jQuery 전역 변수
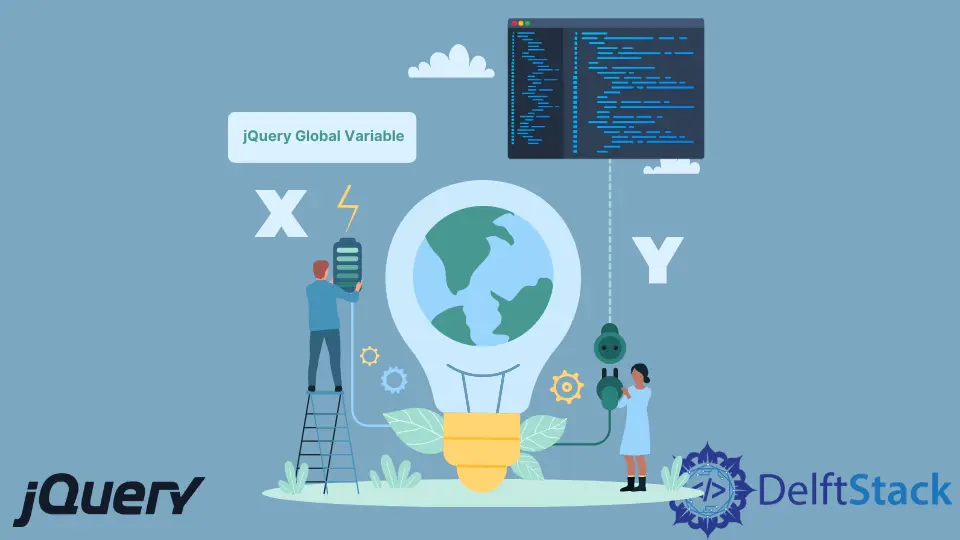
이 자습서는 jQuery에서 전역 변수를 만드는 방법을 보여줍니다.
jQuery 전역 변수
전역 변수는 함수 외부에서 선언되며 jQuery의 모든 함수에서 사용할 수 있습니다. 함수 외부에서 선언된 변수는 전역
범위를 가지며 어디에서나 액세스할 수 있습니다.
jQuery에서 전역 변수를 선언하는 두 가지 방법이 있습니다.
- 값을 초기화하지 않고
var
키워드를 사용하여 함수 외부에서 변수를 선언합니다. var
키워드 없이 변수를 선언하고 해당 값을 초기화합니다. 전역 변수 범위를 제공합니다.
전역 변수를 선언하는 구문은 다음과 같습니다.
var globalVariable = value;
// The globalVariable can be used here
function Fun() {
// The globalVariable can also be used here
}
여기서 globalVariable
은 변수 이름이고 value
는 변수를 초기화할 때 할당됩니다.
첫 번째 방법의 예를 살펴보겠습니다.
<!DOCTYPE html>
<html lang= "en" >
<head>
<meta charset= "utf-8" >
<script type = "text/javascript" src="https://code.jquery.com/jquery-3.6.0.js" ></script>
<title> jQuery global variable </title>
<script>
// global variable
var globalVariable;
$(function(){
globalVariable = "Global Variable";
// use globalVariable
alert(globalVariable);
// local variable
var localVariable = "Local Variable";
$("#Demobutton").click(function(){
// Use both local and globalVariable
alert(globalVariable+" is added to "+localVariable);
return false;
});
});
</script>
</head>
<body>
<button id="Demobutton"> Click Here</button>
</body>
</html>
먼저 var
키워드로 globalVariable
을 선언한 다음 함수에서 value
를 할당했습니다. 이 경우 변수와 값은 모두 전역적입니다.
출력을 참조하십시오.
이제 두 번째 방법에 대한 예를 살펴보겠습니다.
<!DOCTYPE html>
<html lang= "en" >
<head>
<meta charset= "utf-8" >
<script type = "text/javascript" src="https://code.jquery.com/jquery-3.6.0.js" ></script>
<title> jQuery global variable </title>
<script>
// global variable
globalVariable = "Global Variable";
$(function(){
// use globalVariable
alert(globalVariable);
// local variable
var localVariable = "Local Variable";
$("#Demobutton").click(function(){
// Use both local and globalVariable
alert(globalVariable+" is added to "+localVariable);
return false;
});
});
</script>
</head>
<body>
<button id="Demobutton"> Click Here</button>
</body>
</html>
마찬가지로 var
키워드 없이 전역 변수를 함수 외부의 값으로 초기화합니다. 변수와 해당 값은 모두 전역적입니다.
출력이 유사한지 확인합니다.
전역 변수의 사용법을 보여주는 또 다른 예를 살펴보겠습니다.
<!DOCTYPE html>
<html>
<head>
<meta charset= "utf-8" >
<script type = "text/javascript" src="https://code.jquery.com/jquery-3.6.0.js" ></script>
<title> jQuery global variable </title>
<style>
#Main {
border: 5px solid green;
background-color : lightgreen;
height: 10%;
width: 20%;
}
</style>
<script>
// Global Variable means the default value
var DefaultNumber1 = 10;
var DefaultNumber2 = 40;
function sum() {
// check if number 1 and number 2 are entered or not
if($("#Number1").val() & $("#Number2").val()) {
// change the global variable value based on the input
DefaultNumber1 = Number(document.getElementById("Number1").value);
DefaultNumber2 = Number(document.getElementById("Number2").value);
}
var MultiplyResult = DefaultNumber1 * DefaultNumber2;
var PrintMessage = "Multiplication of given numbers is : " + MultiplyResult;
// display the result
document.getElementById("PrintResult").innerHTML = PrintMessage;
}
</script>
</head>
<body >
<div id = "Main">
<h1> Multiplication of two numbers </h1>
<p> Enter two numbers to perform multiplication, if any number is not entered, the multiplication will be performed based on the default values(global variable values 10 and 40).
<br>
<b>Enter number 1: </b>
<input type="number" id="Number1">
<br><br>
<b>Enter number 2: </b>
<input type="number" id="Number2">
<br><br>
<button onclick="sum()"> Multiply </button>
<p id="PrintResult" "></p>
</div>
</body>
</html>
보시다시피 전역 변수를 기본값으로 초기화했으므로 시스템이 입력에서 값을 찾을 수 없으면 전역 값을 사용합니다. 출력을 참조하십시오.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook