How to Reset HTML Form Using jQuery
-
Use the
trigger()
Method to Reset HTML Form Using jQuery -
Create a jQuery Form Object and Use JavaScript’s
reset()
Method to Reset HTML Form
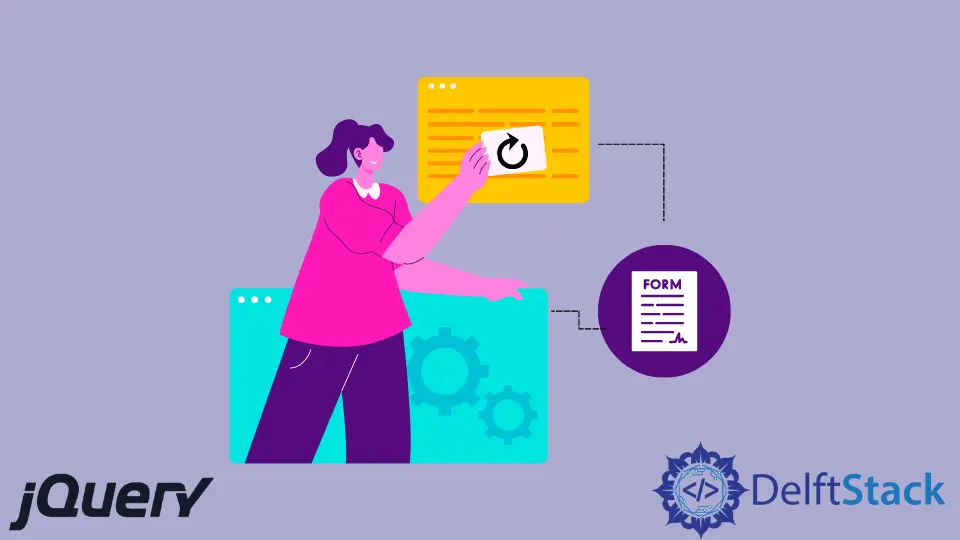
jQuery does not have a method like the JavaScript reset()
method, but we can use jQuery’s trigger()
method to reset the HTML form.
This tutorial demonstrates how to use the trigger()
method to reset the HTML form in jQuery.
Use the trigger()
Method to Reset HTML Form Using jQuery
The trigger()
method can trigger a specified event handler on selected elements. We can use this method with the reset
parameter to reset the HTML form.
The syntax for the trigger()
method to reset the form is:
$("Form").trigger("reset");
Where the Form
is a selector for the form, it can be an id, class, or the form
keyword.
Let’s try an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>ResetForm Using JQuery</title>
</head>
<body>
<form action="" method="post" id="DemoForm">
<label>Name:</label>
<input type="text" name="name">
<br><br>
<label>ID:</label>
<input type="text" name="id">
<br><br>
<label>Address:</label>
<input type="text" name="address">
<br><br
<label>Salary:</label>
<input type="number" name="salary">
<input type="submit" value="Submit">
</form>
<br>
<button type="button" id='ResetButton'>Reset Form</button>
<script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>
<script>
$(document).ready(function(){
$("#ResetButton").click(function(){
$("#DemoForm").trigger("reset");
});
});
</script>
</body>
</html>
The code above will reset the form on the button click. See output:
Create a jQuery Form Object and Use JavaScript’s reset()
Method to Reset HTML Form
Another method to reset the form in jQuery is that we can make a jQuery object of the form and reset it with the JavaScript reset()
method. The syntax for this method is:
$("#DemoForm")[0].reset()
Where [0]
will convert the jQuery object to a JavaScript object and then apply the reset()
method.
See the following example for this method:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Reset Form Using JQuery</title>
</head>
<body>
<form action="" method="post" id="DemoForm">
<label>Name:</label>
<input type="text" name="name">
<br><br>
<label>ID:</label>
<input type="text" name="id">
<br><br>
<label>Address:</label>
<input type="text" name="address">
<br><br
<label>Salary:</label>
<input type="number" name="salary">
<input type="submit" value="Submit">
</form>
<br>
<button type="button" id='ResetButton'>Reset Form</button>
<script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>
<script>
$(document).ready(function() {
$("ResetButton").click(function() {
$("#DemoForm")[0].reset()
});
});
</script>
</body>
</html>
The code above will convert the jQuery object to JavaScript and reset the form using the reset()
method. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook