How to Disable Button in jQuery
-
Disable Button in jQuery by Passing the
event
Object to theonclick
Event Handler -
Disable Button in jQuery With the
this
Reference in theonclick
Event Handler -
Disable Button in jQuery Using the
bind()
Method to Assign the Correct Value tothis
-
Disable Button in jQuery Using the
call()
Method to Assign the Correct Value tothis
- Disable Button in jQuery With Element ID Taking Advantage of JavaScript’s Flexible Dynamic Typing
-
Disable Button in jQuery With the
disabled
DOM Property Using the jQuery.prop()
Method
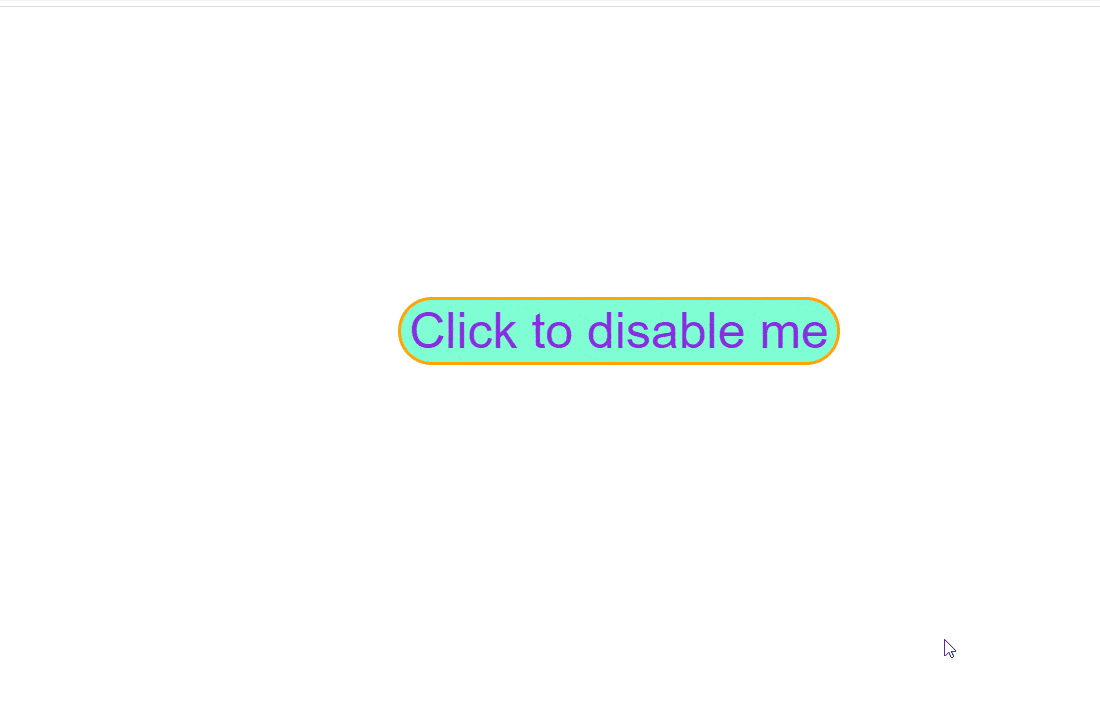
We will use the onclick
event handler with different arguments like the event
object, the this
reference, and the element id
to disable the button in jQuery in this tutorial. You will also find tips about dynamic typing in function arguments, event.target
versus event.currentTarget
, and the jQuery .prop()
versus .attr()
methods here.
Disable Button in jQuery by Passing the event
Object to the onclick
Event Handler
The onclick
event handler fires whenever we click on the target element. By default, the callback to onclick
takes only one argument, the event
object.
Let us use this default argument as our first method to disable the button in jQuery.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Default event argument</title>
<script type="text/javascript" src="jQuery.js"></script>
<script type="text/javascript">
function disable(e){
$(e.currentTarget).attr("disabled","disabled");
//updating the text to disabled
$(e.currentTarget).html("I am disabled");
}
</script>
<link rel="stylesheet" href="disable_button_jQuery.css">
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable(event)">Click to disable me</button>
</div>
</body>
</html>
We have a button
element where we attach an onclick
event handler. The callback to it takes in the default event
object argument.
We disable the button with the key code line:
$(e.currentTarget).attr("disabled","disabled")
The currentTarget
property of the event
object identifies the element to which the event handler is attached, the button
element in our case.
Finally, we set the disabled HTML attribute of this button
element to disabled
with the jQuery .attr()
method.
We also update the button
text to show its disabled state in the next line of code with the jQuery .html()
method.
Here is the working demo of this solution.
Pro Tip:
The event target
property is similar to the currentTarget
property, but it refers to the element on which the event fires. This could be different when the DOM element propagates in cases of event bubbling.
So, it is better to use the currentTarget
property in this case.
Disable Button in jQuery With the this
Reference in the onclick
Event Handler
the Problem With the Default this
Reference in the onclick
Event Handler
The this
keyword inside the onclick
event handler refers to the element on which it fires. But, we know the JavaScript this
keyword takes its context dynamically at run time.
So, by default, it does not refer to the triggering element in this case.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Default this Keyword</title>
<script type="text/javascript" src="jQuery.js"></script>
<link rel="stylesheet" href="disable_button_jQuery.css"/>
<script type="text/javascript">
function disable(){
console.log("The value of this by default is : " + this);
$(this).attr("disabled","disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable()">Click to disable me</button>
</div>
</body>
</html>
We use the this
reference inside the onclick
callback disable
function. We also log the value of the this
object for better understanding.
Let us look at the output of this code below:
Two things happened here:
- First, our code did not disable the button as we wanted.
- Second, when we look at the value of
this
in the console, it refers to thewindow
object. This is expected behavior.
As we execute the disable
callback outside any function in the inline event handler, JavaScript dynamically refers its this
keyword to the global window
object.
There are a couple of ways to fix this issue.
Disable Button in jQuery Using the bind()
Method to Assign the Correct Value to this
We can set the reference of the this
keyword in the onclick
handler’s disable
callback with the bind()
method.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Bind method</title>
<script type="text/javascript" src="jQuery.js"></script>
<link rel="stylesheet" href="disable_button_jQuery.css"/>
<script type="text/javascript">
function disable(e){
console.log("The value of 'this' by explicity specifying it with bind method is : " + this);
$(this).attr("disabled","disabled");
//updating the text to disabled
$(this).html("I am disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable.bind(this)()">Click to disable me</button>
</div>
</body>
</html>
We set the proper context with the line onclick = "disable.bind(this)()"
. As lexically, the this
keyword of the onclick
refers to the button
element, we pass this
to the bind()
method to set this value as the permanent value.
After this, the value of this
does not change at run time.
The rest of the code is similar to our solutions above. Let us look at the output below:
Our code now disables the button as we wanted. Also, the value of this
in the console output now refers to an HTMLButtonElement
.
Gotcha to Watch Out For
Do not forget the pair of brackets after the .bind()
method, like so:
onclick = "disable.bind(this)()"
.bind()
wraps the underlying function in a new function with the new this
value and returns this function. So, make sure you call this wrapper function with the trailing pair of brackets ()
.
Disable Button in jQuery Using the call()
Method to Assign the Correct Value to this
We can also assign the correct value to this
when we call it with the call()
method. Note that call()
only assigns a particular context to this
for the specific function call, while .bind()
fixes the value of this
permanently.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Call method</title>
<script type="text/javascript" src="jQuery.js"></script>
<link rel="stylesheet" href="disable_button_jQuery.css"/>
<script type="text/javascript">
function disable(e){
console.log("The value of 'this' by specicifying it during execution with call method is : " + this);
$(this).attr("disabled","disabled");
//updating the text to disabled
$(this).html("I am disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable.call(this)">Click to disable me</button>
</div>
</body>
</html>
We assign the proper value of this
during execution with the code snippet:
onclick = "disable.call(this)"
We have already broken down the logic of the rest of the code earlier.
Here is the output of this program.
Gotcha to Watch Out For
There are no trailing pair of brackets ()
after the .call()
method here. The .call()
method does not return a new function, like the .bind()
method does.
It only sets the new this
context in the same function call, so we do not need to call it again with the brackets at the end.
Disable Button in jQuery With Element ID Taking Advantage of JavaScript’s Flexible Dynamic Typing
One of the beautiful things about JavaScript is its flexibility. You can get it to do whatever you wish with almost the same code.
The same holds for JavaScript functions. Every function in JavaScript can take how so many arguments we wish to pass in.
We do not need to pass in the same argument data types as in the function definition because JS is a dynamically typed language.
Let us exploit this feature of JavaScript to disable the button in jQuery with the Element ID using almost similar code as we have written above.
We mentioned that the callback to the onclick
accepts only one argument, the event
object, as per its function definition. But we will pass it the element id
argument, and JavaScript won’t complain!
Here is the magical code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Using Dynamic Typing</title>
<script type="text/javascript" src="jQuery.js"></script>
<link rel="stylesheet" href="disable_button_jQuery.css"/>
<script type="text/javascript">
function disable(id){ // replace id with e when you want to display the default event object type
$("#"+id).attr("disabled","disabled");
console.log("The type of argument \nwhen we pass the \n'id' type object as argument is : \n"
+ typeof arguments[0]);
// console.log("The type of argument \nwhen we pass the \ndefault 'event' object as argument is : \n"
// + typeof arguments[0]);
//update the text to disabled
$("#"+id).html("I am disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable('disable_me')">Click to disable me</button>
</div>
</body>
</html>
We pass the id
to the callback to the event handler onclick ="disable('disable_me')"
JavaScript lets us use this argument without complaining that its type is different than the hard-coded event
argument type in the function definition.
We then use it to set the disabled
HTML attribute of the button
element to the value disabled
, like so:
$("#" + id).attr("disabled","disabled");
Let us look at the output:
We see our code works correctly. Also, in the console output to the right, the argument type is a string, which is our element id
passed to the callback.
Let’s also compare the argument type when we pass the default event
type argument.
<script type="text/javascript">
function disable(event){ // replace id with e when you want to display the default //event object type
//$("#"+id).attr("disabled","disabled");
// console.log("The type of argument \nwhen we pass the \n'id' type object as //argument is : \n"
// + typeof arguments[0]);
console.log("The type of argument \nwhen we pass the \ndefault 'event' object as argument is : \n"
+ typeof arguments[0]);
//update the text to disabled
// $("#"+id).html("I am disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable(event)">Click to disable me</button>
</div>
Here is the output:
Here we see the type of the argument in the console is object
as event
is an object in JavaScript.
Disable Button in jQuery With the disabled
DOM Property Using the jQuery .prop()
Method
Lastly, we can also use the disabled
DOM property in all our solutions above. This property corresponds to the HTML disabled
attribute, and we can access it with the jQuery .prop()
method.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Using prop method</title>
<script type="text/javascript" src="jQuery.js"></script>
<script type="text/javascript">
function disable(e){
$(e.currentTarget).prop("disabled",true);
//updating the text to disabled
$(e.currentTarget).html("I am disabled");
}
</script>
<link rel="stylesheet" href="disable_button_jQuery.css">
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable(event)">Click to disable me</button>
</div>
</body>
</html>
The code is almost the same as the one we used for the method using the event
default argument above. We only use the DOM disabled
property, like so:
$(e.currentTarget).prop("disabled",true);
Here is the working demo:
Gotcha to Watch Out For
Note that the disabled
DOM property takes a Boolean value, unlike the disabled
HTML attribute, which takes the value disabled
. So, make sure you pass the value true
in the .prop()
method for this method.