How to Loop Through Dictionary in JavaScript
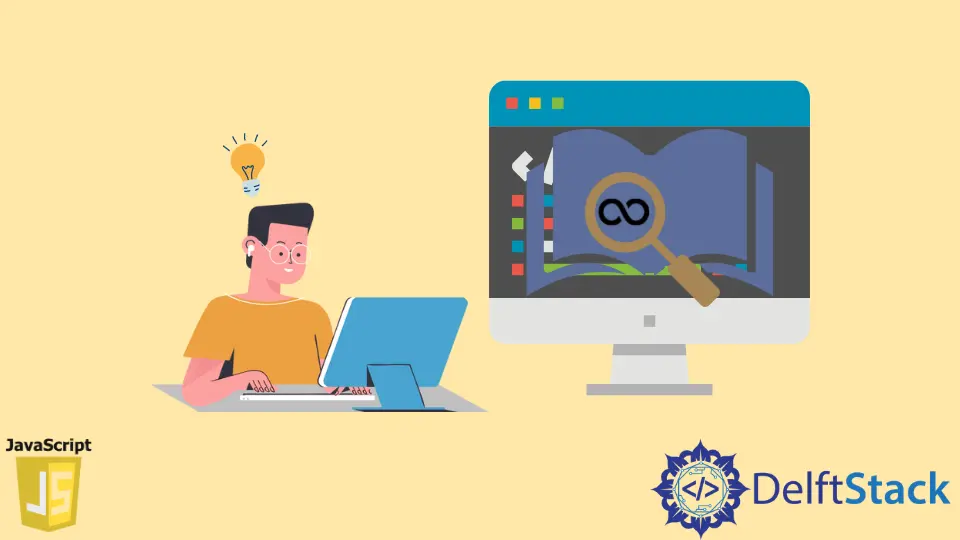
JavaScript objects are very flexible and can be used to create key-value pairs. These objects work similarly to Python dictionaries.
Dictionaries and objects are widely used because each stored value has its unique key (similar to JavaScript Array), and those values can be accessed through those keys. This allows excellent flexibility in reading and storing data.
Today’s post will teach us how to iterate the object or dictionary to extract the key-value pairs in JavaScript.
Loop Through Dictionary in JavaScript Using Object.entries()
We can return an array of [key, value]
pairs of string-key enumerable properties of a given object using the Object.entries()
method.
This is similar to iterating with a for...in
loop. However, this loop enumerates properties in the prototype chain.
Its order of the array returned is the same as that returned by a for...in
loop. An enumerable property in JavaScript means that a property is visible when iterated using the for...in
loop or the Object.keys()
method.
By default, all properties created by a simple assignment or property initializer are enumerable.
Syntax:
Object.entries(obj)
The Object.entries
method accepts an object as an argument, a required parameter.
You can find more information about Object.entries()
in this documentation.
Let’s take an example of an employee object. This object contains basic employee details.
When you iterate over an employee object, it will print all its enumerable properties.
const object = {
employeeName: 'John Doe',
employeeId: 27,
salary: {
'2018 - 19': '400000INR',
'2019 - 20': '500000INR',
'2020 - 21': '650000INR'
},
}
Object.defineProperty(student, 'address', {
value: 'India',
configurable: true,
writable: false,
enumerable: false,
});
for (const [key, value] of Object.entries(object)) {
console.log(key, value);
}
We have added the new address
property in the above example, but its enumerable type is set to false
. Since the Object.entries()
method only iterate through enumerable properties, it will not print the address property.
Try to run the above example in any browser. You will see the following result.
Output:
"employeeName", "John Doe"
"employeeId", 27
"salary", {
2018-19: "400000INR",
2019-20: "500000INR",
2020-21: "650000INR"
}
You may also access the complete example given above here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn