How to Move Mouse Pointer to a Specific Position Using JavaScript
- Create a Basic HTML Structure With Some CSS Styling
- Use JavaScript To Move Mouse Pointer to a Specific Position
- Conclusion
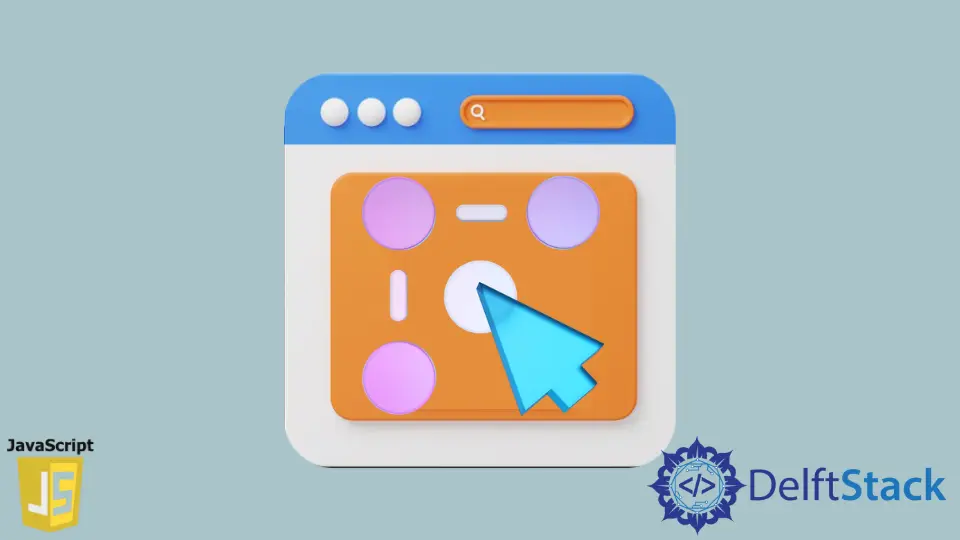
Note that there is no way to move the mouse pointer to a specific position in JavaScript. The primary reason is that it can create security issues for the users and hurt the user experience.
In this article, we will create a fake or a custom mouse pointer, which may look similar to the default system’s mouse pointer, and then we will move its position to a different position using JavaScript. This technique of moving a mouse pointer to a specific position is for demonstration purposes and should be completely avoided on production-ready websites.
Create a Basic HTML Structure With Some CSS Styling
We will create two buttons on the screen (one on the left and another on the right side of the screen), and our aim will be when we click on the first button, the mouse pointer will automatically be moved onto the second button on the screen.
First, we create two HTML elements inside our HTML, one img
tag and another the div
element. The img
tag will contain the image of the custom or the fake cursor that we will be used in place of the original system mouse cursor.
You can use any mouse cursor image you want from the internet. The div
will have two buttons, "button 1"
and "button 2"
inside it.
We will also give an id
to all these elements so that we can reference them inside JavaScript and style them inside CSS.
Code Snippet - HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Move mouse pointer</title>
<style>
* {
cursor: none;
}
.block{
display: flex;
justify-content: space-between;
}
img {
pointer-events: none;
position: absolute;
}
</style>
</head>
<body>
<img id="cursor" src="./mouse_cursor.png" width="16" height="22" />
<div class="block">
<button id="btn1">Button 1</button>
<button id="btn2">Button 2</button>
</div>
</body>
</html>
Inside the CSS, we hide the original system’s mouse pointer from the entire page with the help of the cursor: none
CSS property inside the asterisk (*), also known as the CSS universal selector. Then we will add a flexbox to the div
element so there can be a space between them.
On the img
tag, our custom cursor will add the pointer-events: none
property so that no pointer events can be applied to it. You can learn more about pointer-events on MDN, and we’ll make it absolute so we can move it anywhere on the screen.
Use JavaScript To Move Mouse Pointer to a Specific Position
Let’s now implement the functionality for it using JavaScript. First, we will grab all the elements cursor, btn1
and btn2
, using the getElementById()
method inside the JavaScript.
Since we want to move the mouse cursor to a specific position, in this case, on button 2
, we first have to grab the positions (left, top, width, height) of button 2
so that when we click on button 1
, the mouse pointer will automatically move to button 2
.
For this, we will use the getBoundingClientRect()
method on the btn2
, which will provide us with an object that contains various positions and dimensions like left, top, bottom, width, height, etc., and we will store this object inside a variable called rect
. Then we will calculate the finalPositionX
and finalPositionY
of button 2
, so we can take the mouse pointer to the center of button 2
when clicking button 1
.
Our custom mouse pointer does not move on the screen at this position. To make it move freely on the browser, we must add an event listener to the window object.
This method takes two parameters, the first is the type of event on which we want to execute a function, and the second is the function itself that will be executed when that event is triggered.
Since we want to move our custom mouse pointer, we will use the mousemove
event as our first parameter, and then inside our callback function, we will be grabbing the clientX
and clientY
coordinates, and then we will be assigning it to the left
and top
position of our custom mouse pointer as shown below.
Don’t forget to add "px"
at the end of it; otherwise, it won’t work.
Code Snippet - JavaScript:
let cursor = document.getElementById('cursor');
let btn1 = document.getElementById('btn1');
let btn2 = document.getElementById('btn2');
let rect = btn2.getBoundingClientRect();
let finalPositionX = rect.left + rect.width / 2;
let finalPositionY = rect.top + rect.height / 2;
// Moving our custom mouse pointer
window.addEventListener('mousemove', (e) => {
x = e.clientX;
y = e.clientY;
cursor.style.left = x + 'px';
cursor.style.top = y + 'px';
});
btn1.addEventListener('mouseup', (e) => {
cursor.style.left = finalPositionX + 'px';
cursor.style.top = finalPositionY + 'px';
})
At this stage, the custom mouser cursor will move as the normal mouse cursor. Now we will be moving the custom mouse cursor to a specific position.
For that, we will add a mouseup
event on the btn1
and then set the mouse cursors left
and top
positions with the finalPositionX
and finalPositionY
positions.
Output:
Conclusion
There is no direct way of changing mouse position using JavaScript, as it has some downfalls. But there are always different and unofficial ways of doing things in programming.
To solve the problem of moving the mouse pointer to a specific position, we have to hide the original mouse pointer. Then, we have two create our custom mouse pointer and write some JavaScript code to make it move to a specific position.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn