How to Switch Multiple Cases in Javascript
-
Switch
With Multiple Cases in Javascript Using Fall Through Model -
Handling Multiple Cases in
Switch
Using Mathematical Expressions in JavaScript -
Handling Multiple Cases in
Switch
Using String Expressions in Javascript -
Handling Multiple Cases in
Switch
Using Logical Expressions in Javascript
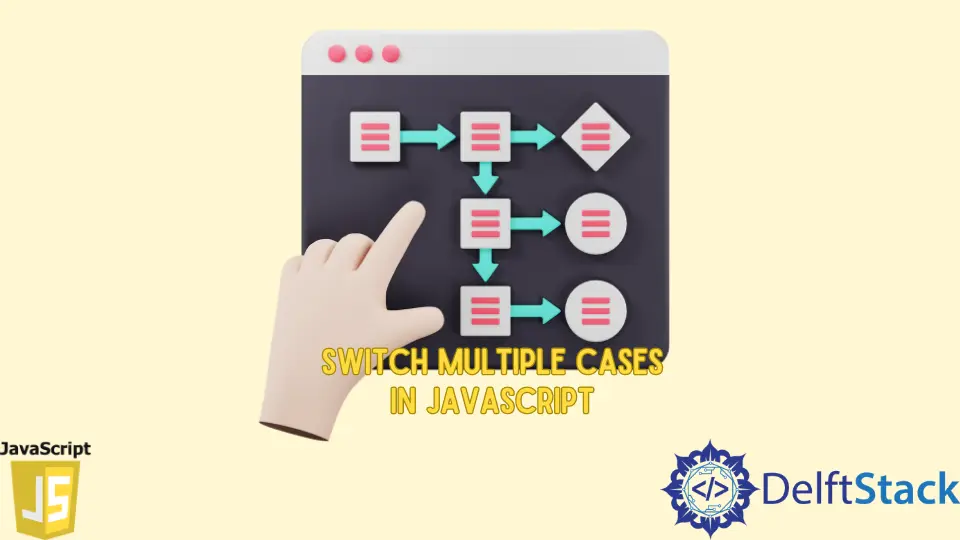
Switch cases are much performant than the if-else
ladder. They have a direct map of values from a variable, in the switch(variable)
block, to their corresponding values, in the case: value
block. We are familiar with the usual switch-case
usage with a single value map, but can it support multiple cases? Let us look at a few ways, in javascript, in which we can have multiple cases in the switch-case
block.
Switch
With Multiple Cases in Javascript Using Fall Through Model
Fall through
model is a first step in supporting switch
with more than one case
block in javascript. Here, we capture multiple values of the switched variable through multiple cases. We apply the break
keyword for a set of valid cases. For instance, if we want to log the quarter name of a year depending on the month passed, we will club the month indices based on the quarter. Refer to the following code.
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
}
function findQuarter(dateString) {
var monthIndex = new Date(dateString).getMonth();
switch (monthIndex) {
case 0:
case 1:
case 2:
console.log('First Quarter');
break;
case 3:
case 4:
case 5:
console.log('Second Quarter');
break;
case 6:
case 7:
case 8:
console.log('Third Quarter');
break;
case 9:
case 10:
case 11:
console.log('Fourth Quarter');
break;
}
}
Output:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
The findQuarter()
function accepts a date string in format yyyy-mm-dd
. It then extracts the month index from the dateString
argument by first converting it into a javascript date object and then getting the month index from the date object. The getMonth()
is a javascript function that applies on a Date
object and returns the month index starting from 0
. We use multiple cases here, stacked one below the other to capture multiple values for the output.
For instance, for months from January through March, the respective month indices will range from 0 to 2 based on output returned by the getMonth()
function. We stack all the cases from 0
to 2
without the break
keyword. Hence, they stumble and will break out off the switch-case
on meeting the break
that happens to be in the case 2:
line where it logs the value First Quarter
. This set of codes seems bulky. We can rewrite the same as follows to reduce the number of lines.
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
}
function findQuarter(dateString) {
var monthIndex = new Date(dateString).getMonth();
switch (monthIndex) {
case 0:
case 1:
case 2:
console.log('First Quarter');
break;
case 3:
case 4:
case 5:
console.log('Second Quarter');
break;
case 6:
case 7:
case 8:
console.log('Third Quarter');
break;
case 9:
case 10:
case 11:
console.log('Fourth Quarter');
break;
}
}
Output:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
Note that here the output remains the same. It is just another way of writing the Fall through
switch-case
model. It makes the code now look cleaner and saves space.
Handling Multiple Cases in Switch
Using Mathematical Expressions in JavaScript
We are dealing with numerical values of a month as per the example mentioned in the earlier section. Can we use expressions in case
blocks? Definitely yes! Let us refer to the following code before we explain the functioning.
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
findQuarter('2021-2-30');
}
function findQuarter(dateString) {
var monthIndex = new Date(dateString).getMonth();
switch (monthIndex) {
case ( (monthIndex < 2) ? monthIndex : -1 ):
console.log('First Quarter');
break;
case ( ((monthIndex > 2) && (monthIndex < 5)) ? monthIndex : -1):
console.log('Second Quarter');
break;
case ( ((monthIndex > 5) && (monthIndex < 8)) ? monthIndex : -1):
console.log('Third Quarter');
break;
case ( ((monthIndex > 8) && (monthIndex < 11)) ? monthIndex : -1):
console.log('Fourth Quarter');
break;
default:
console.log('Invalid date');
break;
}
}
Output:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
If we use expressions in the switch case, they must return a numeric value else -1
. In the above code snippet, we have added numerical expressions in the case
block instead of their actual values. These expressions evaluate for the corresponding quarter of the month and log the quarter name. Here, we have included the default
clause in the switch case
for handling Invalid date
. The getMonth()
method in javascript returns a value (between 0
and 11
) if the month
in date
is valid (within range of 1
to 12
) else it will return NaN
. The default
block will catch such invalid date scenarios.
Handling Multiple Cases in Switch
Using String Expressions in Javascript
We can also use string expressions inside the case
block. These expressions evaluate at run time, executing the case
clause. For our month example, let us try to switch the month name. For this, we will be applying the toLocaleDateString()
function on the date object. In the options parameter of the function, we give { month: 'long' }
. The output for the toLocaleDateString('en-US', { month: 'long' })
will now be a month name, the full month name. Then we switch the full month name output from the toLocaleDateString('en-US', { month: 'long' })
function.
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
findQuarter('2021-21-30');
}
function findQuarter(dateString) {
var monthName =
new Date(dateString).toLocaleDateString('en-US', {month: 'long'});
switch (true) {
case['January', 'February', 'March'].includes(monthName):
console.log('First Quarter');
break;
case['April', 'May', 'June'].includes(monthName):
console.log('Second Quarter');
break;
case['July', 'August', 'September'].includes(monthName):
console.log('Third Quarter');
break;
case['October', 'November', 'December'].includes(monthName):
console.log('Fourth Quarter');
break;
default:
console.log('Invalid date');
break;
}
}
Output:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
Invalid date
In the above code, we have used the switch(true)
statement. In the case
clause, we have the string comparison. We created a temporary array of months for each quarter and added them to each case
. Then we check if the monthName
is available in the quarter array. If found, it will log the name of the quarter. If the date is invalid, the toLocaleDateString('en-US', { month: 'long' })
function will output Invalid Date
. This scenario is caught by the default
block as the Invalid Date
string will not match the predefined arrays holding the names of the months in a quarter. Hence, we handle the invalid date scenario smoothly here.
.indexOf()
instead of .includes()
or find()
functions to support multiple browsers. The .includes()
and find()
function will not be supported in Internet Explorer.Handling Multiple Cases in Switch
Using Logical Expressions in Javascript
As we saw in the mathematical and the string expressions, we can also use logical operators in multiple cases
in the switch-case
block. In the month example, we will use the logical OR
operator ||
for deciding if the month name is in a particular quarter. Let us refer to the following code.
window.onload =
function() {
findQuarter('2021-08-08');
findQuarter('2021-05-12');
findQuarter('2021-02-28');
findQuarter('2021-11-30');
findQuarter('2021-21-30');
}
function findQuarter(dateString) {
var monthName =
new Date(dateString).toLocaleDateString('en-US', {month: 'long'});
switch (true) {
case (
monthName === 'January' || monthName === 'February' ||
monthName === 'March'):
console.log('First Quarter');
break;
case (monthName === 'April' || monthName === 'May' || monthName === 'June'):
console.log('Second Quarter');
break;
case (
monthName === 'July' || monthName === 'August' ||
monthName === 'September'):
console.log('Third Quarter');
break;
case (
monthName === 'October' || monthName === 'November' ||
monthName === 'December'):
console.log('Fourth Quarter');
break;
default:
console.log('Invalid date');
break;
}
}
Output:
Third Quarter
Second Quarter
First Quarter
Fourth Quarter
Invalid date
In the code, we check if the monthName
value equals the month of a quarter. Accordingly, we console the name of the quarter. Here too, we handle the case of invalid date as it will not satisfy any of the conditions coded in the case
clause and will fall into the default
block, which then prints Invalid date
to the browser console.