How to Set and Delete a Cookie in jQuery
-
Create and Delete a Cookie Using
jquery-cookie
in jQuery - Create and Delete a Cookie Using a Custom Function in jQuery
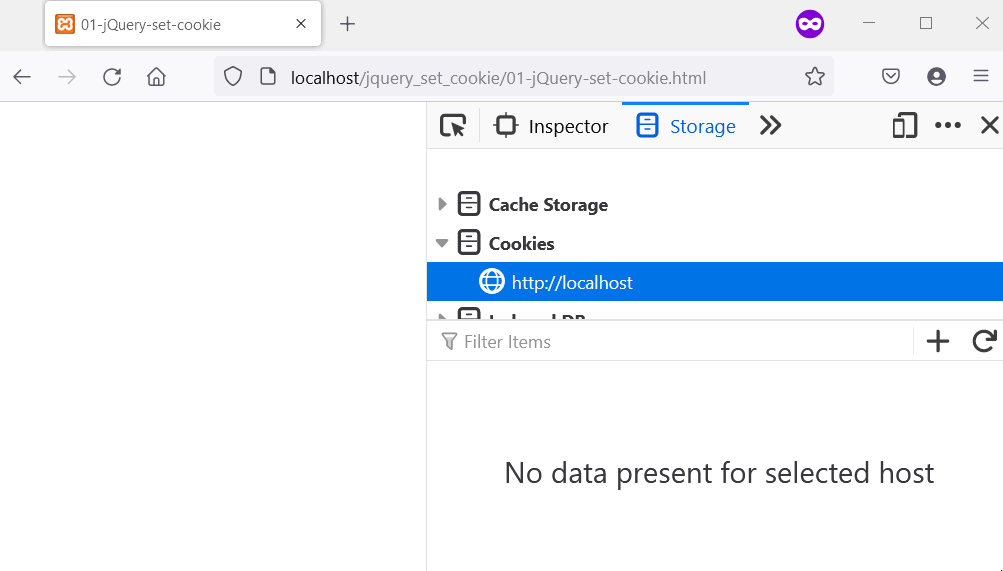
This article teaches two examples of how to set and delete a cookie using jQuery. In the first example, you’ll use a plugin called jquery-cookie
that you can get from GitHub.
The second example will use a custom function to create and delete the cookie.
Create and Delete a Cookie Using jquery-cookie
in jQuery
The jquery-cookie
plugin allows you to create and delete cookies on your web browser. The plugin has two functions that make this possible; they are $.cookie()
and $.removeCookie()
.
The $.cookie()
function allows you to set the cookie name and its parameters. While the $.removeCookie()
function will delete the cookie.
You can get jquery-cookie
from their GitHub repo; after that, save it in your working directory. From there, ensure you have linked jQuery as well.
The following code contains details on how to use jquery-cookie
to set and delete a cookie.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-set-cookie</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<script type="text/javascript" src="js/jquery-cookie.js"></script>
<script>
// Define a cookie called DelftStack_Cookie
// and set its value to 4500.
$.cookie("DelfStack_Cookie", 4500);
let cookie_value = $.cookie("DelfStack_Cookie");
/* Remove the cookie
$.removeCookie("DelfStack_Cookie");*/
</script>
</body>
</html>
Output (observe the browser console):
Create and Delete a Cookie Using a Custom Function in jQuery
A custom function that calculates time and uses the document.cookie()
method can set and delete a cookie. Afterward, you’ll use the time calculation to determine the cookie’s expiry date.
For this, you’ll need the Date
object methods; these are setTime()
, getTime()
, and toGMTString()
. Moreover, you’ll escape characters in the cookie name using encodeURIComponent
.
In the following code, you’ll find the custom function you can use to set a cookie. But, we’ve left the code that can delete a cookie as a comment.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-set-cookie</title>
</head>
<body>
<script>
function create_cookie(cookie_name, cookie_value, cookie_life_time) {
let cookie_expires;
if (cookie_life_time) {
let current_date = new Date();
let current_time = current_date.getTime();
current_date.setTime(current_time + (cookie_life_time * 24 * 60 * 60 * 1000));
cookie_expires = "; expires=" + current_date.toGMTString();
} else {
cookie_expires = "";
}
document.cookie = encodeURIComponent(cookie_name) + "=" + encodeURIComponent(cookie_value) + cookie_expires + "; path=/";
}
create_cookie('Habdul Hazeez', '14092022', 1);
/* Erase the stored cookie
function erase_cookie(cookie_name) {
create_cookie(cookie_name, "", -1);
}*/
</script>
</body>
</html>
Output (observe the browser console):
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn