How to Wait for Page to Load in JavaScript
- Understanding the Window Load Event
- Using the DOMContentLoaded Event
- Using Promises to Wait for Page Load
- Using jQuery for Page Load Management
- Conclusion
- FAQ
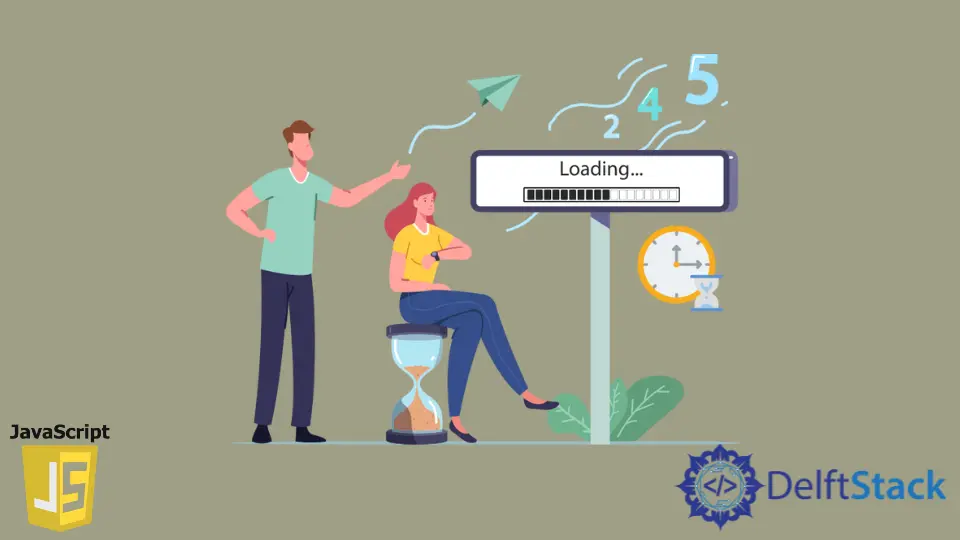
In today’s digital landscape, ensuring that your web pages load seamlessly is crucial for user experience and retention. But how can you effectively wait for a page to load entirely in JavaScript? Understanding this concept is vital for web developers looking to enhance their applications.
In this article, we’ll explore various methods to handle page loading events in JavaScript, ensuring that your scripts execute only when the entire page is ready. Whether you’re dealing with images, scripts, or external resources, mastering these techniques will elevate your web development skills. So, let’s dive in and discover how to wait for a page to load in JavaScript!
Understanding the Window Load Event
One of the simplest ways to wait for a page to load is by using the window.onload
event. This event triggers when the entire page, including all dependent resources, has completed loading. This is particularly useful when you want to ensure that all elements are available before executing your scripts.
Here’s how you can implement the window.onload
event:
window.onload = function() {
console.log("Page fully loaded");
};
Output:
Page fully loaded
In this code snippet, we assign a function to window.onload
. When the page fully loads, the message “Page fully loaded” is logged to the console. This method is straightforward and effective for most use cases. However, keep in mind that if you have multiple scripts that need to wait for the page load, they may overwrite each other. Thus, it’s often better to use event listeners for more complex scenarios.
Using the DOMContentLoaded Event
An alternative to window.onload
is the DOMContentLoaded
event. This event triggers when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading. This is particularly useful for scripts that don’t rely on images or external resources.
Here’s an example of how to use DOMContentLoaded
:
document.addEventListener("DOMContentLoaded", function() {
console.log("DOM fully loaded and parsed");
});
Output:
DOM fully loaded and parsed
In this example, we add an event listener for DOMContentLoaded
. When the DOM is fully loaded and parsed, the message “DOM fully loaded and parsed” is logged. This method is generally preferred for executing scripts as soon as the DOM is ready, allowing for faster interactions. It’s especially beneficial for improving the perceived performance of your web applications.
Using Promises to Wait for Page Load
With the advent of modern JavaScript, using Promises to manage asynchronous operations has become increasingly popular. You can create a Promise that resolves when the page has fully loaded. This approach is particularly useful when you want to chain multiple operations that depend on the page load.
Here’s how you can implement this:
function waitForPageLoad() {
return new Promise((resolve) => {
window.onload = resolve;
});
}
waitForPageLoad().then(() => {
console.log("Page load complete using Promise");
});
Output:
Page load complete using Promise
In this code, we define a function waitForPageLoad
that returns a Promise. The Promise resolves when the window.onload
event triggers. By calling waitForPageLoad()
, we can then chain a .then()
method to execute code after the page has fully loaded. This method is particularly useful for more complex applications where you need to ensure that multiple asynchronous actions are completed before proceeding.
Using jQuery for Page Load Management
If you are working with jQuery, it provides a simplified way to wait for the page to load. The $(document).ready()
method is specifically designed to run code when the DOM is fully loaded. This is similar to the DOMContentLoaded
event but is tailored for jQuery users.
Here’s how to use it:
$(document).ready(function() {
console.log("jQuery DOM is ready");
});
Output:
jQuery DOM is ready
In this example, we use jQuery’s $(document).ready()
method to execute a function once the DOM is ready. This method is very efficient and is widely used in jQuery-based applications. It simplifies the process of ensuring that your scripts run at the right time, making it a popular choice among developers.
Conclusion
Waiting for a page to load in JavaScript is crucial for ensuring that your scripts execute only when all elements are available. Whether you choose to use the window.onload
event, the DOMContentLoaded
event, Promises, or jQuery, each method has its strengths and use cases. By mastering these techniques, you can enhance user experience, improve performance, and create more robust web applications. So, go ahead and implement these methods in your projects, and watch your web development skills flourish!
FAQ
-
What is the difference between window.onload and DOMContentLoaded?
window.onload waits for the entire page, including images and resources, to load, while DOMContentLoaded triggers when the HTML is fully loaded and parsed. -
Can I use Promises to manage page load events?
Yes, you can create a Promise that resolves when the page load event occurs, allowing for more complex asynchronous operations. -
Is jQuery necessary for waiting for page load?
No, jQuery is not necessary, but it simplifies the process for those who prefer using it in their projects. -
Which method is the best for waiting for page load?
The best method depends on your specific use case. For quicker interactions,DOMContentLoaded
is preferable, whilewindow.onload
is suitable for waiting for all resources. -
Can I use multiple event listeners for page load?
Yes, you can use multiple event listeners, but be cautious of overwriting thewindow.onload
event if you use that method.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn