Regex Variable in JavaScript
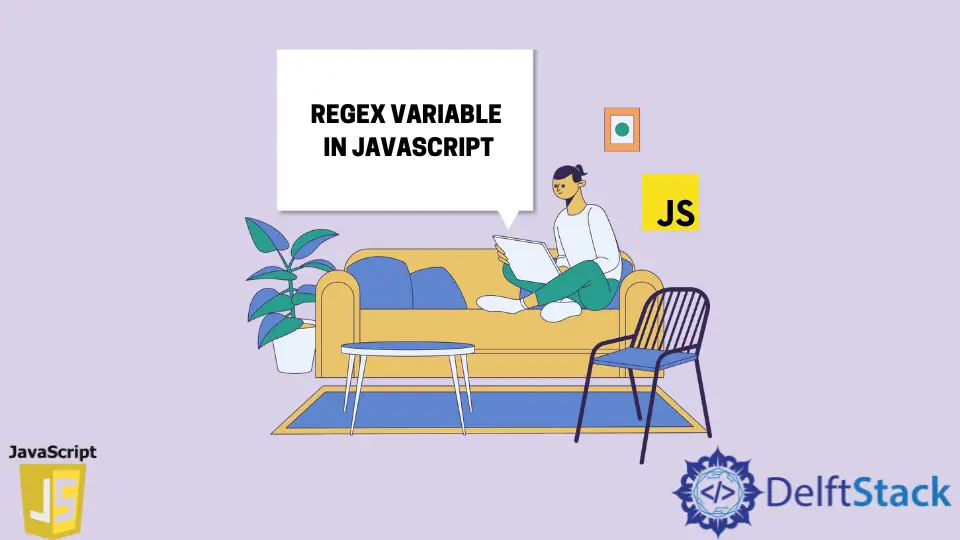
A regular expression, also known as regex, is a sequence of characters that defines a pattern of text to be matched. A regular expression is an object used only with strings.
To learn more about the regular expressions and the various character classes and flags available, you can check the official documentation. This article shortly discusses the regular expression in JavaScript.
Use a Variable in a Regular Expression Using Template Literals in JavaScript
There are two ways to define a regular expression in JavaScript, one by using the regular expression literal where patterns are enclosed inside slashes /
and the other by calling the constructor function of the RegExp
object. This constructor function takes two parameters, the first is the pattern
and the second is the flag
.
For this tutorial, we will use the constructor function of the RegExp
object and will see how to use a variable within a regular expression in JavaScript. In the below example, we have taken an inputString
that contacts various characters and numbers.
Our aim here is to create a pattern, pass it to the RegExp
constructor function, and replace all the digits within the string with a word.
let inputString = "I work at 1. Android is developed by 2.";
Since the RegExp
constructor function takes the pattern as the first parameter, let’s create a new variable called pattern
. We will pass the \\d
that will search for the digits inside the string inside this variable.
We will enclose the pattern
variable with the \\b
character and pass it as the first argument to the RegExp
constructor function. But just passing the pattern
variable as \\bpattern\\b
will not work, throwing an invalid escape sequence
error.
We have to use the template literals or template strings for this to work. We will enclose the entire string inside the backticks characters, and then wherever we want to add the pattern
variable, we will use the ${}
and pass the pattern
variable inside the curly braces as shown in the below code snippet.
Code Snippet - JavaScript:
let pattern = "\\d";
let re = new RegExp(`\\b${pattern}\\b`, 'gi');
console.log(inputString.replace(re, "Google"));
And then, we will pass gi
as the second argument to the RegExp
constructor function. The g
will perform a global search on the entire input string, and i
will replace all the instances of a specific word provided inside the replace
function.
Then we will store the result of the RegExp
constructor function inside the re
(regular expression) variable. Finally, we will use the string replace()
function on the inputString
and pass the regex as the first parameter and the new word replaced with the matched.
Output:
I work at Google. Android is developed by Google.
In this case, we want to replace the numbers 1
and 2
with the word Google
. So, the regular expression stored inside the re
variable will find these two digits within the inputString
, and then it will replace these numbers with the word Google
with the help of the replace()
function.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn