How to Get Form Data Using JQuery
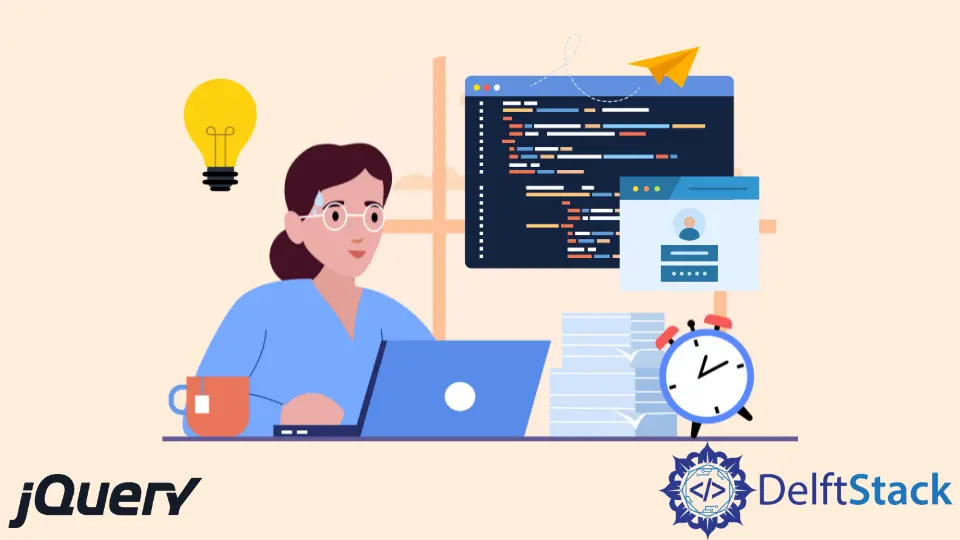
This article educates about how to get form data using JQuery. The serialize()
method is widely used to avail data from a form submission or any update in the input fields.
Another method, serializeArray()
, is parallelly used to represent form data in the form of an array. The format to store form data as an object is yet integrated with any defined method, but it is also possible.
Let’s learn how we can follow each of the approaches mentioned above.
Get Form Data Using JQuery
In jQuery, if we want to fetch the form or input field data, there are two ways to follow. One is the serialize()
method and the other is serializeArray()
method.
These techniques are helpful to get the form data if we have full knowledge of user preference, user categorization, and many more. These can also be stored in the database.
So, what format can be when we get the form data?
The serialize()
method returns a string, and the different input types are separated by &
. Finally, we have the serializeArray()
method that fetches the form data more scalable way.
It shows data as an array. And here, we will also examine if we can get those data as an object. So, let’s hop on to the code segment!
Use the serialize()
Method to Get Form Data
In this example, we will have two fields. One text
type input field and the other is a simple select-option
section. Whatever input we enter in those fields, we can get those data in a string format by using the serialize()
method. So let’s see what’s happening in the code base.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<title>form data</title>
<style>
div {
margin-top: 27px;
}
#serialize {
background-color: #eee;
border: 1px solid #111;
padding: 3px;
margin: 9px;
width: 300px;
}
</style>
</head>
<body>
<form action="">
Name: <input type="text" name="name">
<select name="hair" id="hair">
<option value="Hair">Hair</option>
<option value="black">black</option>
<option value="brown">brown</option>
<option value="blue">brown</option>
</select>
</form>
<div>Serialize:
<div id="serialize"></div>
</div>
<script>
$(function() {
var update = function() {
$('#serialize').text(
JSON.stringify($('form').serialize())
);
};
update();
$('form').change(update);
})
</script>
</body>
</html>
Output:
When we type the value in the Name
field, we see that the update is getting added. And also, in the select box, the hair color was chosen to be black
and modified in the string determined by the serialize()
method.
Use the serializeArray()
Method to Get Form Data
This specific example implies the same task done in the previous segment, but the difference will be that the output here will be in an array form. An array is more defined, and this array convention is not like the typical style; instead, it is an array of objects.
So, the 0
indexed array element will hold the key-pair
value of the first input field, and the 1
indexed array will store the select-option
choice. But, first, let’s check the code.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<title>form data</title>
<style>
div {
margin-top: 27px;
}
#serializearray{
background-color: #eee;
border: 1px solid #111;
padding: 3px;
margin: 9px;
width: 300px;
}
</style>
</head>
<body>
<form action="">
Name: <input type="text" name="username">
<select name="hair" id="hair">
<option value="Hair">Hair</option>
<option value="black">black</option>
<option value="brown">brown</option>
</select>
</form>
<div>Serialize Array:
<div id="serializearray"></div>
</div>
<script>
$(function() {
var update = function() {
$('#serializearray').text(
JSON.stringify($('form').serializeArray())
);
};
update();
$('form').change(update);
})
</script>
</body>
</html>
Output:
Here, the value is altered, and the hair’s color is updated. But you notice that the result is in an array form, and it took two objects as its entries.
Next, we wish to examine if we can directly store the form data as objects. So let’s go for it.
Get Form Data in an Object Form
For this example, we use the same code as before, but here we will have to use two div
elements to output two objects. We have added a $.each()
method that will loop through the array generated by the serializeArray()
method and parse them as objects.
You will notice we used two conditional statements to define the array indexes. Otherwise, it looped through the last element, resulting in only the last field. So first, let’s check the code snippet.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<title>form data</title>
<style>
div {
margin-top: 27px;
}
#serializeobj1, #serializeobj2{
background-color: #eee;
border: 1px solid #111;
padding: 3px;
margin: 9px;
width: 300px;
}
</style>
</head>
<body>
<form id="form">
Name: <input type="text" name="username">
<select name="hair" id="hair">
<option value="Hair">Hair</option>
<option value="black">black</option>
<option value="brown">brown</option>
<option value="green">brown</option>
</select>
</form>
<div>Serialize Object:
<div id="serializeobj1"></div>
<div id="serializeobj2"></div>
</div>
<script>
$(function() {
var obj = {}
var cnt=0;
var update = function() {
$.each(
$('#form').serializeArray(), function(i, val){
if(i==0){
$('#serializeobj1').text(
JSON.stringify(
obj[i]=val
)
)
}
if(i==1){
$('#serializeobj2').text(
JSON.stringify(
obj[i]=val
)
)
}
})
};
update();
$('form').change(update);
})
</script>
</body>
</html>
Output: