How to Check jQuery Version in a User Browser
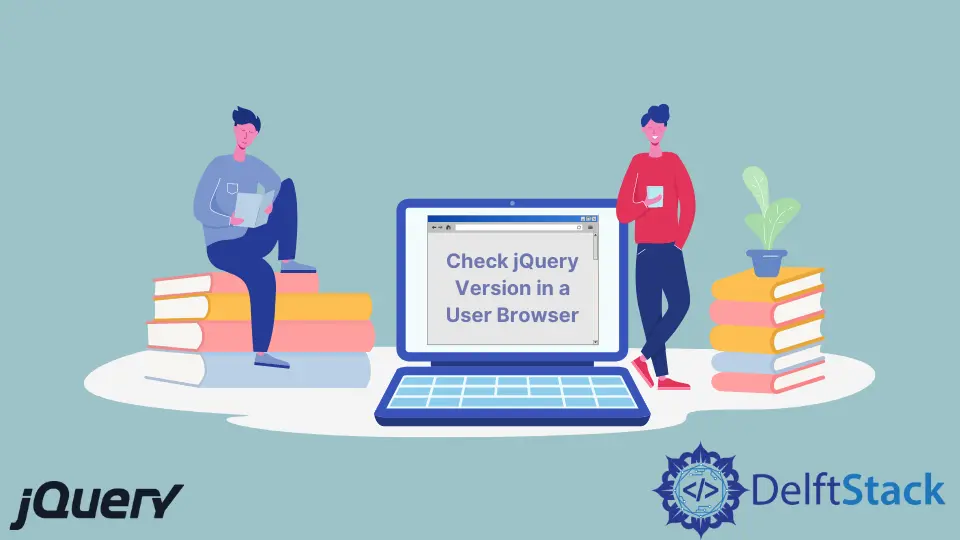
This article teaches two methods to get a jQuery version on a web page. The first method checks the version if the web page has loaded jQuery, while the second method checks the version if jQuery exists in the window
object.
Get jQuery Version Using the typeof
Operator
When a web page loads the jQuery library, it becomes available via the jQuery
keyword or the dollar ( $
) sign. With this, you can check if $
or jQuery
exists using the typeof
operator.
If the check returns true
, jQuery exists in the web browser, so you can print the version number.
That’s what we’ve done in the following code. What follows is the output in a web browser.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-get-jQuery-version</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<script>
// Check if jQuery exists using the typeof
// operator. The code in the if block will
// run ONLY if jQuery exists in the user's
// web browser
if (typeof jQuery !== undefined) {
// Show the jQuery version number in the
// alert dialog box.
alert("The current jQuery version is: " + jQuery.fn.jquery);
}
</script>
</body>
</html>
Output:
Get jQuery Version Using the window
Object
If a web browser has loaded the jQuery library, its alias becomes available in the window
object. With this knowledge, you can check jQuery in the window
object and get the version number.
Unlike our previous example, we’ll get the version using jQuery().jquery
. Mind you, this will create an object, so if you don’t want that, use our first example.
In the following, we check if the window
object contains jQuery before we print the version.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-get-jQuery-version</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
body {
background-color: #1a1a1a;
}
</style>
</head>
<body>
<script>
// Check if jQuery exists in the window
// object.
if (window.jQuery) {
// Show the jQuery version number in the
// alert dialog box.
alert("The current jQuery version is: " + jQuery().jquery);
}
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn