How to Redirect to a Webpage in JavaScript
-
JavaScript Redirect a WebPage With
location.href()
-
JavaScript Redirect a WebPage With
location.replace()
-
3. JavaScript Redirect a WebPage With
location.assign()
- 4. JavaScript Redirect a WebPage by Creating an Anchor Element Dynamically
- Conclusion
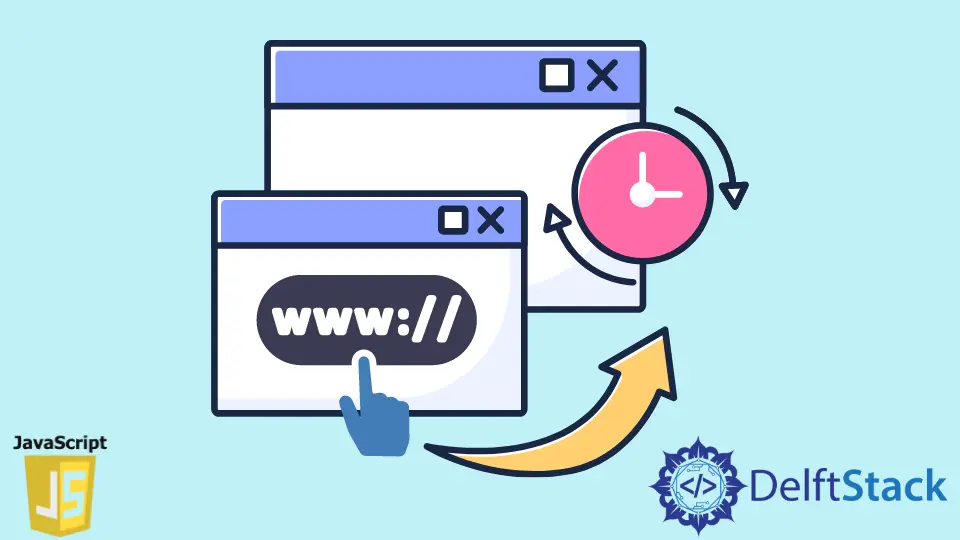
There are various ways in JavaScript to re-route the user. It depends upon the business requirement on what sort of redirect behavior the site should have. You can redirect your users in the following ways:
location.href()
location.replace()
location.assign()
- Create anchor element dynamically
JavaScript Redirect a WebPage With location.href()
Use the location
interface in conjunction with Document
and Window
objects for redirecting. Typically the window.location.href
returns the URL of the current page. For instance, if you run the following code, you will see the page URL:
console.log(window.location.href)
Output:
"https://www.delftstack.com/"
The trick is to replace this URL by assigning a different URL to the window.location.href
. It will make the browser load the page specified by the URL, hence redirecting to it. In terms of the site history stack, this method changes the current reference URL. The following code will navigate to the how-to page of DelfStack
.
window.location.href = 'https://www.delftstack.com/howto/';
Remarks:
- Once the new URL is loaded, the older web page is accessible by the browser back button.
- It is the most commonly used method for redirection
JavaScript Redirect a WebPage With location.replace()
If you wish to permanently move to a webpage, use the location.replace
. The difference is that location.replace
will replace the current URL with a new URL. Hence, the user will not be able to go back to the previous URL. In terms of the browser history stack, the method pops the last web page URL and pushes the URL in the value.
window.location.replace('https://www.delftstack.com');
Executing this will load the https://www.delftstack.com
site.
Remarks:
- We recommend using this method only when necessary.
- One cannot go back to the previous link using this method. Hence, it may not be a good User Experience.
3. JavaScript Redirect a WebPage With location.assign()
Like the location.replace()
, the assign()
method comes with a difference that the current link remains in the browser history. Hence, the user will be able to go back to the previous page using the browser back button. This method also takes the target URL as the parameter.
window.location.assign('https://www.delftstack.com');
4. JavaScript Redirect a WebPage by Creating an Anchor Element Dynamically
In older browsers, especially Internet Explorer, having version 8 or lower, the location interface is not supported. Hence, we create an anchor tag (<a>
) dynamically and set the href
attribute with the target URL. As mentioned earlier, the anchor tag is a passive element that requires user interaction to invoke it. So, a click event is triggered in the code to get the redirection to work.
let targetURL = 'https://www.delftstack.com';
let newURL = document.createElement('a');
newURL.href = targetURL;
document.body.appendChild(newURL);
newURL.click();
Here, we achieve the redirect by:
- Creating an anchor tag element
document.createElement('a');
- Setting
href
property with thenew URL newURL.href = targetURL
- Attaching the dynamically created tag to the DOM node with
document.body.appendChild(newURL)
- Finally, invoking it by emulating a user click
newURL.click()
The browser will load the new URL.
Conclusion
Depending on business requirements, it will be good to use the meta refresh method to redirect a user as soon as he lands on the under-maintenance site web page. Using anchor tag is quite common if navigation is intended based on user click. We can use methods of the Location interface in JavaScript, the window.location.href
and window.location.assign()
, to programmatically send a user to a new URL.