Select Onchange in JavaScript
- Understanding the onChange Event
- Setting Up the HTML Structure
- Implementing the onChange Event in JavaScript
- Handling Multiple Select Elements
- Conclusion
- FAQ
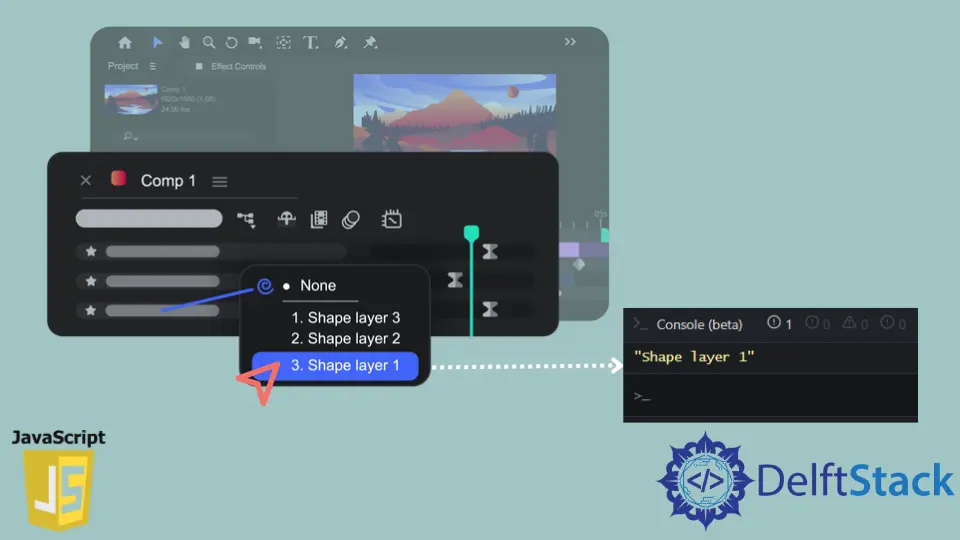
In today’s post, we’ll learn how to detect the onChange event of the select element in JavaScript. This functionality is essential for creating dynamic web applications that respond to user input. Whether you’re building forms, filters, or dropdown menus, understanding how to handle the onChange event can significantly enhance user experience. By the end of this article, you’ll have a solid grasp of how to implement this event in your projects. So, let’s dive into the world of JavaScript and explore how to effectively manage the onChange event for select elements.
Understanding the onChange Event
The onChange event is a built-in JavaScript event that triggers when the value of a select element changes. This event is particularly useful for forms where users need to select options from a dropdown menu. When a user selects a new option, the onChange event fires, allowing you to execute a specific function or set of instructions in response.
To effectively utilize the onChange event, you can use JavaScript’s event listeners. By adding an event listener to a select element, you can specify what actions should take place when the user selects a different option. This could involve updating other elements on the page, making API calls, or triggering animations.
Setting Up the HTML Structure
Before we dive into the JavaScript code, let’s set up a simple HTML structure that includes a select element. This will serve as our base for implementing the onChange event.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Select Onchange Example</title>
</head>
<body>
<label for="options">Choose an option:</label>
<select id="options">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
<option value="option3">Option 3</option>
</select>
<div id="output"></div>
<script src="script.js"></script>
</body>
</html>
This HTML code creates a dropdown menu with three options and a div to display the selected output. Now, let’s move on to the JavaScript part.
Implementing the onChange Event in JavaScript
Now that we have our HTML structure, let’s implement the onChange event in JavaScript. We’ll add an event listener to our select element that listens for changes and updates the output div accordingly.
document.getElementById('options').addEventListener('change', function() {
const selectedValue = this.value;
document.getElementById('output').innerText = `You selected: ${selectedValue}`;
});
In this code, we first select the dropdown menu using getElementById
. We then attach an event listener that listens for the change
event. Inside the event listener, we retrieve the selected value using this.value
and update the inner text of the output div to reflect the user’s choice.
Output:
You selected: option1
This simple implementation allows the user to see their selection immediately after changing the dropdown option. It enhances user interaction and provides instant feedback.
Handling Multiple Select Elements
What if your application requires handling multiple select elements? You can easily extend the previous example to accommodate this. Let’s modify our HTML to include another select element.
<label for="options1">Choose your favorite fruit:</label>
<select id="options1">
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="cherry">Cherry</option>
</select>
<label for="options2">Choose your favorite color:</label>
<select id="options2">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
<div id="output1"></div>
<div id="output2"></div>
Now, let’s implement the JavaScript code to handle both select elements.
document.getElementById('options1').addEventListener('change', function() {
const selectedFruit = this.value;
document.getElementById('output1').innerText = `You selected fruit: ${selectedFruit}`;
});
document.getElementById('options2').addEventListener('change', function() {
const selectedColor = this.value;
document.getElementById('output2').innerText = `You selected color: ${selectedColor}`;
});
In this updated code, we add separate event listeners for each select element. When a user changes their selection, the corresponding output div updates to reflect the selected fruit or color.
Output:
You selected fruit: apple
You selected color: red
By implementing multiple event listeners, you can manage various select elements in your application seamlessly.
Conclusion
Detecting the onChange event of select elements in JavaScript is a straightforward yet powerful technique to enhance user interaction on your web pages. By utilizing event listeners, you can create dynamic and responsive applications that react to user selections in real-time. Whether you’re working with a single select element or multiple dropdowns, mastering this functionality will greatly improve the overall user experience. So, get out there and start implementing the onChange event in your projects!
FAQ
-
What is the onChange event in JavaScript?
The onChange event is triggered when the value of a select element changes, allowing you to execute specific actions in response. -
How do I attach an event listener to a select element?
You can use theaddEventListener
method to attach an event listener to a select element, specifying the event type (like ‘change’) and the function to execute. -
Can I handle multiple select elements with onChange?
Yes, you can attach separate event listeners to multiple select elements to handle changes independently. -
What is the difference between onChange and onInput events?
The onChange event triggers when the user changes the value and moves away from the element, while the onInput event triggers immediately as the user types or changes the value.
- How can I display the selected value of a dropdown?
You can retrieve the selected value usingthis.value
within the event listener and then display it in another element on the page.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn