How to Redirect Page With Onclick in JavaScript
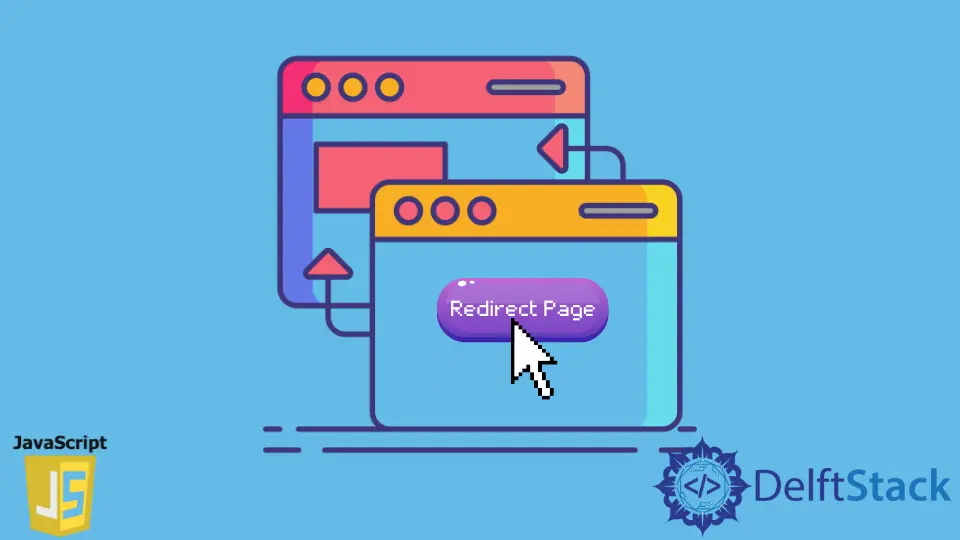
This tutorial will teach you how to create a JavaScript redirect when a user clicks on an HTML button.
We’ll use the onclick
event to listen to click events on the button. If the user clicks the button, we’ll perform the redirect.
Redirect to Another Page With onclick
Event in JavaScript
-
Initial Setup
We aim to explain how to do this on a website; however, you can try this out on a local development server. That said, download a server like Apache.
You can get Apache via the XAMPP installation. Once you have XAMPP installed, you should put all the code in this tutorial in the
htdocs
folder; you’ll find thehtdocs
folder in the directory of your XAMPP installation.Preferably, you can create a folder in
htdocs
namedjsredirect
. So thejsredirect
folder will contain your code. -
Create the HTML Form
We have two HTML codes below. The first is an HTML form with two form inputs and a button.
The button has a unique id that we’ll use in our JavaScript code. Meanwhile, the second HTML contains a text that reads
Redirected from a form
.The next code is the HTML form that we’ll redirect to. Save it as
home.html
with the necessary doctype for an HTML document.<body> <h1>Redirected from a form</h1> </body>
In the next code, we have the code for the HTML form. Save it as
form.html
.
```html
<main>
<form>
<div class="form-row">
<label for="first-name">First name</label>
<input id="first-name" type="text" name="first-name" required>
</div>
<div class="form-row">
<label for="last-name">Last name</label>
<input id="last-name" type="text" name="last-name" required>
</div>
<div class="form-row">
<button id="submit-form" type="submit">Submit</button>
</div>
</form>
</main>
```
-
Create the JavaScript Code
The JavaScript code below performs the redirect if the user clicks on the button in our form.
First, we get the button by its id using the
document.getElementById
method. Afterward, we attach an event listener to the button.In the redirect function, we use the
location.href
method to describe the location of the redirect. In this case, we set it to our second HTML code saved ashome.html
.Therefore, the redirect URL is
http://localhost/jsredirect/home.html
, wherejsredirect
is the name of our project folder.If you are on a live server, replace localhost with your website’s name.
```javascript
let button = document.getElementById('submit-form');
button.onclick = function(e) {
e.preventDefault();
// Replace localhost and the folder name
// based on your setup
location.href = 'http://localhost/jsredirect/home.html';
}
```
-
Testing Our Code
Now that we have the HTML files and JavaScript set up, we can test if it works. Launch your local server and navigate to your project folder via
localhost/<your_project_folder>
.Once it loads, if you’d like, you can fill out the form with arbitrary data. Also, you can implement logic that ensures your user fills the form.
However, the important thing is to ensure that the redirect works when clicking on the button. So, go ahead and click the button.
Your web browser should redirect to the second HTML file if you’ve done everything right. We show an example of the redirect in the following GIF image.
-
The CSS Code
The next CSS code block styles the form.
```css
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
main {
margin: 2em auto;
width: 50%;
outline: 1px solid #1a1a1a;
}
.form-row {
padding: 0.5em;
display: flex;
justify-content: space-around;
}
.form-row input {
width: 45%;
padding: 0.2em;
}
.form-row button {
padding: 0.5em;
}
```
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn