JavaScript DOM Ready
- Understanding the DOM Ready Concept
- Using Vanilla JavaScript
- Using jQuery
- Using the window Load Event
- Conclusion
- FAQ
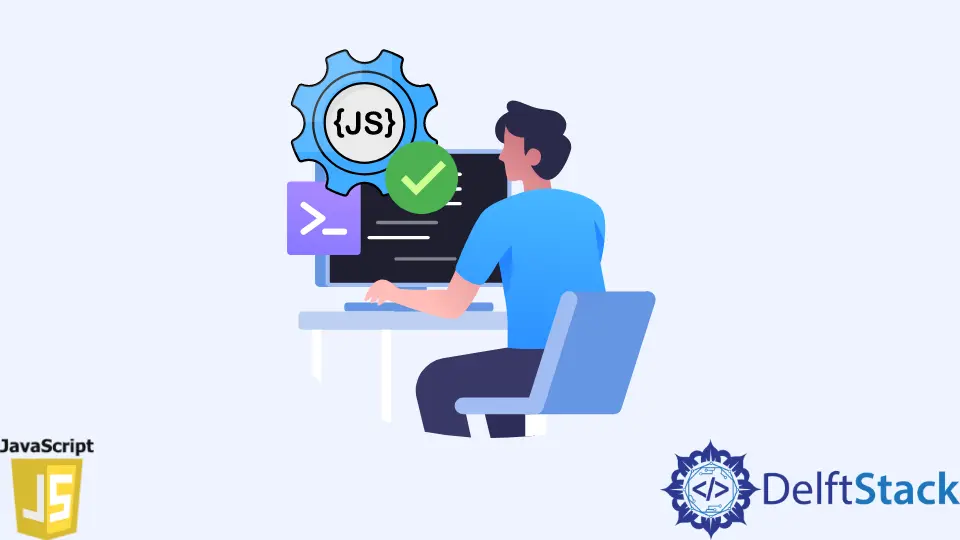
When developing web applications, ensuring that your scripts run at the right time is crucial for a smooth user experience. One common challenge developers face is executing JavaScript functions only after the Document Object Model (DOM) has fully loaded.
This tutorial will guide you through various methods to achieve this, ensuring that your code runs seamlessly without errors. By the end of this article, you’ll have a solid understanding of how to call a function when the DOM is ready, enhancing your web development skills.
Understanding the DOM Ready Concept
Before diving into the methods, it’s essential to grasp what “DOM Ready” means. The DOM is a representation of the HTML structure of your webpage. When we say the DOM is ready, we mean that the browser has finished loading the HTML and can now manipulate it using JavaScript. This is a critical moment because trying to access elements that haven’t been loaded yet will lead to errors.
In this article, we’ll explore different techniques to ensure your functions execute correctly once the DOM is ready. Let’s get started!
Using Vanilla JavaScript
One of the simplest ways to check if the DOM is ready is by using the DOMContentLoaded
event. This event fires when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading.
Here’s how you can implement this in your JavaScript code:
document.addEventListener("DOMContentLoaded", function() {
console.log("DOM is fully loaded and parsed");
});
Output:
DOM is fully loaded and parsed
In this code, we add an event listener to the document that listens for the DOMContentLoaded
event. Once the DOM is fully loaded, the function inside the event listener executes, logging a message to the console. This method is straightforward and works in all modern browsers.
Using jQuery
If you’re using jQuery, it provides a very convenient way to handle the DOM ready event. jQuery simplifies the process with its built-in function $(document).ready()
. This method ensures that your code runs only after the DOM is ready.
Here’s an example of how to use it:
$(document).ready(function() {
console.log("DOM is ready using jQuery");
});
Output:
DOM is ready using jQuery
In this snippet, we utilize jQuery’s ready()
function. The code inside this function will execute as soon as the DOM is ready, allowing you to manipulate elements safely. This method is particularly beneficial if you’re already using jQuery in your project, as it keeps your code clean and concise.
Using the window Load Event
Another approach to ensure that your JavaScript runs after the DOM is ready is to use the load
event on the window object. However, it’s important to note that this event waits for the entire page, including all dependent resources like images and stylesheets, to load.
Here’s how you can use the load
event:
window.onload = function() {
console.log("Window is fully loaded");
};
Output:
Window is fully loaded
In this example, we assign a function to window.onload
, which will execute once everything on the page has loaded. While this method works, it’s generally not recommended for DOM manipulation since it can delay the execution of your scripts unnecessarily. Use this approach if you need to ensure that all resources are fully loaded before running your code.
Conclusion
Understanding how to call a function when the DOM is ready is a fundamental skill for any web developer. Whether you choose to use vanilla JavaScript, jQuery, or the window load event, each method has its advantages. By mastering these techniques, you can ensure that your scripts run smoothly, providing a better experience for users. Remember to choose the method that best fits your project needs. Happy coding!
FAQ
-
what is the DOM?
The DOM (Document Object Model) is a programming interface for web documents. It represents the structure of a document as a tree of objects, allowing programming languages to manipulate the content, structure, and style of the document. -
why should I wait for the DOM to be ready?
Waiting for the DOM to be ready ensures that your JavaScript code interacts with the HTML elements only after they have been fully loaded. This prevents errors and ensures that your scripts function correctly. -
can I use multiple methods to check if the DOM is ready?
Yes, you can use multiple methods in your project. However, it’s best to stick to one method for consistency and to avoid confusion.
-
does the choice of method affect performance?
Generally, usingDOMContentLoaded
or jQuery’sready()
is more efficient than theload
event, as they trigger earlier in the loading process. -
is jQuery necessary for DOM manipulation?
No, jQuery is not necessary. You can achieve DOM manipulation using vanilla JavaScript, which is often more efficient for modern web development.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn