jQuery에서 버튼 비활성화
-
event
객체를onclick
이벤트 처리기에 전달하여 jQuery에서 비활성화 버튼 -
onclick
이벤트 처리기의this
참조를 사용하여 jQuery의 비활성화 버튼 -
bind()
메서드를 사용하여this
에 올바른 값을 할당하는 jQuery의 비활성화 버튼 -
call()
메서드를 사용하여this
에 올바른 값을 할당하는 jQuery의 비활성화 버튼 - JavaScript의 유연한 동적 입력을 활용하는 요소 ID가 있는 jQuery의 비활성화 버튼
-
jQuery
.prop()
메서드를 사용하여disabled
DOM 속성이 있는 jQuery의 비활성화 버튼
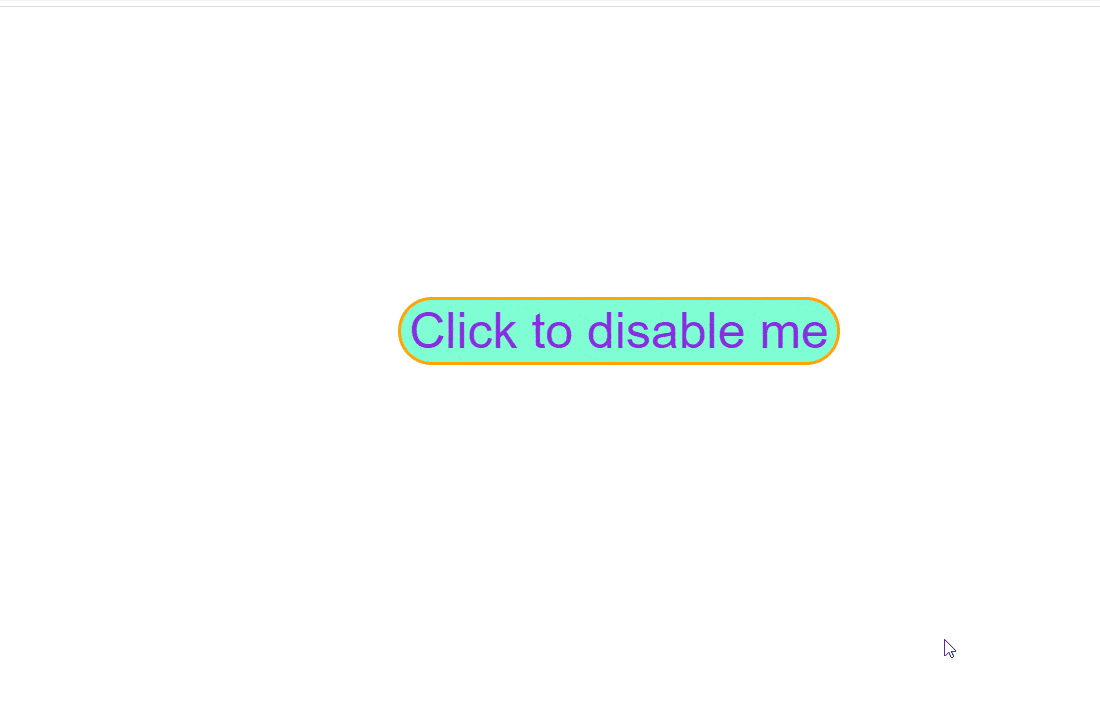
이 자습서에서는 event
개체, this
참조 및 element id
와 같은 다른 인수와 함께 onclick
이벤트 핸들러를 사용하여 jQuery에서 버튼을 비활성화합니다. 또한 여기에서 event.target
대 event.currentTarget
및 jQuery .prop()
대 .attr()
메소드의 동적 타이핑에 대한 팁을 찾을 수 있습니다.
event
객체를 onclick
이벤트 처리기에 전달하여 jQuery에서 비활성화 버튼
onclick
이벤트 핸들러는 대상 요소를 클릭할 때마다 실행됩니다. 기본적으로 onclick
에 대한 콜백은 event
객체라는 하나의 인수만 사용합니다.
이 기본 인수를 jQuery에서 버튼을 비활성화하는 첫 번째 방법으로 사용하겠습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Default event argument</title>
<script type="text/javascript" src="jQuery.js"></script>
<script type="text/javascript">
function disable(e){
$(e.currentTarget).attr("disabled","disabled");
//updating the text to disabled
$(e.currentTarget).html("I am disabled");
}
</script>
<link rel="stylesheet" href="disable_button_jQuery.css">
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable(event)">Click to disable me</button>
</div>
</body>
</html>
onclick
이벤트 핸들러를 연결하는 button
요소가 있습니다. 이에 대한 콜백은 기본 event
개체 인수를 사용합니다.
키 코드 행으로 버튼을 비활성화합니다.
$(e.currentTarget).attr("disabled","disabled")
event
개체의 currentTarget
속성은 이벤트 핸들러가 연결된 요소(여기서는 button
요소)를 식별합니다.
마지막으로 jQuery .attr()
메서드를 사용하여 이 버튼
요소의 비활성화된 HTML 속성을 비활성화
로 설정합니다.
또한 버튼
텍스트를 업데이트하여 jQuery .html()
메서드를 사용하여 코드의 다음 줄에서 비활성화 상태를 표시합니다.
다음은 이 솔루션의 작업 데모입니다.
프로 팁:
이벤트 대상
속성은 currentTarget
속성과 유사하지만 이벤트가 발생하는 요소를 참조합니다. 이는 이벤트 버블링의 경우 DOM 요소가 전파될 때 다를 수 있습니다.
따라서 이 경우 currentTarget
속성을 사용하는 것이 좋습니다.
onclick
이벤트 처리기의 this
참조를 사용하여 jQuery의 비활성화 버튼
onclick
이벤트 핸들러의 기본 this
참조 문제
onclick
이벤트 핸들러 내부의 this
키워드는 실행되는 요소를 참조합니다. 그러나 JavaScript this
키워드는 런타임에 컨텍스트를 동적으로 사용한다는 것을 알고 있습니다.
따라서 기본적으로 이 경우 트리거링 요소를 참조하지 않습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Default this Keyword</title>
<script type="text/javascript" src="jQuery.js"></script>
<link rel="stylesheet" href="disable_button_jQuery.css"/>
<script type="text/javascript">
function disable(){
console.log("The value of this by default is : " + this);
$(this).attr("disabled","disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable()">Click to disable me</button>
</div>
</body>
</html>
onclick
콜백 disable
기능 내에서 this
참조를 사용합니다. 이해를 돕기 위해 this
개체의 값도 기록합니다.
아래에서 이 코드의 출력을 살펴보겠습니다.
여기에서 두 가지 일이 발생했습니다.
- 첫째, 코드가 원하는 대로 버튼을 비활성화하지 않았습니다.
- 둘째, 콘솔에서
this
값을 보면window
객체를 참조합니다. 이것은 예상되는 동작입니다.
인라인 이벤트 핸들러의 함수 외부에서 disable
콜백을 실행할 때 JavaScript는 동적으로 this
키워드를 글로벌 window
객체로 참조합니다.
이 문제를 해결하는 몇 가지 방법이 있습니다.
bind()
메서드를 사용하여 this
에 올바른 값을 할당하는 jQuery의 비활성화 버튼
onclick
핸들러의 disable
콜백에서 bind()
메소드를 사용하여 this
키워드의 참조를 설정할 수 있습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Bind method</title>
<script type="text/javascript" src="jQuery.js"></script>
<link rel="stylesheet" href="disable_button_jQuery.css"/>
<script type="text/javascript">
function disable(e){
console.log("The value of 'this' by explicity specifying it with bind method is : " + this);
$(this).attr("disabled","disabled");
//updating the text to disabled
$(this).html("I am disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable.bind(this)()">Click to disable me</button>
</div>
</body>
</html>
onclick = "disable.bind(this)()"
줄을 사용하여 적절한 컨텍스트를 설정합니다. 사전적으로 onclick
의 this
키워드는 button
요소를 참조하므로 this
를 bind()
메서드에 전달하여 이 값을 영구 값으로 설정합니다.
이후에는 this
값이 런타임 시 변경되지 않습니다.
나머지 코드는 위의 솔루션과 유사합니다. 아래 출력을 살펴보겠습니다.
이제 코드는 원하는 대로 버튼을 비활성화합니다. 또한 콘솔 출력의 this
값은 이제 HTMLButtonElement
를 참조합니다.
주의해야 할 점
다음과 같이 .bind()
메서드 뒤의 대괄호 쌍을 잊지 마십시오.
onclick = "disable.bind(this)()"
.bind()
는 새 this
값을 사용하여 기본 함수를 새 함수로 래핑하고 이 함수를 반환합니다. 따라서 후행 대괄호 ()
쌍을 사용하여 이 래퍼 함수를 호출해야 합니다.
call()
메서드를 사용하여 this
에 올바른 값을 할당하는 jQuery의 비활성화 버튼
call()
메서드로 호출할 때 this
에 올바른 값을 할당할 수도 있습니다. call()
은 특정 함수 호출에 대해 특정 컨텍스트를 this
에 할당하는 반면 .bind()
는 this
의 값을 영구적으로 고정합니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Call method</title>
<script type="text/javascript" src="jQuery.js"></script>
<link rel="stylesheet" href="disable_button_jQuery.css"/>
<script type="text/javascript">
function disable(e){
console.log("The value of 'this' by specicifying it during execution with call method is : " + this);
$(this).attr("disabled","disabled");
//updating the text to disabled
$(this).html("I am disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable.call(this)">Click to disable me</button>
</div>
</body>
</html>
코드 조각으로 실행하는 동안 this
의 적절한 값을 할당합니다.
onclick = "disable.call(this)"
우리는 이미 나머지 코드의 논리를 일찍 분해했습니다.
다음은 이 프로그램의 출력입니다.
주의해야 할 점
여기에서 .call()
메서드 뒤에는 후행 대괄호 ()
가 없습니다. .call()
메서드는 .bind()
메서드처럼 새 함수를 반환하지 않습니다.
동일한 함수 호출에서 새 this
컨텍스트만 설정하므로 끝에 대괄호를 사용하여 다시 호출할 필요가 없습니다.
JavaScript의 유연한 동적 입력을 활용하는 요소 ID가 있는 jQuery의 비활성화 버튼
JavaScript의 아름다운 점 중 하나는 유연성입니다. 거의 동일한 코드로 원하는 모든 작업을 수행할 수 있습니다.
JavaScript 함수도 마찬가지입니다. JavaScript의 모든 함수는 전달하려는 인수 수만큼을 취할 수 있습니다.
JS는 동적으로 유형이 지정되는 언어이기 때문에 함수 정의에서와 동일한 인수 데이터 유형을 전달할 필요가 없습니다.
위에서 작성한 것과 거의 유사한 코드를 사용하여 요소 ID로 jQuery의 버튼을 비활성화하기 위해 JavaScript의 이 기능을 활용해 보겠습니다.
onclick
에 대한 콜백은 함수 정의에 따라 event
객체라는 하나의 인수만 허용한다고 언급했습니다. 그러나 id
인수를 전달하면 JavaScript가 불평하지 않습니다!
마법의 코드는 다음과 같습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Using Dynamic Typing</title>
<script type="text/javascript" src="jQuery.js"></script>
<link rel="stylesheet" href="disable_button_jQuery.css"/>
<script type="text/javascript">
function disable(id){ // replace id with e when you want to display the default event object type
$("#"+id).attr("disabled","disabled");
console.log("The type of argument \nwhen we pass the \n'id' type object as argument is : \n"
+ typeof arguments[0]);
// console.log("The type of argument \nwhen we pass the \ndefault 'event' object as argument is : \n"
// + typeof arguments[0]);
//update the text to disabled
$("#"+id).html("I am disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable('disable_me')">Click to disable me</button>
</div>
</body>
</html>
이벤트 핸들러 onclick ="disable('disable_me')"
에 대한 콜백에 id
를 전달합니다.
JavaScript를 사용하면 유형이 함수 정의에서 하드 코딩된 event
인수 유형과 다르다는 불평 없이 이 인수를 사용할 수 있습니다.
그런 다음 이를 사용하여 다음과 같이 button
요소의 disabled
HTML 속성을 disabled
값으로 설정합니다.
$("#" + id).attr("비활성화됨","비활성화됨");
출력을 살펴보겠습니다.
코드가 올바르게 작동하는 것을 볼 수 있습니다. 또한 오른쪽의 콘솔 출력에서 인수 유형은 콜백에 전달된 요소 id
인 문자열입니다.
기본 event
유형 인수를 전달할 때 인수 유형도 비교해 봅시다.
<script type="text/javascript">
function disable(event){ // replace id with e when you want to display the default //event object type
//$("#"+id).attr("disabled","disabled");
// console.log("The type of argument \nwhen we pass the \n'id' type object as //argument is : \n"
// + typeof arguments[0]);
console.log("The type of argument \nwhen we pass the \ndefault 'event' object as argument is : \n"
+ typeof arguments[0]);
//update the text to disabled
// $("#"+id).html("I am disabled");
}
</script>
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable(event)">Click to disable me</button>
</div>
결과는 다음과 같습니다.
여기에서 event
가 JavaScript의 개체이므로 콘솔의 인수 유형이 object
인 것을 볼 수 있습니다.
jQuery .prop()
메서드를 사용하여 disabled
DOM 속성이 있는 jQuery의 비활성화 버튼
마지막으로 위의 모든 솔루션에서 disabled
DOM 속성을 사용할 수도 있습니다. 이 속성은 HTML disabled
속성에 해당하며 jQuery .prop()
메서드를 사용하여 액세스할 수 있습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Using prop method</title>
<script type="text/javascript" src="jQuery.js"></script>
<script type="text/javascript">
function disable(e){
$(e.currentTarget).prop("disabled",true);
//updating the text to disabled
$(e.currentTarget).html("I am disabled");
}
</script>
<link rel="stylesheet" href="disable_button_jQuery.css">
</head>
<body>
<div id="main">
<button id="disable_me" onclick="disable(event)">Click to disable me</button>
</div>
</body>
</html>
코드는 위의 event
기본 인수를 사용하는 메서드에 사용한 것과 거의 동일합니다. 다음과 같이 DOM disabled
속성만 사용합니다.
$(e.currentTarget).prop("disabled",true);
작업 데모는 다음과 같습니다.
주의해야 할 점
disabled
DOM 속성은 disabled
값을 사용하는 disabled
HTML 특성과 달리 부울 값을 사용합니다. 따라서 이 메서드의 .prop()
메서드에서 true
값을 전달해야 합니다.