jQuery Global Variable
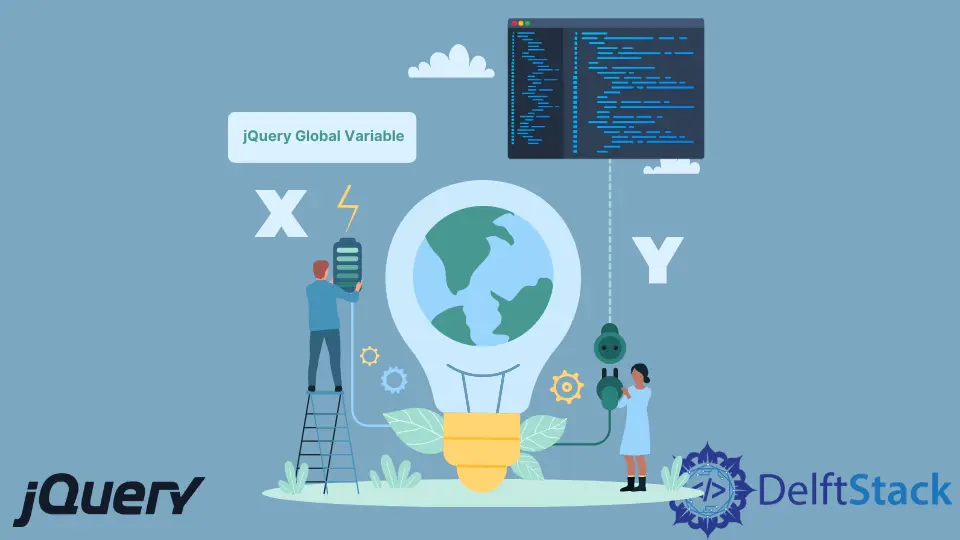
This tutorial demonstrates how to create a global variable in jQuery.
jQuery Global Variable
A global variable is declared outside a function and can be used in any function in jQuery. The variable declared outside the function will get the scope global
and can be accessed anywhere.
There are two methods to declare a global variable in jQuery:
- Declare a variable outside a function with a
var
keyword without initializing the value. - Declare a variable without the
var
keyword and initialize a value for it. It will give the global variable scope.
The syntax to declare a global variable is:
var globalVariable = value;
// The globalVariable can be used here
function Fun() {
// The globalVariable can also be used here
}
Here, the globalVariable
is the variable name, and the value
is assigned when we want to initialize the variable.
Let’s try an example for the first method:
<!DOCTYPE html>
<html lang= "en" >
<head>
<meta charset= "utf-8" >
<script type = "text/javascript" src="https://code.jquery.com/jquery-3.6.0.js" ></script>
<title> jQuery global variable </title>
<script>
// global variable
var globalVariable;
$(function(){
globalVariable = "Global Variable";
// use globalVariable
alert(globalVariable);
// local variable
var localVariable = "Local Variable";
$("#Demobutton").click(function(){
// Use both local and globalVariable
alert(globalVariable+" is added to "+localVariable);
return false;
});
});
</script>
</head>
<body>
<button id="Demobutton"> Click Here</button>
</body>
</html>
We first declared the globalVariable
with the var
keyword and then assigned it a value
in the function. The variable and value are both global in this case.
See the output:
Now let’s try an example for method two:
<!DOCTYPE html>
<html lang= "en" >
<head>
<meta charset= "utf-8" >
<script type = "text/javascript" src="https://code.jquery.com/jquery-3.6.0.js" ></script>
<title> jQuery global variable </title>
<script>
// global variable
globalVariable = "Global Variable";
$(function(){
// use globalVariable
alert(globalVariable);
// local variable
var localVariable = "Local Variable";
$("#Demobutton").click(function(){
// Use both local and globalVariable
alert(globalVariable+" is added to "+localVariable);
return false;
});
});
</script>
</head>
<body>
<button id="Demobutton"> Click Here</button>
</body>
</html>
Similarly, we initialize a global variable without the var
keyword with a value outside a function. Both variables and their value be global.
See that the output is similar:
Let’s try another example to show the usage of a global variable:
<!DOCTYPE html>
<html>
<head>
<meta charset= "utf-8" >
<script type = "text/javascript" src="https://code.jquery.com/jquery-3.6.0.js" ></script>
<title> jQuery global variable </title>
<style>
#Main {
border: 5px solid green;
background-color : lightgreen;
height: 10%;
width: 20%;
}
</style>
<script>
// Global Variable means the default value
var DefaultNumber1 = 10;
var DefaultNumber2 = 40;
function sum() {
// check if number 1 and number 2 are entered or not
if($("#Number1").val() & $("#Number2").val()) {
// change the global variable value based on the input
DefaultNumber1 = Number(document.getElementById("Number1").value);
DefaultNumber2 = Number(document.getElementById("Number2").value);
}
var MultiplyResult = DefaultNumber1 * DefaultNumber2;
var PrintMessage = "Multiplication of given numbers is : " + MultiplyResult;
// display the result
document.getElementById("PrintResult").innerHTML = PrintMessage;
}
</script>
</head>
<body >
<div id = "Main">
<h1> Multiplication of two numbers </h1>
<p> Enter two numbers to perform multiplication, if any number is not entered, the multiplication will be performed based on the default values(global variable values 10 and 40).
<br>
<b>Enter number 1: </b>
<input type="number" id="Number1">
<br><br>
<b>Enter number 2: </b>
<input type="number" id="Number2">
<br><br>
<button onclick="sum()"> Multiply </button>
<p id="PrintResult" "></p>
</div>
</body>
</html>
As we can see, we initialized global variables as default values, so if the system cannot find values from the input, it uses the global values. See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook