How to Select the First Option of the Dropdown Using jQuery
-
Select the First Option of the
<Select>
Tag Using jQuery -
Use the
SelectedIndex
Property -
Use the
val()
Method -
Use the
prop()
Method -
Use the
attr()
Method
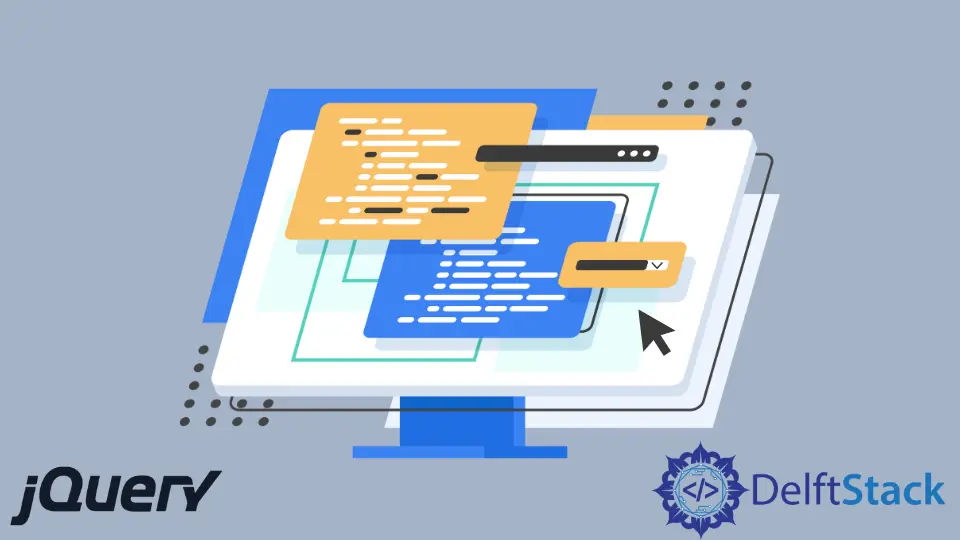
This tutorial will use jQuery to select the first option of the select
tag. We’ll look into various jQuery implementation strategies to help us implement the functionality we want.
Select the First Option of the <Select>
Tag Using jQuery
Drop-down menus and lists are very familiar and handy on our web pages. It might help display the filters on our website, or we can use it to create a drop-down menu on the navigation bar that displays many pages from the same categories all in one place.
Regardless of how we utilize it on our websites, there are times when we wish to pre-select a particular option, and this is the focus of today’s article. We’re going to talk about using jQuery to implement this feature.
jQuery is a lightweight JavaScript library created to make it simple to utilize JavaScript on your web pages. We will discuss the following four methods for selecting the first option of the <select>
tag using jQuery.
SelectedIndex
Property.val()
Method.prop()
Method.attr
Method
Use the SelectedIndex
Property
The selected option’s index is set or returned in a drop-down list by the HTML DOM’s selectedIndex
property. The index begins at zero.
To deselect all options, enter a value of -1
. If the user selects no options, the selectedIndex
property returns -1
.
Syntax:
-
It will return the
selectedIndex
property.selectObject.selectedIndex
-
It will set the
selectedIndex
property.selectObject.selectedIndex = number
Check the example below to understand the working of this property.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$('#drop_down')[0].selectedIndex = 0;
});
</script>
</head>
<body>
<select id="drop_down">
<option>Value1</option>
<option>Value2</option>
<option>Value3</option>
</select>
</body>
</html>
Output:
Let’s see what we did in the code. We must choose the <select>
tag to select the first option.
So at first, we select the element using the id
. You might find it confusing that we put [0]
after the jQuery selector.
To help you grasp this, let us provide an in-depth explanation.
When we use the jQuery selector to select a particular element, it gives us an array of the selected items. Because we’re using ID, there will only be one element in the array, which must be at index 0
.
Because of this, we write [0]
to choose the first index of the returned array.
When the required element is selected, we call the selectedIndex
property on it and assign the value 0
to it, which means it will choose the first option.
We set 0
and not 1
because we deal with indexes, and indexes start from 0
, so 0
means the first value, 1
means the second value, and so on.
Use the val()
Method
The val()
method implements actions on the values of HTML elements. The value of a certain element can be set or retrieved using this method.
The val()
method can only be applied to HTML elements with a value
property.
Syntax:
-
It will return the value of the selected element.
$(selector).val()
-
It will set the value of the selected element to the value we specified.
$(selector).val(value)
-
This will use a function to set the value of the selected element. If specified, the
currentvalue
returns the element’s value, and theindex
parameter returns the element’s index.$(selector).val(function(index, currentvalue))
We will use the val()
method to select the first item from a drop-down list. Look at two strategies below that can help us attain that functionality.
Scenario 1
We can use the above-discussed syntax to select the first or any option from the drop-down list. We know that $(selector).val(value)
is used to set the value of the selected element, and we also understand that this method works on the value
attribute.
So first, set the value of each option
tag in the select
element, and then we will select our required option using the val()
method.
Let’s choose the second option now. Check the code below.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$('#drop_down').val("2");
});
</script>
</head>
<body>
<select id="drop_down">
<option value="1">Value1</option>
<option value="2">Value2</option>
<option value="3">Value3</option>
</select>
</body>
</html>
Output:
This is quite simple. We called the val()
function with the argument value 2
after setting the value to the option
tags in the value
field.
Scenario 2
Another method to select the first option is using the .first()
method of jQuery, which returns the first element of the selected tag. Observe the code below to understand it more clearly.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#drop_down").val($("#drop_down option").first().val());
});
</script>
</head>
<body>
<select id="drop_down">
<option value="1">Value1</option>
<option value="2"selected>Value2</option>
<option value="3">Value3</option>
</select>
</body>
</html>
Output:
To help you grasp this approach, we choose the second option as the default selected option by writing selected
in the tag, and if jQuery is not used, the second option will be displayed as the selected option.
However, we added jQuery to our code and used the .first()
method on the option
tag of the chosen element. It shows the first option as the selected one, as seen in the output.
In the older versions of jQuery we had this .first()
method as a pseudo selector and to use it we write $("#drop_down").val($("#drop_down option:first").val())
, but this is deprecated in the latest releases and is not recommended to use this way.
Use the prop()
Method
Properties and values of the selected items are set or returned by the prop()
function. As we can access the properties by using this function, we can easily choose the first option of the drop-down list.
Let’s discuss two methods for implementing our required functionality using this method.
Scenario 1
We know that the prop()
method lets us access the element’s properties. The first approach we discussed for selecting the required option in this article is using the selectedIndex
property.
We can also access that property using the prop()
method. Here’s how.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#drop_down").prop("selectedIndex", 1)
});
</script>
</head>
<body>
<select id="drop_down">
<option>Value1</option>
<option>Value2</option>
<option>Value3</option>
</select>
</body>
</html>
Output:
The code is pretty simple and easy to understand. We told the prop()
method to select index 1
, which indicates that option two will be chosen.
Scenario 2
Accessing the selected
property of the option
tag is another method for choosing the required option from the drop-down list using the prop()
method. We frequently want a specific option to be selected by default, so we add the selected
attribute to the tag, indicating that the option is selected.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#drop_down option").first().prop("selected", "true");
});
</script>
</head>
<body>
<select id="drop_down">
<option>Value1</option>
<option>Value2</option>
<option>Value3</option>
</select>
</body>
</html>
Use the attr()
Method
The attr()
method aims to set or return the values and attributes of the elements that have been selected. We can easily choose our required option from the list using this method.
Look at the two strategies listed below.
Scenario 1
Let’s talk about a particular situation when we want to choose the first non-disabled choice and want to ignore the disabled options, even if they are the first ones. To learn how to implement the required functionality, examine the code below.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#drop_down option:not([disabled])").first().attr('selected','selected');
});
</script>
</head>
<body>
<select id="drop_down" style="width:150px">
<option selected disabled>Choose the Option</option>
<option>Value1</option>
<option>Value2</option>
<option>Value3</option>
</select>
</body>
</html>
First, let’s see what the output will be if we don’t use this method.
The selected option is the first one. Although it is disabled, we can still set its selected
attribute to show this option as selected.
Now let’s see which option will be selected with our above-written code.
Amazing, right? The first non-disabled element that is available will be chosen, and the value of its selected
attribute will be set.
Scenario 2
Another intriguing scenario is when we wish to remove any selected option
tag before selecting our desired option. This can quickly be done using some of the jQuery methods.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$('#drop_down').removeAttr('selected').find('option:first').attr('selected', 'selected');
});
</script>
</head>
<body>
<select id="drop_down">
<option>Value1</option>
<option>Value2</option>
<option>Value3</option>
</select>
</body>
</html>
First, we called the removeAttr()
method, which will remove any selected option. Then using the find()
method, we searched for the first option and then set the value of its selected
attribute.
One important thing here is that we used first()
as the pseudo selector in our code, and we already discussed that this is deprecated now. So if your browser doesn’t support it you can write it like $('#drop_down').removeAttr('selected').find('option').first().attr('selected', 'selected')
.