How to Format Date and Time Using DateTimePicker in jQuery
- Understanding DateTimePicker
- Basic Date Formatting
- Adding Time Formatting
- Setting Date Ranges
- Customizing the DateTimePicker
- Conclusion
- FAQ
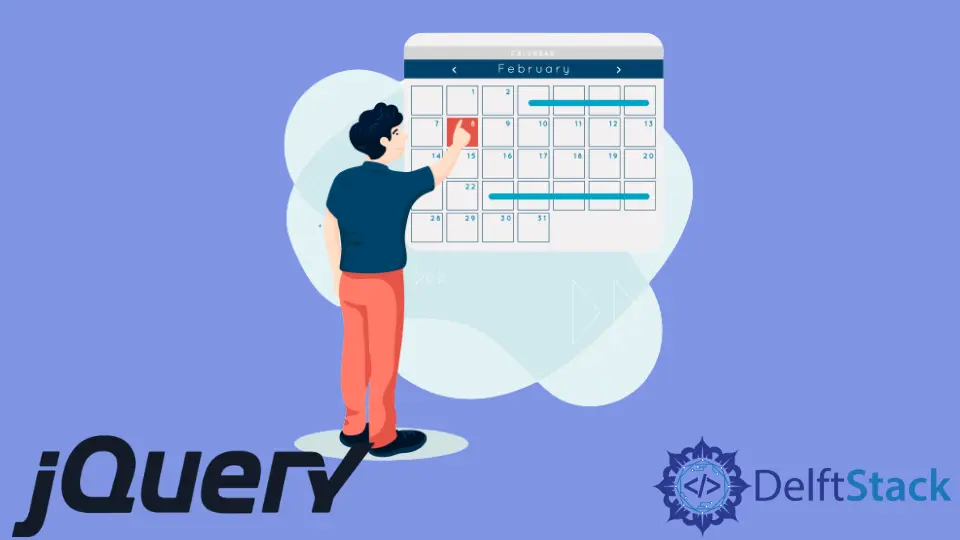
When it comes to web development, managing date and time input can be a tricky task. Fortunately, jQuery provides a powerful tool called DateTimePicker that simplifies this process.
This article will guide you through the steps of formatting date and time using the DateTimePicker plugin in jQuery. Whether you’re building a booking system, an event calendar, or any application that requires date and time input, mastering DateTimePicker will enhance user experience and ensure data accuracy. Let’s dive into the methods and examples that will help you format date and time effectively.
Understanding DateTimePicker
Before we explore the formatting techniques, it’s essential to understand what DateTimePicker is. This jQuery plugin allows users to select both date and time easily. It offers a user-friendly interface that can be customized to fit your application’s needs. You can define formats, restrict date ranges, and even integrate it with other libraries.
To get started, ensure you have included jQuery and the DateTimePicker plugin in your project. Here’s a simple way to include these resources:
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jquery-datetimepicker/2.5.20/jquery.datetimepicker.min.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-datetimepicker/2.5.20/jquery.datetimepicker.full.min.js"></script>
With these scripts in place, you are ready to implement the DateTimePicker in your application.
Basic Date Formatting
The first step in formatting date and time with DateTimePicker is to set the desired date format. You can easily configure this when initializing the DateTimePicker. Here’s how you can do it:
$(document).ready(function(){
$('#datetimepicker').datetimepicker({
format: 'Y-m-d H:i'
});
});
Output:
2023-10-05 14:30
In this code, we set the format to ‘Y-m-d H:i’, which corresponds to a year-month-day hour:minute format. This is a common format used in many applications, especially for database entries. By specifying the format, you ensure that the user input is consistent and easily readable.
Additionally, you can customize the format based on your application’s requirements. For example, you might want to display the date in a more user-friendly format, such as ’d/m/Y H:i’, which would show the date as ‘05/10/2023 14:30’. This flexibility allows you to cater to different audiences and their preferences.
Adding Time Formatting
In addition to basic date formatting, you can also manage how time is displayed. The DateTimePicker allows you to include time in various formats. Here’s an example of how to format the time along with the date:
$(document).ready(function(){
$('#datetimepicker').datetimepicker({
format: 'd/m/Y H:i A'
});
});
Output:
05/10/2023 02:30 PM
In this example, we added ‘A’ to the format string, which converts the time to a 12-hour format and appends AM/PM. This is particularly useful for applications targeting users in regions where the 12-hour clock is the norm.
You can also specify additional options such as limiting the time selection to certain hours or disabling specific dates. This feature enhances user interaction, ensuring they select valid entries.
Setting Date Ranges
Another important aspect of formatting date and time is setting date ranges. This feature allows you to restrict users from selecting dates outside a specified range. Here’s how to implement it:
$(document).ready(function(){
$('#datetimepicker').datetimepicker({
format: 'Y-m-d H:i',
minDate: 0,
maxDate: '+1M'
});
});
Output:
2023-10-05 14:30
In this code snippet, minDate: 0
prevents users from selecting past dates, while maxDate: '+1M'
allows selection only up to one month into the future. This is especially useful in booking applications where you want to ensure that users can only select valid dates.
By controlling the date range, you enhance the usability of your DateTimePicker, making it easier for users to navigate through available options. This not only improves user experience but also minimizes errors in date selection.
Customizing the DateTimePicker
Customization is key to making your DateTimePicker align with your application’s design. You can change colors, button styles, and even the layout. Here’s an example of how to customize the DateTimePicker:
$(document).ready(function(){
$('#datetimepicker').datetimepicker({
format: 'Y-m-d H:i',
theme: 'dark',
step: 15
});
});
Output:
2023-10-05 14:30
In this example, we’ve added a theme
option to apply a dark theme to the DateTimePicker. The step
option allows you to define the increment of time selection, in this case, every 15 minutes. This is particularly useful for applications where appointments are scheduled in short intervals.
Customizing the DateTimePicker not only enhances its functionality but also ensures that it visually integrates with your website or application. A well-designed interface can significantly improve user engagement and satisfaction.
Conclusion
Formatting date and time using the DateTimePicker in jQuery is a straightforward process that can significantly enhance the user experience of your web applications. By mastering the various formatting options, date ranges, and customization features, you can create a robust date and time input system that meets your users’ needs. Whether you are developing a booking system, an event calendar, or any other application requiring date and time input, the DateTimePicker plugin is an invaluable tool in your arsenal.
FAQ
-
How do I include the DateTimePicker plugin in my project?
You can include it by adding the necessary CSS and JavaScript links for jQuery and the DateTimePicker plugin in your HTML file. -
Can I customize the DateTimePicker appearance?
Yes, you can customize the appearance by using various options like themes, colors, and button styles. -
Is it possible to restrict date selection in DateTimePicker?
Absolutely! You can set minimum and maximum date limits using theminDate
andmaxDate
options.
-
What formats can I use for date and time?
The DateTimePicker supports various formats such as ‘Y-m-d H:i’, ’d/m/Y H:i A’, and many more, allowing you to tailor it to your needs. -
Can I use DateTimePicker for time-only selection?
Yes, you can configure the DateTimePicker to focus solely on time selection by adjusting the format and disabling date selection.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn