jQuery를 사용하여 CSS 속성 제거
-
jQuery
.css()
API를 사용하여 CSS 속성 제거 -
jQuery
.prop()
API를 사용하여 CSS 속성 제거 -
jQuery
.removeAttr()
API를 사용하여 CSS 속성 제거 -
jQuery
.removeClass()
API를 사용하여 CSS 속성 제거
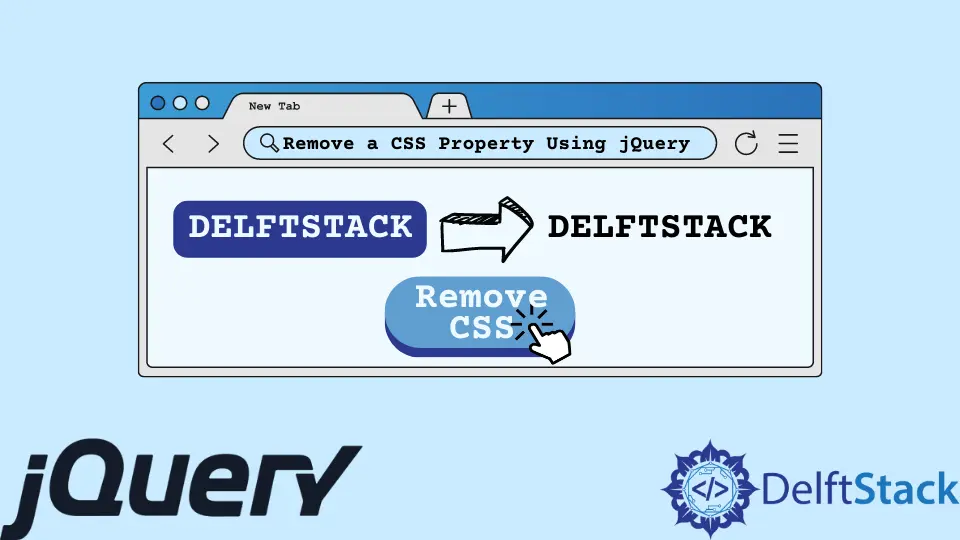
이 기사에서는 jQuery를 사용하여 CSS 속성을 제거하는 네 가지 방법을 설명합니다. 이러한 메서드는 .css()
, .removeAttr()
, .removeClass()
및 .prop()
인 네 가지 jQuery API를 사용합니다.
jQuery .css()
API를 사용하여 CSS 속성 제거
jQuery에서는 기본적으로 .css()
API를 사용하여 요소에 CSS 속성과 해당 값을 설정합니다. 여기에서 jQuery는 HTML style
속성을 통해 속성과 값을 인라인 스타일로 적용합니다.
그러나 속성 값을 빈 문자열로 설정하여 CSS 속성을 제거하는 데 사용할 수도 있습니다. 즉, CSS가 <style>
태그 또는 외부 CSS 스타일 시트에 있는 경우 이 방법이 작동하지 않습니다.
다음 코드에서 jQuery .css()
API를 사용하여 요소에 CSS 속성을 적용했습니다. 그런 다음 버튼을 누를 수 있습니다. CSS 속성을 제거하는 함수를 호출합니다.
함수의 핵심은 CSS 속성 값을 빈 문자열로 설정하는 것입니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<p id="test">Random Text</p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add two CSS properties
$("#test").css({
'font-size': '2em',
'background-color': 'red'
});
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
$("#test").css({
'font-size': '',
'background-color': ''
});
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
출력:
jQuery .prop()
API를 사용하여 CSS 속성 제거
jQuery .css()
API를 통해 CSS를 적용한 경우 jQuery .prop()
API를 사용하여 CSS 속성을 제거할 수 있습니다. 이러한 요소에는 .prop()
API를 사용하여 액세스할 수 있는 style
속성이 있기 때문입니다.
다음에서는 .prop()
API를 사용하여 CSS 속성을 제거하도록 이전 코드를 업데이트했습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<p id="test">Random Text 2.0</p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add two CSS properties
$("#test").css({
'font-size': '3em',
'background-color': '#1560bd'
});
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
let element_with_css_property = $("#test").prop('style');
element_with_css_property.removeProperty('font-size');
element_with_css_property.removeProperty('background-color');
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
출력:
jQuery .removeAttr()
API를 사용하여 CSS 속성 제거
.removeAttr()
API는 요소에서 style
속성을 제거하여 CSS 속성을 제거합니다. 이는 CSS가 인라인이어야 하거나 jQuery .css()
API를 사용하여 CSS를 적용했음을 의미합니다.
버튼은 스타일
속성을 제거하여 모든 CSS 속성을 제거합니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<p id="test">Random Text 3.0</p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add two CSS properties
$("#test").css({
'padding': '2em',
'border': '5px solid #00a4ef',
'font-size': '3em'
});
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
$("#test").removeAttr('style');
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
출력:
jQuery .removeClass()
API를 사용하여 CSS 속성 제거
.removeClass()
API는 <style>
태그 또는 외부 스타일 시트를 통해 추가된 CSS 속성을 제거합니다. 클래스 이름을 전달하면 제거됩니다. 그렇지 않으면 요소의 모든 클래스 이름이 제거됩니다.
다음 예제는 작동 중인 .removeClass()
를 보여줍니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>04-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
.colorful_big_text {
color: #1560bd;
font-size: 3em;
}
</style>
</head>
<body>
<main>
<p id="test"><i>Random Text 4.0</i></p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add a CSS class that contains CSS properties
$("#test").addClass('colorful_big_text');
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
$("#test").removeClass();
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
출력:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn