How to Play Audio Files in JavaScript
-
Use
.play()
to Play Audio Files in JavaScript - Use the Web Audio API to Play Audio Files
-
Use the
howler.js
Library to Play Audio Files in JavaScript
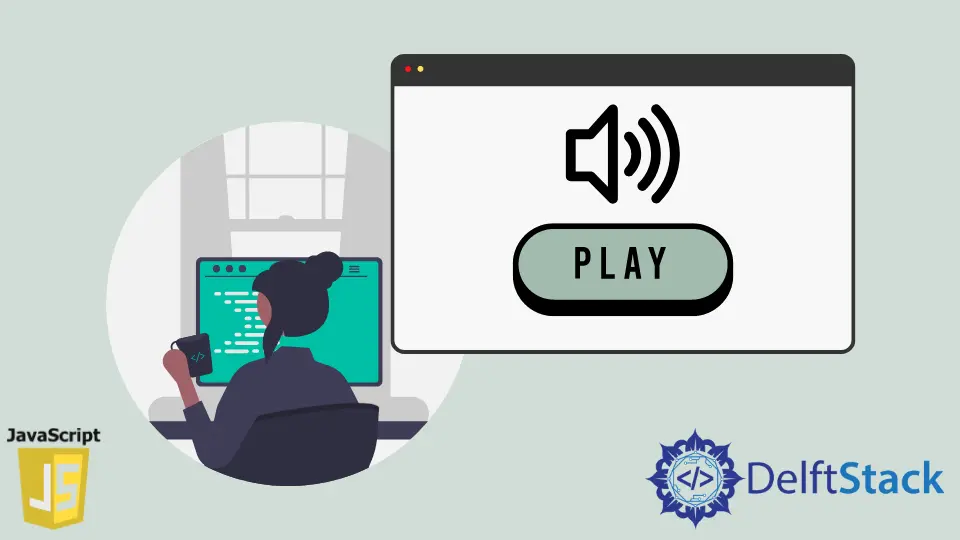
In this article, we will learn how to play audio files in JavaScript.
We can add audio files to our page simply by using the <audio>
tag. It is the easiest way to play audio files without involving JavaScript at all. The src
attribute of the <audio>
tag specifies the audio file’s address. It also has other helpful attributes like control
, autoplay
, and loop
. But there are times when we want to take control automatically and play sounds automatically, like in a game, when we click, or any other event. In such situations, we want JavaScript to take control and play files according to our logic.
Use .play()
to Play Audio Files in JavaScript
We can load an audio file in JavaScript simply by creating an audio object instance, i.e. using new Audio()
. After an audio file is loaded, we can play it using the .play()
function.
const music = new Audio('adf.wav');
music.play();
music.loop = true;
music.playbackRate = 2;
music.pause();
qqazszdgfbgtyj
In the above code, we load an audio file and then simply play it. JavaScript provides us with a lot of flexibility and tons of features. We can control the playback rate, loop the audio, pause, and play the sound. The only problem is handling multiple sounds at once and somewhat limited control compared to the latest sophisticated.
Use the Web Audio API to Play Audio Files
Although a bit cumbersome to set up, Web Audio API provides us with a lot of flexibility and control over the sound. It is a significant advancement from the typical HTML5 audio and allows complex audio manipulation. It uses HTML5 audio to represent the audio elements as nodes on a directed graph-like structure called the audio context. There can be many types of nodes like volume nodes connected between the audio source and destination and help us manipulate volume.
<audio src='audio_file.mp3'></audio>
const audioContext = new AudioContext();
const element = document.querySelector(audio);
const source = audioContext.createMediaElementSource(element);
source.connect(audioContext.destination)
audio.play();
Here, we first initialize an audio context and get the reference to the source of the audio file. We then connect that source to a global destination, and our audio setup is done.
Use the howler.js
Library to Play Audio Files in JavaScript
howler.js
is an audio manipulation library. It allows us to harness the power of Web Audio API and the simplicity of HTML 5 Audio. It is widely used with react class components to handle browser-based audio, especially while playing multiple audio sources. It enables the control of global audio context using a Howler object and then controls audio or a group of audios using Howl.
<script src="https://cdnjs.cloudflare.com/ajax/libs/howler/2.2.1/howler.min.js"></script>
<script>
var sound = new Howl({
src: ['https://www.soundhelix.com/examples/mp3/SoundHelix-Song-1.mp3'],
volume: 1.0,
onend: function () {
alert('We finished with the setup!');
}
});
sound.play()
</script>
All the major browsers except Internet Explorer support all these methods. Internet Explorer does not have support for Web Audio API and howler.js
.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn