinguishes references that can and cannot hold null references. For instance, a standard String variable cannot hold null
, but String?
can.
Here are the examples to demonstrate the same.
The first example uses a standard variable, which means a non-null reference.
fun main() {
var s: String = "Hello" // Regular initialization means a non-null value
println(s)
}
Output:
If we try to use null
with a standard variable, it throws an error.
fun main() {
var s: String = null
println(s)
}
Output:
The second example uses String?
, which can be null
.
fun main() {
var s: String? = null // we can set this to null
print(s)
}
Output:
As we can see, only String?
can hold null
. Hence, if it’s a standard variable initialization, Kotlin’s type system can understand that it will not have a null
value.
So you can quickly call such a reference without worrying about NPE.
But what about the variables that can hold null
? We cannot access those variables directly as they are not NPE safe.
fun main() {
var s: String? = null // we can set this to null
print(s)
val l = s.length // This throws error as 's' can be null
}
Output:
But then, how do you access such variables? And the answer is through ?
and !!
operators.
Null Safety Operator ?
in Kotlin
One way to access null reference members is with the help of ?
. Using this operator returns the value if the variable is not null and null
otherwise.
Let’s consider the examples below to understand this.
We will use a non-null value for the first example and get its length.
Example 1:
fun main() {
var s: String? = "Hello"
println(s)
val l = s?.length
print(l)
}
Output:
Now, we will initialize the variable as null
.
Example 2:
fun main() {
var s: String? = null
println(s)
val l = s?.length
print(l)
}
Output:
The safe operator ?
is also helpful in creating chains.
For example, consider an employee of a company. He might have a team leader who will be working under a manager.
We can use ?
to create a chain and access the name of David’s manager in the following way:
employeename?.teamleader?.manager?.name
We can read the above syntax as:
- If the
employeename
is not null, return the department. - If the
department
is not null, return the manager. - If the
manager
is not null, return the name.
If any of the variables are null
, the syntax will return null
.
Not-Null Assertion !!
Operator in Kotlin
Another option to access null reference members is using the !!
operator. It is for NPE lovers.
So if you want the action of accessing non-null reference members to either give the value if there is or else throw NPE, you can use this operator in Kotlin.
Let’s consider the same two examples we did for the safe ?
operator to understand how the not-null assertion !!
operator works.
Example 1:
fun main() {
var s: String? = "Hello"
println(s)
val l = s!!.length
print(l)
}
Output:
Example 2:
fun main() {
var s: String? = null
println(s)
val l = s!!.length
print(l)
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn