How to Create a Beep in JavaScript
- Create a Beep in JavaScript
-
Use
audio
HTML Tag for Beep in JavaScript -
Use
audio
Constructor Function for Beep in JavaScript
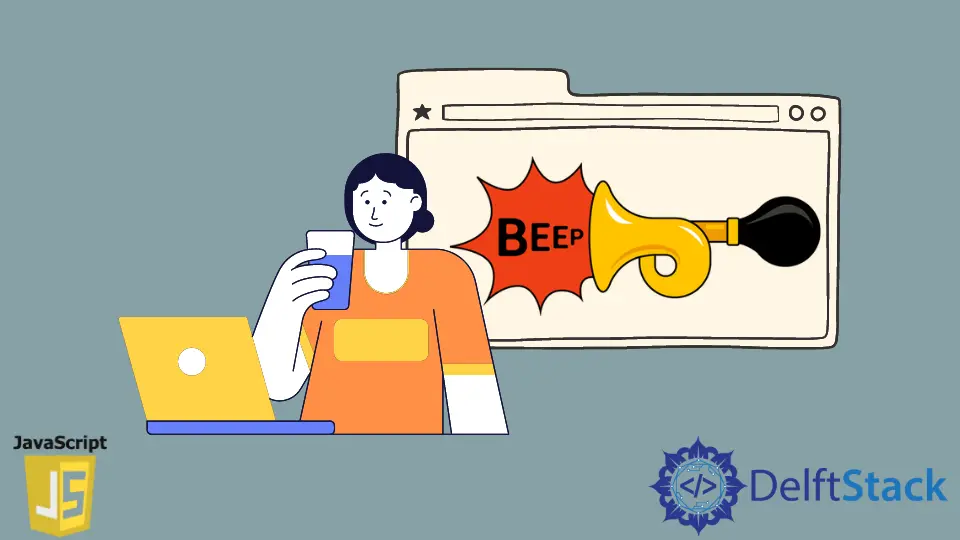
This article shows two ways to create a beep in JavaScript.
Create a Beep in JavaScript
A beep usually works as an alert for certain functionality. But the general JavaScript convention does not have any specific method or property to follow such action.
There are two ways of performing the task. One is to interact with the HTML tag, and the other way is to operate in the script
tag.
Here we will see the examples to add an audio source in the HTML tag. We will also see another instance that takes the URL of a beep in the audio constructor function and later gets triggered after action.
Use audio
HTML Tag for Beep in JavaScript
We need to add a source for the sound in the HTML audio
tag. We add a button element to trigger a function playbeep()
when defining the audio and source structure.
We will take the audio element and perform its function in the script section. When it gets fired by sound.play()
, it creates a sound (beep) corresponding to the source attached.
Code Snippet:
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<p>Press the Button</p>
<audio id="Audio" >
<source src="https://www.soundjay.com/misc/censor-beep-01.mp3"
type="audio/mpeg">
</audio>
<button onclick="playbeep()">Press Here!</button>
<script>
var sound = document.getElementById('Audio');
function playbeep(){
sound.play()
}
</script>
</body>
</html>
Output:
Use audio
Constructor Function for Beep in JavaScript
The JavaScript Audio
constructor takes the sound source. In the HTML part, all it takes is a button followed by an onclick
function, and whenever it is called, the sound
object that takes the URL gets triggered.
Code Snippet:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h2>Press the Button</h2>
<button onclick="playbeep()">Press Here!</button>
<script>
function playbeep() {
var sound = new Audio('https://www.soundjay.com/misc/censor-beep-01.mp3');
sound.play();
}
</script>
</body>
</html>
Output: