How to Remove a CSS Property Using jQuery
-
Remove a CSS Property Using jQuery
.css()
API -
Remove a CSS Property Using jQuery
.prop()
API -
Remove a CSS Property Using jQuery
.removeAttr()
API -
Remove a CSS Property Using jQuery
.removeClass()
API
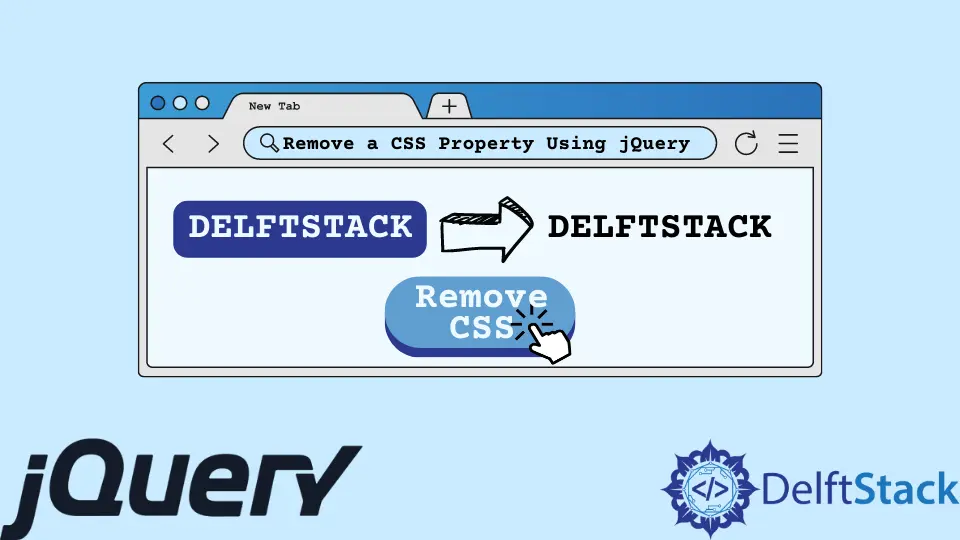
This article teaches four methods to remove a CSS property using jQuery. These methods will use four jQuery APIs which are .css()
, .removeAttr()
, .removeClass()
, and .prop()
.
Remove a CSS Property Using jQuery .css()
API
In jQuery, you’ll default use the .css()
API to set a CSS property and its value on an element. From this, jQuery will apply the property and the value as inline styles via the HTML style
attribute.
But you can also use it to remove a CSS property by setting the property’s value to an empty string. This means this method will not work if the CSS is from the <style>
tag or an external CSS style sheet.
We’ve applied a CSS property to an element using jQuery .css()
API in the following code. Afterward, you can press the button; this calls a function that will remove the CSS property.
The function’s core is to set the value of a CSS property to an empty string.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<p id="test">Random Text</p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add two CSS properties
$("#test").css({
'font-size': '2em',
'background-color': 'red'
});
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
$("#test").css({
'font-size': '',
'background-color': ''
});
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
Output:
Remove a CSS Property Using jQuery .prop()
API
You can use the jQuery .prop()
API to remove a CSS property if you’ve applied the CSS via the jQuery .css()
API. That’s because such an element will have the style
attribute you can access using the .prop()
API.
In the following, we’ve updated the previous code to use the .prop()
API to remove the CSS property.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<p id="test">Random Text 2.0</p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add two CSS properties
$("#test").css({
'font-size': '3em',
'background-color': '#1560bd'
});
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
let element_with_css_property = $("#test").prop('style');
element_with_css_property.removeProperty('font-size');
element_with_css_property.removeProperty('background-color');
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
Output:
Remove a CSS Property Using jQuery .removeAttr()
API
The .removeAttr()
API will remove a CSS property by removing the style
attribute from the element. This means the CSS should be inline, or you’ve applied it using the jQuery .css()
API.
The button will remove all the CSS properties by removing the style
attribute.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<p id="test">Random Text 3.0</p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add two CSS properties
$("#test").css({
'padding': '2em',
'border': '5px solid #00a4ef',
'font-size': '3em'
});
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
$("#test").removeAttr('style');
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
Output:
Remove a CSS Property Using jQuery .removeClass()
API
The .removeClass()
API will remove a CSS property added via the <style>
tag or an external style sheet. If you pass a class name, it will remove it; otherwise, it will remove all the class names on the element.
The following example shows .removeClass()
in action.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>04-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
.colorful_big_text {
color: #1560bd;
font-size: 3em;
}
</style>
</head>
<body>
<main>
<p id="test"><i>Random Text 4.0</i></p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add a CSS class that contains CSS properties
$("#test").addClass('colorful_big_text');
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
$("#test").removeClass();
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn