JavaScript Equivalent of var_dump() in PHP
- Understanding var_dump() in PHP
- Using console.log() for Debugging
- Using console.dir() for Better Structure
- Using JSON.stringify() for String Representation
- Conclusion
- FAQ
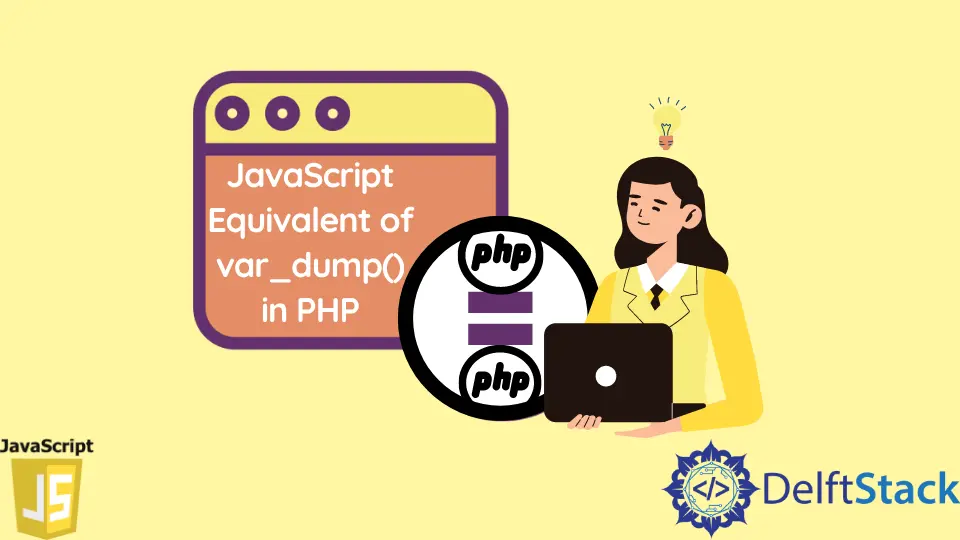
In the world of web development, debugging is an essential skill. PHP developers often rely on var_dump()
to inspect variables and see their structure and values. But what about JavaScript? If you’re transitioning between these two languages or simply looking for a similar debugging tool in JavaScript, you’ve come to the right place.
In this article, we’ll explore the JavaScript equivalent of var_dump()
in PHP, discuss various methods to inspect objects and arrays, and provide clear examples to enhance your understanding. Whether you’re debugging a complex application or just curious about how to better visualize your data, this guide will equip you with the knowledge you need to effectively analyze your JavaScript variables.
Understanding var_dump() in PHP
Before we dive into JavaScript, let’s briefly recap what var_dump()
does in PHP. This function displays structured information about one or more variables, including their type and value. It’s particularly useful for debugging because it reveals the inner workings of arrays and objects.
Now, let’s look at how we can achieve similar functionality in JavaScript.
Using console.log() for Debugging
One of the most straightforward ways to inspect variables in JavaScript is by using console.log()
. This method prints the value of a variable to the console, making it easy to see what’s happening in your code.
let user = {
name: "John Doe",
age: 30,
hobbies: ["reading", "gaming", "traveling"]
};
console.log(user);
Output:
{ name: "John Doe", age: 30, hobbies: ["reading", "gaming", "traveling"] }
The console.log()
function outputs the entire user
object to the console. You can expand this object in the console to view its properties and values in a more structured way. This method is particularly useful when you want to see the state of an object or array at a specific point in your code.
However, while console.log()
is great for basic logging, it doesn’t provide as detailed a structure as var_dump()
. For larger or more complex objects, it can be challenging to interpret the output directly from the console.
Using console.dir() for Better Structure
If you need a more structured view of your objects, console.dir()
is a better option. This method displays an interactive list of the properties of the specified JavaScript object, which is especially useful for exploring complex objects.
let product = {
id: 101,
name: "Laptop",
specifications: {
processor: "Intel i7",
ram: "16GB",
storage: "512GB SSD"
}
};
console.dir(product);
Output:
{ id: 101, name: "Laptop", specifications: { processor: "Intel i7", ram: "16GB", storage: "512GB SSD" } }
When you use console.dir()
, the output is more structured, allowing you to expand nested objects and view their properties easily. This is particularly beneficial when dealing with deep object structures, as it provides a clearer representation than console.log()
.
In addition, console.dir()
can take a second parameter that allows you to customize the depth of the output, giving you control over how much detail you want to see.
Using JSON.stringify() for String Representation
Another method to inspect JavaScript objects is by converting them to a string format using JSON.stringify()
. This approach is particularly useful when you want to log objects in a more readable format.
let settings = {
theme: "dark",
notifications: true,
language: "en"
};
console.log(JSON.stringify(settings, null, 2));
Output:
{
"theme": "dark",
"notifications": true,
"language": "en"
}
In this example, JSON.stringify()
takes the settings
object and converts it into a JSON string. The second argument, null
, is for a replacer function (which we don’t need here), and the third argument, 2
, specifies the number of spaces to use for indentation. This makes the output much more readable, especially for larger objects.
However, keep in mind that JSON.stringify()
will only serialize properties that are serializable. Functions and undefined values will be omitted from the output, so it’s not a complete equivalent to var_dump()
, but it can be very useful for logging purposes.
Conclusion
In conclusion, while PHP’s var_dump()
function is a powerful tool for inspecting variables, JavaScript offers several alternatives that can help you achieve similar results. Whether you choose console.log()
, console.dir()
, or JSON.stringify()
, each method has its own strengths and can be used effectively based on your specific debugging needs. By understanding these tools, you can enhance your debugging skills and gain better insights into your JavaScript applications.
As you continue your journey in web development, remember that effective debugging is key to building robust applications. So, experiment with these methods and find the one that works best for you!
FAQ
-
What is the main purpose of var_dump() in PHP?
var_dump() is used to display structured information about variables, including their type and value. -
Can I use console.log() to inspect nested objects in JavaScript?
Yes, console.log() allows you to inspect nested objects, but the output may not be as structured as with other methods. -
What is the difference between console.log() and console.dir()?
console.log() outputs a variable’s value, while console.dir() provides a more structured view of an object’s properties. -
How can I make JSON.stringify() output more readable?
You can pass a third argument to JSON.stringify() to specify indentation, making the output more human-readable. -
Are there any limitations to using JSON.stringify()?
Yes, JSON.stringify() does not serialize functions or undefined values, so some object properties may be omitted.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn