How to Set onClick With JavaScript
- What Is an Event and EventListener in JavaScript
-
How to Add an
onClick
EventListener to an Element in JavaScript -
How to Pass a Reference of an Element to an
onClick
EventListener in JavaScript -
How to Add
onClick
EventListener to Jquery in JavaScript
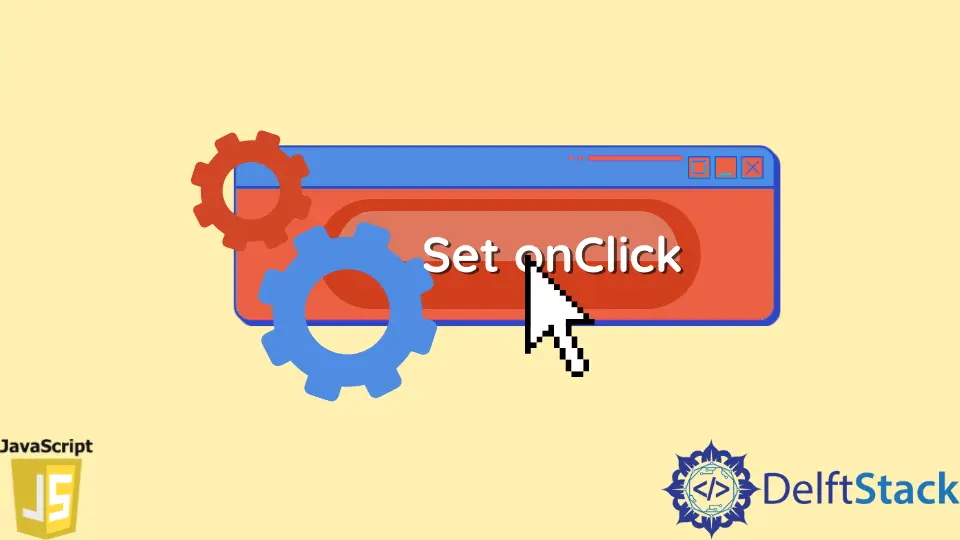
In this tutorial, we will discuss how to attach an onClick
listener to an element using JavaScript; we will discuss:
- What is an Event and EventListener or EventHandler in JavaScript
- How to add an
onClick
EventListener to an element - How to pass a reference to an
onClick
EventListener - How to add
onClick
EventListener using Jquery
What Is an Event and EventListener in JavaScript
An event is something that a browser or an end-user does. These events can be handled by a JavaScript concept called Event Handlers or Event Listeners. An Event Listener is executed when a particular event happens.
onClick
is one of these Event Listeners. An onClick
event listener triggers/executes when a user clicks on an element.
How to Add an onClick
EventListener to an Element in JavaScript
<!DOCTYPE html>
<html>
<body>
<div id="textElement" onclick="ChangeColor()">
Change Color to Red
</div>
<script>
function ChangeColor() {
document.getElementById("textElement").style.color = "red";
}
</script>
</body>
</html>
The above code would trigger/execute the ChangeColor
method, which will access the element, using the Document object model, having an id of textElement
and changing its text color to red.
Notice that the onClick
is set as a property of an element in HTML similar to class
, id
, etc.
Alternatively, you can set the attribute onClick
of an element directly using JavaScript
.
<!DOCTYPE html>
<html>
<body>
<div id="textElement">
Change Color to Red
</div>
<script>
document.getElementById("textElement").onclick = function() {
document.getElementById("textElement").style.color = "red";
};
</script>
</body>
</html>
This will access the textElement
and assign it a function
; this function
will execute when the user clicks the HTML element, the result will be similar. For example, the element’s color having an id
of textElement
would be changed to red.
Furthermore, you can use the addEventListener
method/function of the JavaScript element to perform the same functionality.
<!DOCTYPE html>
<html>
<body>
<div id="textElement">
Change Color to Red
</div>
<script>
let element = document.getElementById("textElement");
element.addEventListener("click", function(){
element.style.color = "red";
});
</script>
</body>
</html>
The addEventListener
takes two arguments; the first is the type of event to listen to on an HTML element, and the second is the callback function that would execute when a certain event occurs.
If you are using Internet Explorer, the addEventListener
will not work because of compatibility issues. We would have to use a fall-back using attachEvent
to replicate this behavior.
<!DOCTYPE html>
<html>
<body>
<div id="textElement">
Change Color to Red
</div>
<script>
let element = document.getElementById("textElement");
if(element.addEventListener){
element.addEventListener('click', function(){
element.style.color = "red";
});
}else if(element.attachEvent){
element.attachEvent('onclick', function(){
element.style.color = "red";
});
}
</script>
</body>
</html>
How to Pass a Reference of an Element to an onClick
EventListener in JavaScript
<!DOCTYPE html>
<html>
<body>
<div id="textElement" onclick="ChangeColor(this,'red')">
Change Color to passed Color
</div>
<script>
function ChangeColor(element,color) {
element.style.color = color;
}
</script>
</body>
</html>
In the example, we pass two arguments, this
and red
, this
passes a reference to a DOM element to the function, whereas red
is just a string.
You can notice that by just changing the second argument, we can change the element’s color. This approach increases reusability compared to the other approach. For example, we can use the ChangeColor
method with multiple elements.
<!DOCTYPE html>
<html>
<body>
<div id="textElement" onclick="ChangeColor(this,'red')">
Change Color to Red
</div>
<div id="textElement" onclick="ChangeColor(this,'blue')">
Change Color to Blue
</div>
<script>
function ChangeColor(element,color) {
element.style.color = color;
}
</script>
</body>
</html>
How to Add onClick
EventListener to Jquery in JavaScript
If you are not using Vanilla JavaScript but are using something like Jquery, a JavaScript library, the process is similar; the only difference is the Syntax.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$("#textElement").click(function(){
$(this).css('color','red')
});
});
</script>
</head>
<body>
<div id="textElement">Change Color to Red</p>
</body>
</html>
It will add an event listener to the element having an id
of textElement
as soon as the document loads.
Related Article - JavaScript EventListener
- How to Change Input Value in JavaScript
- How to addEventListener vs. Onclick in JavaScript
- How to Check Whether a Button Is Clicked by JavaScript