JavaScript RegEx Group
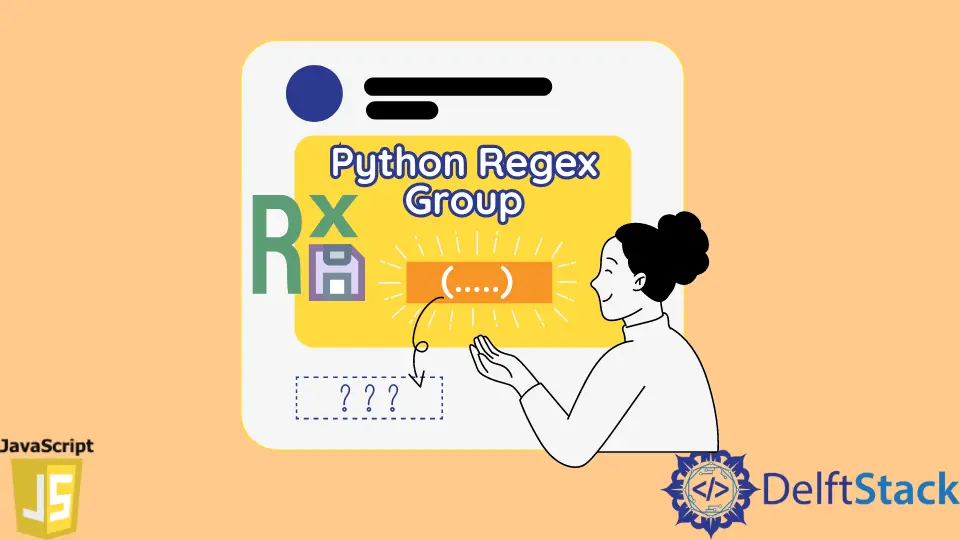
Regular expressions are patterns that match combinations of characters or words from a given string. Regular expressions are also objects in JavaScript.
These patterns are defined with test()
and exec()
of RegExp and with the methods matchAll()
, match()
, replaceAll()
, replace()
, search()
and split()
of String.
Today’s post will learn about the regex groups in JavaScript.
RegEx Group in JavaScript
Part of a pattern can be enclosed in brackets (...)
. This is referred to as a capture group
.
This has two effects:
- You can keep part of the match as a separate element in the result array.
- If we put a quantifier after the brackets, it applies to the brackets as a whole.
Syntax:
const re = /ab+c/;
const re = new RegExp('ab+c');
The above syntax shows the normal regular expression pattern. There are two ways of lookahead assertions: positive
and negative
.
The syntax for a positive lookahead is (?=...
). The construction for a negative lookahead is (?!...)
. A forward negative asserts that a pattern does not follow any particular pattern.
ES2018 complements lookahead assertions by integrating lookahead assertions into JavaScript. Marked with (?<=...)
, a backspace assertion allows you to match a pattern only if another pattern precedes it.
You can find more information about groups and ranges in the documentation for groups and ranges
.
Name Capture Groups
You can group part of a regular expression by enclosing the characters in parentheses. This allows you to limit the alternation to just part of the pattern or apply a quantizer to the entire group.
Also, you can extract the matching value in parentheses for further processing.
The following code gives an example of how to find a year, month and date in a string:
const regEx = /(\d{4})-(\d{2})-(\d{2})/;
const matchedPattern = regEx.exec('2022-04-22');
console.log(matchedPattern[0]);
console.log(matchedPattern[1]);
console.log(matchedPattern[2]);
console.log(matchedPattern[3]);
In the above example, we defined the regex for a year, month and day. Finally, when we run the regex against the specified string, it captures the pattern and groups it with the matching group.
We must remember that the pattern is for a year, month, and day. After the entire regex is executed, it prints something like the below result:
Output:
"2022-04-22"
"2022"
"04"
"22"
The solution to above problem is capturing groups. The most advanced version is capturing groups using a more expressive syntax in the form of (?<name>...)
:
const regEx = /(?<year>\d{4})-(?<month>\d{2})-(?<day>\d{2})/;
const matchedPattern = regEx.exec('2022-03-22');
console.log(matchedPattern.groups);
console.log(matchedPattern.groups.year);
console.log(matchedPattern.groups.month);
console.log(matchedPattern.groups.day);
In the above example, we defined the regex for a year and named its year. Similarly, we have defined the regex for month and day.
Finally, when we run the regex against the specified string, it captures the pattern and groups it with the matching group. After the entire regex is executed, it prints something like the below result:
Output:
{
day: "22",
month: "03",
year: "2022"
}
"2022"
"03"
"22"
You can also access the complete code here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn