How to Open URL in the Same Window or Tab in JavaScript
-
Use
window.open()
Method to Open URL in the Same Window or Tab in JavaScript -
Use
location.replace()
Method to Open URL in the Same Window or Tab in JavaScript
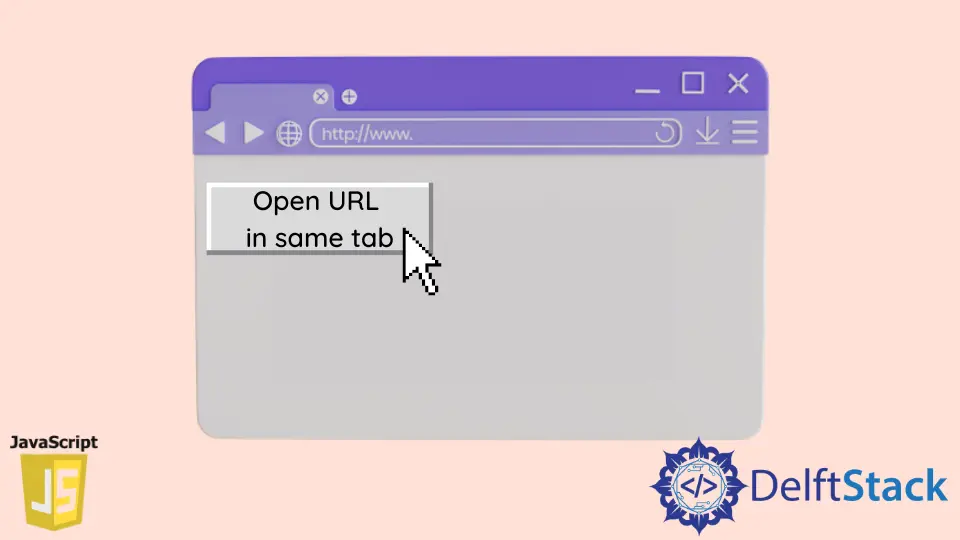
We’ll look at how to open URLs in the same window or tab in this article.
Use window.open()
Method to Open URL in the Same Window or Tab in JavaScript
The window.open()
method is a JavaScript pre-defined window technique for opening a new tab or window in the same window and tab in the browser.
A new window or tab will open depending on your browser settings or parameters supplied in the window.open()
method.
Syntax:
window.open(url, '_self');
The _self
value where the URL will replace the previous output and a new window will appear in the same frame if this option is passed.
Example:
Save the code with the .html
extension and run the following script.
<html>
<body>
<form id="formA" runat="server">
<div style="text-align:center; font: size 25px;">
<label>Welcome to same page redirect</label><br><br>
<input type="button" value="Google.Com" class="btn" id="btnn">
</div>
</form>
<script type="text/javascript">
document.getElementById("btnn").addEventListener("click", (e) =>{
let url ="https://www.google.com/";
window.open(url, "_self");
})
</script
</body>
</html>
Output:
Before clicking the button:
After clicking the button, it will open a new URL in the same tab in the same window.
Use location.replace()
Method to Open URL in the Same Window or Tab in JavaScript
The replace()
method replaces the current page with another page in JavaScript. The replace()
method changes the current window’s URL to the URL specified in the replace()
method.
Syntax:
location.replace(URL);
This function only accepts a single URL argument. The URL links to another page that must be replaced with an earlier version.
This method returns or opens a new window with a new page URL in the same frame.
Example:
<!DOCTYPE html>
<html>
<body>
<h1>Welcome to same page redirect to different url</h1>
<h2>The replace Property</h2>
<button onclick="Function()">Replace document</button>
<script>
function Function() {
location.replace("https://www.google.com")
}
</script>
</body>
</html>
Before clicking the button:
After clicking the button:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn