How to Add Vector Class in JavaScript
-
Add Vector Class Using a
for
Loop in JavaScript -
Add Vector Class Using
Array.proyotype.map()
in JavaScript - Add Vector Class Using an ES6 Class in JavaScript
- Add Vector Class by Extending the Array Class in JavaScript
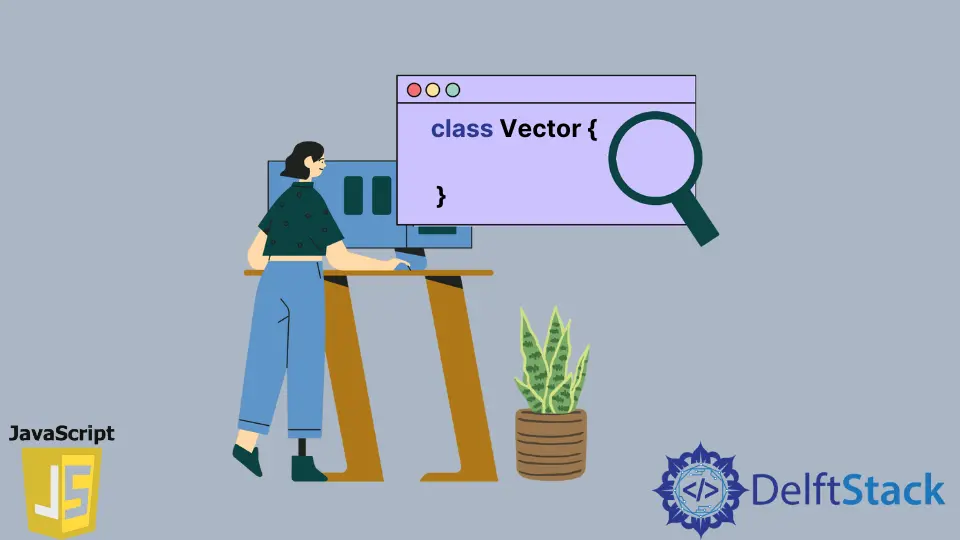
This tutorial will teach you how to add two vectors in JavaScript using different methods.
Add Vector Class Using a for
Loop in JavaScript
You can use the for
loop to add two vectors in JavaScript. Meanwhile, the vectors should be JavaScript arrays.
Define a constructor function that’ll perform the addition and return the result to get started. It’s best to write the addition function separately from the constructor function to make the code clean and reusable in other projects.
The addition function should work so that the first vector can call it on the second one.
Therefore, the first vector is the caller, and the second is the called. As a result, the addition function should work as follows:
- Ensure the arguments are arrays of the same length. The arguments are the first vector (the caller) and the second array (the called).
- Construct an empty array to store the final result of the vector addition.
- Loop through the caller array.
- Use the loop index to add the caller array and the called array elements.
- Push the result into the empty array created from Step 1. So, it is no longer empty because it has the result of the addition.
- Return a new instance of the constructor function with the new array.
- If something went wrong along the way, return an error.
The code below is the implementation of these steps. We’ve implemented additional checks that ensure the user passes the correct vector value.
We’ve added two vectors to test the vector addition and logged the result to the web browser’s console.
Code:
function Vector(arg) {
this.array = arg;
this.add = add;
}
function add(called_array) {
if (Array.isArray(this.array) && Array.isArray(called_array.array)) {
if (this.array.length === called_array.array.length) {
let result = [];
for (let i = 0; i < this.array.length; i++) {
if (typeof (this.array[i]) == 'number' &&
typeof (called_array.array[i]) == 'number') {
result.push(this.array[i] + called_array.array[i]);
} else {
result.push('Invalid vector value');
}
}
return new Vector(result);
} else {
return new Vector('The vectors are not the same length.');
}
} else {
return new Vector('One of your arguments or both of them is not an array.');
}
}
let caller_array = new Vector([3, 5, 7]);
let called_array = new Vector([5, 2, 9]);
console.log(caller_array.add(called_array).array);
Output:
Array(3) [ 8, 7, 16 ]
Add Vector Class Using Array.proyotype.map()
in JavaScript
Adding vectors using Array.prototype.map()
requires the vectors themselves to be JavaScript arrays. Similar to the previous section, the first array should be the caller.
This means you’ll define a constructor function that’ll aid the addition. However, we won’t define the add()
function separately from the constructor function.
This time, we’ll make the add()
function part of the constructor function prototype.
The add()
function will return a new instance of the constructor function. However, the argument of this new instance is the result of the caller array on the called array using Array.prototype.map()
with a custom function.
The custom function performs the addition of the arrays.
We used Array.prototype.map()
to add the vectors in the code below. We checked if the arguments were arrays of the same length during the process.
Anything other than that, the code throws a TypeError
.
Code:
var Vector = function(arg) {
this.array = arg;
};
Vector.prototype.add =
function(called_array) {
called_array = called_array.array;
let caller_array = this.array;
if (Array.isArray(caller_array) && Array.isArray(called_array)) {
if (caller_array.length !== called_array.length) {
throw new TypeError('Vectors have different length');
}
} else {
return new Vector('One of your arguments or both of them is not an array.');
}
return new Vector(
caller_array.map(function(caller_array_element, called_array_element) {
if (typeof (caller_array_element) == 'number' &&
typeof (called_array_element) == 'number') {
return caller_array_element + called_array[called_array_element];
} else {
return 'Invalid Vector value';
}
}));
}
let caller_array = new Vector([9, 8, 5]);
let called_array = new Vector([2, 5, 8]);
console.log(caller_array.add(called_array).array);
Output:
Array(3) [ 11, 13, 13 ]
Add Vector Class Using an ES6 Class in JavaScript
The fundamental logic behind using an ES6 class to add vectors is a custom add()
function, which adds the vectors using a for
loop and returns a new instance of the ES6 class. Before adding the vectors, ensure that you store them in an array.
Afterward, the first array should call the add()
function on the second array.
We have an ES6 class in the following code that adds two arrays using an add()
function. After the addition, it passes the result of the addition to a new instance of the ES6 class.
Code:
class Vector {
constructor(arr) {
this.arr = arr;
}
add(called_array) {
let caller_array = called_array.arr;
if (Array.isArray(this.arr) && Array.isArray(caller_array)) {
if (this.arr.length === caller_array.length) {
let result = [];
for (let key in this.arr) {
if (typeof (this.arr[key]) == 'number' &&
typeof (caller_array[key]) == 'number') {
result[key] = this.arr[key] + caller_array[key];
} else {
result[key] = 'Invalid Vector Value.';
}
}
return new Vector(result);
} else {
return new Vector('The vectors are not the same length.');
}
} else {
return new Vector(
'One of your arguments or both of them is not an array.');
}
}
}
let caller_array = new Vector([3, 6, 0]);
let called_array = new Vector([1, 5, 0]);
console.log(caller_array.add(called_array).arr);
Output:
Array(3) [ 4, 11, 0 ]
Add Vector Class by Extending the Array Class in JavaScript
You can create a vector class that extends JavaScript native Array class. So, the vector class should implement a function that’ll add the vectors.
The first vector can call the function on the second vector. As a result, you’ll get the result of the addition.
In the following code, we have extended the native Array class and used the map()
function to step through the called array. Then, we can add its element to the caller array and produce a result.
Code:
class Vector extends Array {
add(called_array) {
if (this.length == called_array.length) {
return this.map((caller_array_element, called_array_element) => {
if (typeof (caller_array_element) == 'number' &&
typeof (called_array_element) == 'number') {
return caller_array_element + called_array[called_array_element];
} else {
return 'Invalid Vector value';
};
})
} else {
return 'The vectors are not the same length.';
}
}
}
let caller_array = new Vector(1, 2, 4);
let called_array = new Vector(3, 9, 20);
console.log(caller_array.add(called_array));
Output:
Array(3) [ 4, 11, 24 ]
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn