How to Check String is Palindrome in JavaScript
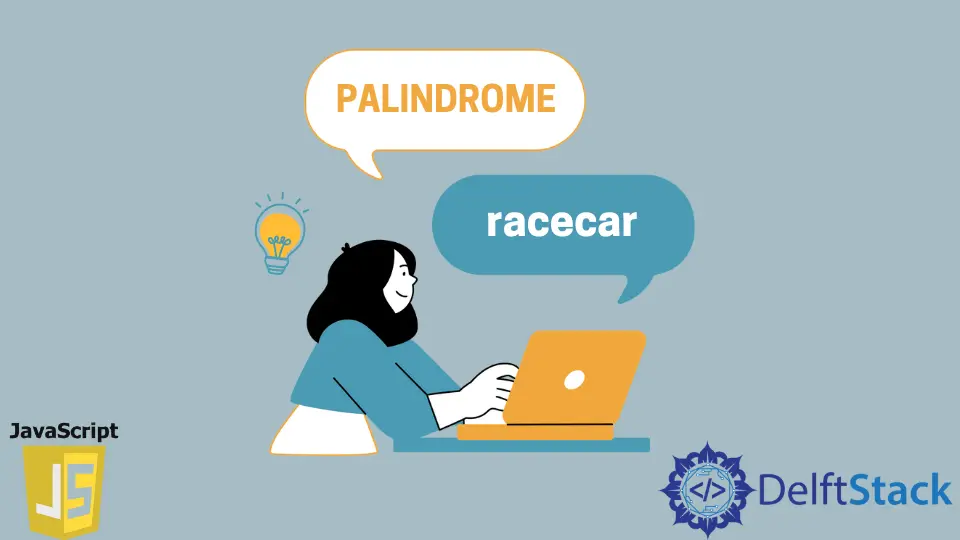
A palindrome is a word, number, phrase, or other sequence of characters that can be read backward and forwards, like adida
or racecar
. There are also numeric palindromes, including date and time stamps using short digits.
For example, 11 November 2011
is considered a palindrome day 111111
using the dd-mm-yy
format as it can be read from left to right or vice versa. Sentence-length palindromes ignore punctuation, capitalization, and word boundaries.
This article teaches us to check if string/number is a palindrome in JavaScript.
Palindrome in JavaScript With Built-in Functions
JavaScript provides several functions that we can use to detect whether a string is a palindrome or not.
-
First, convert the string into an array using the
string.split()
function.The
split()
method is a built-in method provided by JavaScript. This method splits the string into a list of substrings.This cut is performed by taking the separator as an input.
This separator is purely an optional input. And based on that, all these substrings are placed in an array, and the array is returned.
You can also pass limit as input to the method, limiting the number of substrings in the array.
-
Second, use the
reverse()
method to reverse the array’s values.The
reverse()
method is a built-in method provided by JavaScript. This method reverses an original array instead.The first element of an array becomes the last element of an array, and the array’s last element becomes the first element.
-
Last, use the
join()
method to group values of the array into the string.The
join()
method is a built-in method provided by JavaScript. This method creates and returns a new string by concatenating all the elements in an array (or array-like object), separated by commas or a specified delimiter string.If the array has only one element, that element is returned without using the delimiter.
Let’s combine all the above methods and check if the input string is a palindrome.
function palindromeFn(inputString) {
return inputString == inputString.split('').reverse().join('');
}
console.log(palindromeFn('aviddiva'))
console.log(palindromeFn('Hello'))
If we run the above code in any browser, it will show the following result.
Output:
"true"
"false"
Palindrome in JavaScript With for
Loop
Another method to check if a string is a palindrome or not is by using a for
loop. Below are the steps to check if a string is a palindrome in JavaScript.
-
First, take a temporary variable containing the string’s length to be checked.
-
Apply the
for
loop and set the condition to check for half the length of the string. -
Compare the original string character to the inverted string character.
-
If the forward and backward characters are the same, the string or number is a palindrome. Otherwise, the specified string or number is not a palindrome.
function palindromeFn(string) {
const stringLength = string.length;
for (let i = 0; i < stringLength / 2; i++) {
if (string[i] !== string[stringLength - 1 - i]) {
return 'It is not a palindrome.';
}
}
return 'It is a palindrome.';
}
console.log(palindromeFn('aviddiva'));
console.log(palindromeFn('Hello'));
If we run the above code in any browser, it will show the following result.
Output:
"It is a palindrome."
"It is not a palindrome."
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn