How to Validate Email With Regex in JavaScript
- Check Email Between Two and Three Letter TLD and Disallow Characters in Email and Domain Names in JavaScript
- Check Email Between Two and Six TLD Names and Disallow the Plus Sign in Email Names in JavaScript
- Check Email and Allow Numbers and Valid Characters in Email and Domain Name in JavaScript
- Check Email Address With RFC 5322 Format in JavaScript
- Conclusion
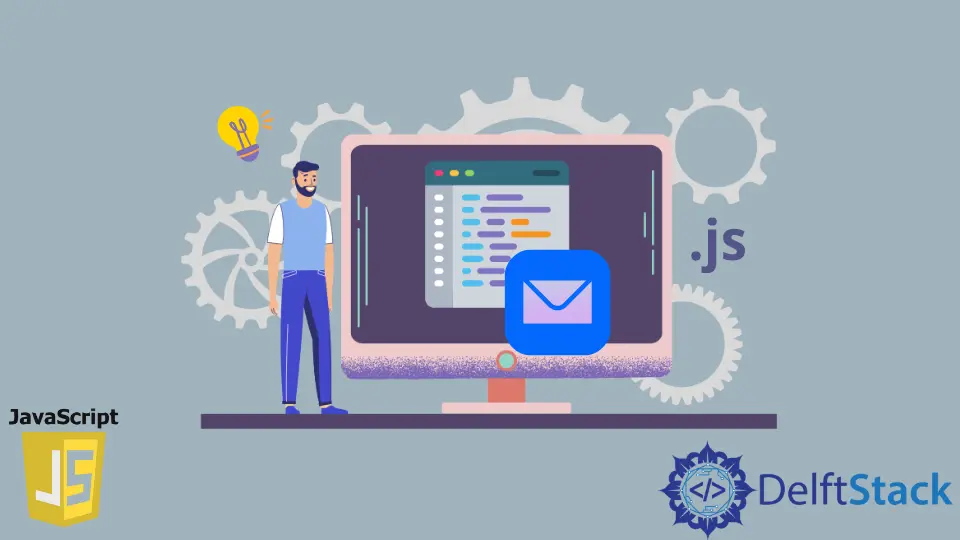
This article will teach you multiple regular expressions that can validate different types of email addresses. Therefore, you can modify or select one of the patterns that best suits your use case.
Check Email Between Two and Three Letter TLD and Disallow Characters in Email and Domain Names in JavaScript
You can write a regular expression that allows email addresses between two and three TLDs. For example, .com
and .io
.
This also means the pattern will not allow an email with the following characteristics.
- A TLD longer than three characters TLD, e.g.,
.mobi
- Multiple TLD, e.g. (
.gmail.sa
) - Domain with numbers, e.g.,
tech125.com
- The full stop (
.
) or a hyphen (-
) in the email name - The hyphen in the domain name
In our example code below, we have a regular expression that’ll return true
for email addresses like abc@gmail.com
, hello@hello.com
, and ab0c@gmail.com
.
let pattern = /^\w+@[a-zA-Z_]+?\.[a-zA-Z]{2,3}$/;
function checkEmailAddress(email_address) {
let result = pattern.test(email_address);
console.log(result);
}
checkEmailAddress('abc@gmail.com')
Output:
true
Meanwhile, the pattern will return false
for the following email address.
ab0c@my-domain.com
Check Email Between Two and Six TLD Names and Disallow the Plus Sign in Email Names in JavaScript
A regular expression pattern can permit the full stop sign (.
) and disallow the plus sign (+
) in an email address. This means email addresses with the plus sign will not pass the regular expression.
So, they’ll return false
, even if it’s valid. The pattern we’ll define in this section will return false
for an email with any following characteristics.
- An email with the plus sign (
+
) - A TLD with more than six characters, e.g. (
.technology
)
In the following code, we have a new regular expression. Meanwhile, this pattern returns true
for the last email in the previous section.
let pattern = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6}$/;
function checkEmailAddress(email_address) {
let result = pattern.test(email_address);
console.log(result);
}
checkEmailAddress('ab0c@my-domain.com')
Output:
true
Meanwhile, the below-listed email addresses will not pass this pattern. However, they are all valid for some email providers.
ab+c@my-domain.com
mr+john@my-domain.com.ga
Check Email and Allow Numbers and Valid Characters in Email and Domain Name in JavaScript
You can write a flexible, regular expression pattern that permits most of the valid mail addresses that exist today. Such an email will have the following characteristics.
- Some characters like the full stop and sign in the email and TLD name.
- The new TLD like
.technology
and.business
.
The downside of this pattern is that it’ll allow multiple of these characters. As a result, the user can pass an extremely long email address that appears valid, but it’s not.
let pattern =
/^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
function checkEmailAddress(email_address) {
let result = pattern.test(email_address);
console.log(result);
}
checkEmailAddress('johndoe@citadel-consulting.com');
Output:
true
However, the following invalid email address will pass this pattern.
aaa++++++++++@gmail.technologyyyyyyyyyyyyyyyyyyyyyyyyyyy.com
Check Email Address With RFC 5322 Format in JavaScript
You can write a regular expression pattern compliant with the RFC 5322 format. However, such a regular expression will have a long length.
As a result, it can be incomprehensible. So, you can use the shorthand version with the RegExp
class in JavaScript.
In the following code, we’ve implemented a regular expression pattern compliant with RFC 5322. So, it’ll return true
for the most valid email addresses.
let pattern = new RegExp(
'([!#-\'*+/-9=?A-Z^-~-]+(\.[!#-\'*+/-9=?A-Z^-~-]+)*|"\(\[\]!#-[^-~ \t]|(\\[\t -~]))+")@([!#-\'*+/-9=?A-Z^-~-]+(\.[!#-\'*+/-9=?A-Z^-~-]+)*|\[[\t -Z^-~]*])');
function checkEmailAddress(email_address) {
let result = pattern.test(email_address);
console.log(result);
}
checkEmailAddress('aa@gmail.com.sa');
Output:
true
Conclusion
This article discussed different regular expression patterns that validate an email address. However, it’s all on the client-side.
We implore you to also validate the email on the server-side for added security.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn