How to Get Dictionary Length in JavaScript
-
Use the
Object.keys(dict).length
Method to Find the Length of the Dictionary in JavaScript -
Use the
for
Loop to Find the Length of the Dictionary in JavaScript
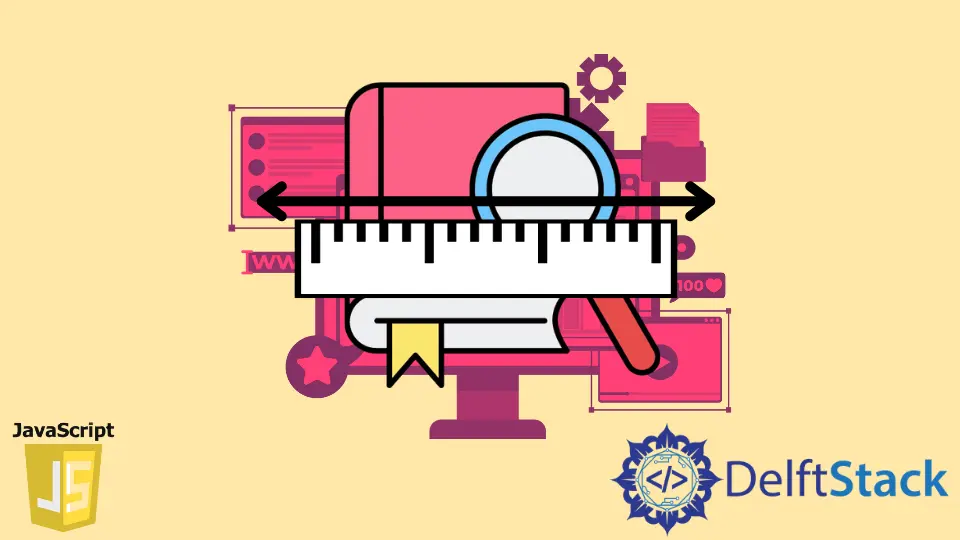
As JavaScript is a dynamic language, the objects are pretty flexible and can be used to create dictionaries.
We can create key-pair values in JavaScript by using objects. These key-pair values work like dictionaries in JavaScript.
Use the Object.keys(dict).length
Method to Find the Length of the Dictionary in JavaScript
The Object.keys(dict).length
method returns the total number of elements stored in a dictionary or an object.
We use this method to find the total number of elements in a dictionary in JavaScript.
We will create a dictionary dict
by using the object. We stored some data inside the dictionary.
let dict = {
name: 'Abid',
age: 24,
profession: 'Software Engineer',
job: 'Freelancer',
counrty: 'Pakistan'
} console.log('The length of the Dictionary = ' + Object.keys(dict).length);
The numbers of items stored in the dictionary dict
are five.
Output:
The length of the Dictionary = 5
So, using object.keys(dict).length
in console.log will return the length of the dictionary. In the output, we get 5
as the length of the dictionary.
Use the for
Loop to Find the Length of the Dictionary in JavaScript
We can find the length of a dictionary manually by using the for
loop. We will create a dictionary named data.
We store the data in the dictionary. As we can see, there are five pair-key values stored in the dictionary.
We display the count in coonsole.log
, which will give us the total length of the dictionary.
var data = {
Name: 'Abid',
age: 24,
profession: 'Software Engineer',
job: 'Freelancing',
country: 'Pakistan'
};
var count = 0;
for (var i in data) {
if (data.hasOwnProperty(i)) count++;
}
console.log('The length of the Dictionary = ' + count);
Output:
The length of the Dictionary = 5
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn