How to Create Multiselect Dropdown in jQuery
-
Create Multiselect Dropdown Using
Select2
-
Create Multiselect Dropdown Using
Selectize.js
-
Create Multiselect Dropdown Using jQuery
Multiselect.js
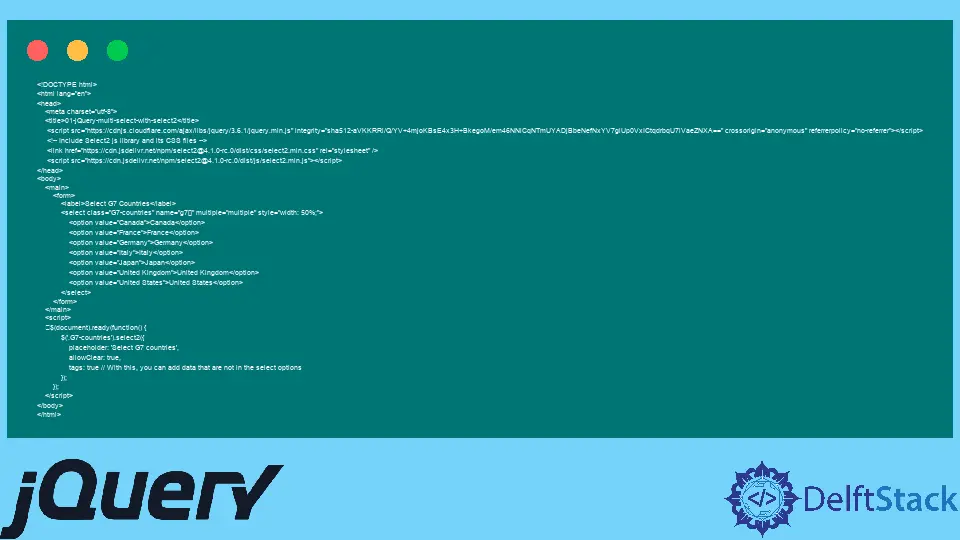
This article teaches you how to create a multiselect dropdown in jQuery. We’ll do this using three libraries; Select2
, Selectize.js
, and jquery multiselect.js
.
These libraries have different implementations, but they’ll help you create a multiselect dropdown.
Create Multiselect Dropdown Using Select2
Select2
is a jQuery library that allows you to turn a normal HTML <select>
menu into a multiselect menu. To get started, ensure your <select>
menu have some data in the <option>
fields.
From there, include Select2
JavaScript and CSS files from cdnjs
. It’s best to place these files along with jQuery in the <head>
element.
Then in an external JavaScript file or a <script>
tag, you can call Select2
on the <select>
menu. Afterward, you can customize the menu to suit your needs.
For example, you can change the placeholder text and include a clear button
. What’s more, Select2
allows your user to include data that are not in the <select>
menu.
In the code example below, we’ve used Select2
to customize the <select>
menu. Also, you’ll find the customizations that we talked about in the last paragraph.
If you need more customizations, you can check their official website.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-multi-select-with-select2</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<!-- Include Select2 js library and its CSS files -->
<link href="https://cdn.jsdelivr.net/npm/select2@4.1.0-rc.0/dist/css/select2.min.css" rel="stylesheet" />
<script src="https://cdn.jsdelivr.net/npm/select2@4.1.0-rc.0/dist/js/select2.min.js"></script>
</head>
<body>
<main>
<form>
<label>Select G7 Countries</label>
<select class="G7-countries" name="g7[]" multiple="multiple" style="width: 50%;">
<option value="Canada">Canada</option>
<option value="France">France</option>
<option value="Germany">Germany</option>
<option value="Italy">Italy</option>
<option value="Japan">Japan</option>
<option value="United Kingdom">United Kingdom</option>
<option value="United States">United States</option>
</select>
</form>
</main>
<script>
$(document).ready(function() {
$('.G7-countries').select2({
placeholder: 'Select G7 countries',
allowClear: true,
tags: true // With this, you can add data that are not in the select options
});
});
</script>
</body>
</html>
Output:
Create Multiselect Dropdown Using Selectize.js
Selectize.js
is another library that’ll turn your <select>
menu into a dropdown menu. Like Select2
, it has more customizations.
This includes limiting the number of selections and adding a remove button. Also, it has Restore on backup
, so you can hit Backspace without deleting the selected option.
The first example below shows how to change a <select>
menu into a dropdown menu. Also, we added an option to limit the number of selections; but we left it as a comment.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-multi-select-with-selectize-js</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<!-- Include Selectize JS files and a CSS library here,
we are using Bootstrap5 -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/selectize.js/0.13.6/js/standalone/selectize.min.js" integrity="sha512-pgmLgtHvorzxpKra2mmibwH/RDAVMlOuqU98ZjnyZrOZxgAR8hwL8A02hQFWEK25V40/9yPYb/Zc+kyWMplgaA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/selectize.js/0.13.6/css/selectize.bootstrap5.min.css" integrity="sha512-w4sRMMxzHUVAyYk5ozDG+OAyOJqWAA+9sySOBWxiltj63A8co6YMESLeucKwQ5Sv7G4wycDPOmlHxkOhPW7LRg==" crossorigin="anonymous" referrerpolicy="no-referrer" />
</head>
<body>
<main>
<form>
<label>Select G7 Countries</label>
<select class="G7-countries" name="g7[]" multiple="multiple" style="width: 50%;">
<option value="Canada">Canada</option>
<option value="France">France</option>
<option value="Germany">Germany</option>
<option value="Italy">Italy</option>
<option value="Japan">Japan</option>
<option value="United Kingdom">United Kingdom</option>
<option value="United States">United States</option>
</select>
</form>
</main>
<script>
$(".G7-countries").selectize({
// maxItems: 3,
});
</script>
</body>
</html>
Output:
The following is the output when you enable the maxItems
property in the code above. Here, we’ve set it to three items; as a result, you can’t select more than three options.
Afterward, the select menu will not show again.
We’ve changed the HTML in this second example into a single <input>
field. With this, you can create a tagging system; where you type, and it’s included in the selected options.
Moreover, we’ve enabled the remove_button
and restore_on_backspace
plugins.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-multi-select-with-selectize-js</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<!-- Include Selectize JS files and a CSS library here,
we are using Bootstrap5 -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/selectize.js/0.13.6/js/standalone/selectize.min.js" integrity="sha512-pgmLgtHvorzxpKra2mmibwH/RDAVMlOuqU98ZjnyZrOZxgAR8hwL8A02hQFWEK25V40/9yPYb/Zc+kyWMplgaA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/selectize.js/0.13.6/css/selectize.bootstrap5.min.css" integrity="sha512-w4sRMMxzHUVAyYk5ozDG+OAyOJqWAA+9sySOBWxiltj63A8co6YMESLeucKwQ5Sv7G4wycDPOmlHxkOhPW7LRg==" crossorigin="anonymous" referrerpolicy="no-referrer" />
</head>
<body>
<main>
<form>
<input id="input-tags" type="text" name="" style="width: 30%;">
</form>
</main>
<script>
$("#input-for-tags").selectize({
plugins: ["remove_button", "restore_on_backspace"], // plugins
delimiter: ",",
persist: false,
create: function (input) {
return {
value: input,
text: input,
};
},
});
</script>
</body>
</html>
Output:
Create Multiselect Dropdown Using jQuery Multiselect.js
Multiselect.js
works like Select2
and Selectize.js
, but the <select>
menu has a different look. Also, after customizing the <select>
menu, you can enable an option to keep the selection order.
Without it, Multiselect.js
will arrange your selection alphabetically. To get started, download Multiselect.js
from their website, and do the following.
- Extract the downloaded
.zip
file. - Locate the
js
folder and copy thejquery.multi-select.js
into your working directory. - Locate the
css
folder and copy themulti-select.css
file into your working directory. - Locate the
img
folder and copyswitch.png
. - Create an
img
folder in your working directory and pasteswitch.png
. - Link
jquery.multi-select.js
andmulti-select.css
files to your HTML file.
The next code example shows Multiselect.js
in action.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-multi-select-with-selectize-js</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<script src="multi-select-js-and-css/jquery.multi-select.js"></script>
<link rel="stylesheet" type="text/css" href="multi-select-js-and-css/multi-select.css">
</head>
<body>
<main>
<form>
<label>Select G7 Countries</label>
<select id="G7-countries" multiple="multiple" style="width: 30%;">
<option value="Canada">Canada</option>
<option value="France">France</option>
<option value="Germany">Germany</option>
<option value="Italy">Italy</option>
<option value="Japan">Japan</option>
<option value="United Kingdom">United Kingdom</option>
<option value="United States">United States</option>
</select>
</form>
</main>
<script>
$('#G7-countries').multiSelect({
// keepOrder: true
});
</script>
</body>
</html>
Output:
The output with keepOrder
is set to true
.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn