How to Toggle Visibility in jQuery
- jQuery Toggle Visibility
-
Use the
show()
andhide()
Methods to Toggle the Visibility of an Element in jQuery -
Use the
css()
Method to Toggle the Visibility of an Element in jQuery
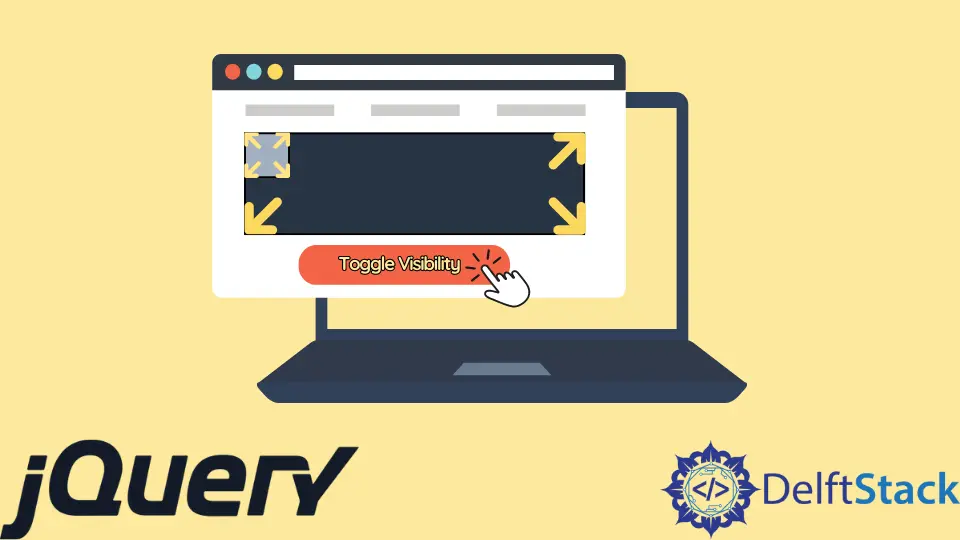
This tutorial demonstrates how to toggle the visibility of an element using jQuery.
jQuery Toggle Visibility
We can toggle the visibility of an HTML element using the jQuery method toggle()
. This implements both show()
and hide()
methods to implement the toggle functionality.
The syntax for this method is:
toggle( speed, [callback])
As we can see, the method takes two parameters. Both are optional, where the speed
is the speed of the toggle animation having three string values slow
, normal
, or fast
, or any integer value in milliseconds, and the callback
is the function that can be called once the animation is completed.
Let’s try a simple example with both optional parameters:
<!doctype html>
<html lang="en">
<head>
<title>jQuery toggle() method</title>
<script src = "https://code.jquery.com/jquery-3.6.0.js"></script>
<script>
$(document).ready(function() {
$("#toggle").click(function(){
$("#toggleDiv").toggle( 'slow', function(){
$("#Info").text('The visibility toggle is performed');
});
});
});
</script>
<style>
#toggleDiv {
border: 5px solid green;
background-color : lightblue;
height: 10%;
width: 20%;
}
</style>
</head>
<body>
<button id = "toggle"> Toggle the Element </button>
<div id = "toggleDiv">
<h1>Delftstack</h1>
</div>
<div id = "Info"></div>
</body>
</html>
The code above will toggle the given div
element on every button click and show the info once the toggle is completed.
See the output:
Use the show()
and hide()
Methods to Toggle the Visibility of an Element in jQuery
jQuery also provides methods show()
and hide()
to perform individual operations. We can also implement these methods together to toggle an element in jQuery.
The syntax for these methods is:
$(selector).hide(speed,callback);
$(selector).show(speed,callback);
The speed
and callback
are the same parameters as the toggle()
method. Let’s try a simple toggle example using these methods:
<!doctype html>
<html lang="en">
<head>
<title>jQuery toggle with Show Hide methods</title>
<script src = "https://code.jquery.com/jquery-3.6.0.js"></script>
<style>
#Demobox1 {
background: lightgreen;
width: 150px;
height: 150px;
margin-bottom:
10px;
}
#Demobox2 {
background: lightblue;
width: 150px;
height: 150px;
margin-bottom:
10px;
}
#Demobox3{
background: pink;
width: 150px;
height: 150px;
margin-bottom:
10px;
}
</style>
</head>
<body>
<div id="div1">
<div id="Demobox1"><h1>1</h1></div>
<div id="Demobox2"><h1>2</h1></div>
<div id="Demobox3"><h1>3</h1></div>
<button id="Click1">Toggle Box 1 visibility</b>
<button id="Click2">Toggle Box 2 visibility</b>
<button id="Click3">Toggle Box 3 visibility</b>
</div>
<script>
$("#Click1").click(function(){
if ( $("#Demobox1:visible").length ){
$("#Demobox1").hide("slow");
} else {
$("#Demobox1").show("slow");
}
});
$("#Click2").click(function(){
if ( $("#Demobox2:visible").length ){
$("#Demobox2").hide("slow");
} else {
$("#Demobox2").show("slow");
}
});
$("#Click3").click(function(){
if ( $("#Demobox3:visible").length ){
$("#Demobox3").hide("slow");
} else {
$("#Demobox3").show("slow");
}
});
</script>
</body>
</html>
The code above will work similarly to the toggle()
method. See the output:
Use the css()
Method to Toggle the Visibility of an Element in jQuery
We can also use the jQuery method css()
to toggle the visibility of an element. To perform that, we have to create conditions so that if the div
’s visibility is hidden, then set it to visible and vice versa.
The syntax for the css()
method is:
$("#Demobox1").css("visibility","hidden");
$("#Demobox1").css("visibility","visible");
If the visibility is hidden, set it to visible. Otherwise, if it is visible, set it to hidden.
Let’s try an example:
<!doctype html>
<html lang="en">
<head>
<title>jQuery toggle with Css method</title>
<script src = "https://code.jquery.com/jquery-3.6.0.js"></script>
<style>
#Demobox1 {
background: lightgreen;
width: 150px;
height: 150px;
margin-bottom:
10px;
}
#Demobox2 {
background: lightblue;
width: 150px;
height: 150px;
margin-bottom:
10px;
}
#Demobox3{
background: pink;
width: 150px;
height: 150px;
margin-bottom:
10px;
}
</style>
</head>
<body>
<div id="div1">
<div id="Demobox1"><h1>1</h1></div>
<div id="Demobox2"><h1>2</h1></div>
<div id="Demobox3"><h1>3</h1></div>
<button id="Click1">Toggle Box 1 visibility</b>
<button id="Click2">Toggle Box 2 visibility</b>
<button id="Click3">Toggle Box 3 visibility</b>
</div>
<script>
$("#Click1").click(function(){
if ( $("#Demobox1").css("visibility") == "visible" ){
$("#Demobox1").css("visibility","hidden");
} else {
$("#Demobox1").css("visibility","visible");
}
});
$("#Click2").click(function(){
if ( $("#Demobox2").css("visibility") == "visible" ){
$("#Demobox2").css("visibility","hidden");
} else {
$("#Demobox2").css("visibility","visible");
}
});
$("#Click3").click(function(){
if ( $("#Demobox3").css("visibility") == "visible" ){
$("#Demobox3").css("visibility","hidden");
} else {
$("#Demobox3").css("visibility","visible");
}
});
</script>
</body>
</html>
The code above contains three different divs
for which the visibility can be toggled using the different buttons by setting the CSS visibility property.
See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook