How to Reverse a String in JavaScript
- Reverse a String Using Built-In Functions in JavaScript
- Reverse a String Using Recursion in JavaScript
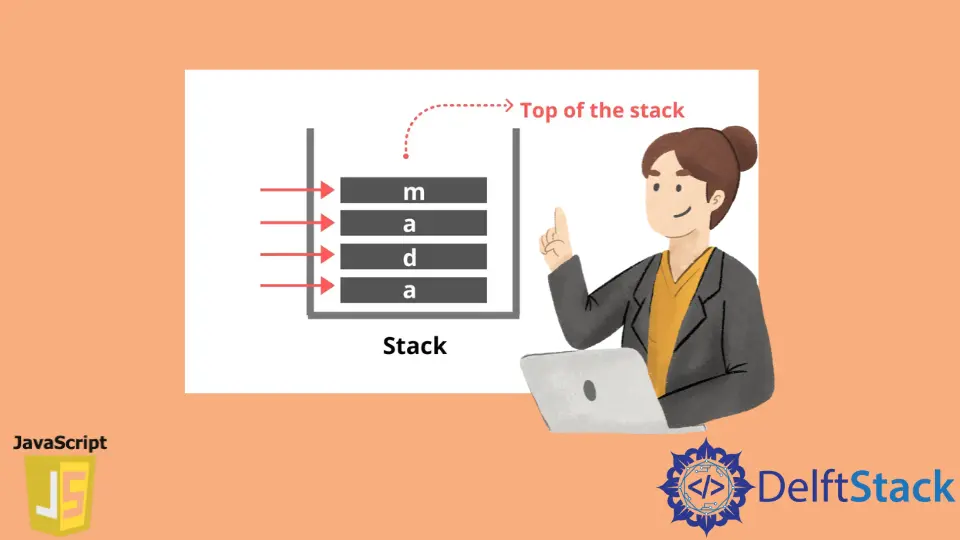
There are various ways in which you can reverse a string in JavaScript in place without using any additional space. The advantage of this is that you can write a program with O(1)
space complexity. It means that you don’t need any extra space in memory for storing the data. You only use the same space provided to us i.e, the size of the input, and reverse the given string within that space.
Below are some of the ways with which you can reverse a string in JavaScript.
Reverse a String Using Built-In Functions in JavaScript
Let’s see how to reverse a string in place with the help of built-in functions available in JavaScript. There are 3 functions using which you can achieve this and those functions - split()
, reverse()
and join()
.
Below is what each of the three functions will do.
split()
will separate each character of a string and convert it into an array.reverse()
will take that array and reverse the elements inside it.join()
will join the characters that have been reversed by thereverse()
function.
function reverse(s) {
return s.split('').reverse().join('');
}
var sss = reverse('adam')
console.log(sss)
Output:
mada
To reverse a string, you first have to apply the split()
function on the input string. This function converts the string into an object which contains all the characters of that string. After that, you can use the built-in JavaScript function reverse()
to reverse a string. This function doesn’t work directly with the strings, so you must first convert the string into an object using the split()
function.
Now you have an object inside which all the characters are in reversed order. To convert this object back to a string, you can use the join()
function. This function will take each character from the string and will join it to form a string.
Reverse a String Using Recursion in JavaScript
Another way of reversing a string is to use recursion. Here, you have created a function named reverse()
, which takes str
as an argument. While writing a recursive function, you first have to make sure that you add a base condition. A base condition allows us to terminate a recursive program during the execution. If there is no basic condition in the program, it will run infinitely, which we don’t want. That’s the reason why you need to check if the string which a user is passing to this function is empty or not. If it is empty, then you will terminate the program; otherwise, you will perform recursive calls.
function reverse(str) {
if (str === '') {
return '';
} else {
return reverse(str.substr(1)) + str.charAt(0);
}
}
let reverseStringIs = reverse('adam')
console.log(reverseStringIs)
Output:
mada
In the else
part, we only have one line of code. You can further think that you have divided this line of code into 2 parts (this is just for assumption so that you can understand the code). The first part is the reverse(str.subset(1))
and the second part is str.charAt(0)
.
The first part takes the entire adam
and takes its subset starting from index no. 1 i.e dam
because d
is at the first position. And the second part takes the entire string i.e adam
, and gets the character at index 0 i.e a
.
We perform a recursion call and then pass this substring to the reverse()
function i.e dam
and not adam
, since you have already created a substring from the original string. Now when you run the else
part, you will have dam
as the input string. You will create a substring of this string using the substr()
function starting from index 1. And the substring will be am
, and the str.charAt(0)
will give us the character at index 0 and i.e d
.
This process continues till the entire string becomes empty. At this point, this is how our stack will look like.
This is the sequence in which the characters will be returned from the stack - m, a, d, a
. As soon as the string becomes empty, the program will start popping the elements one by one from the stack, and you will get the final string in reverse order, madam
, as an output.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn