How to Specify Multiple Conditions Inside the if Statement in JavaScript
-
Using Logical Operators to Add Multiply Conditions Inside the
if
Statement in JavaScript -
Using AND and OR Operators in Combination Inside the
if
Statement in JavaScript
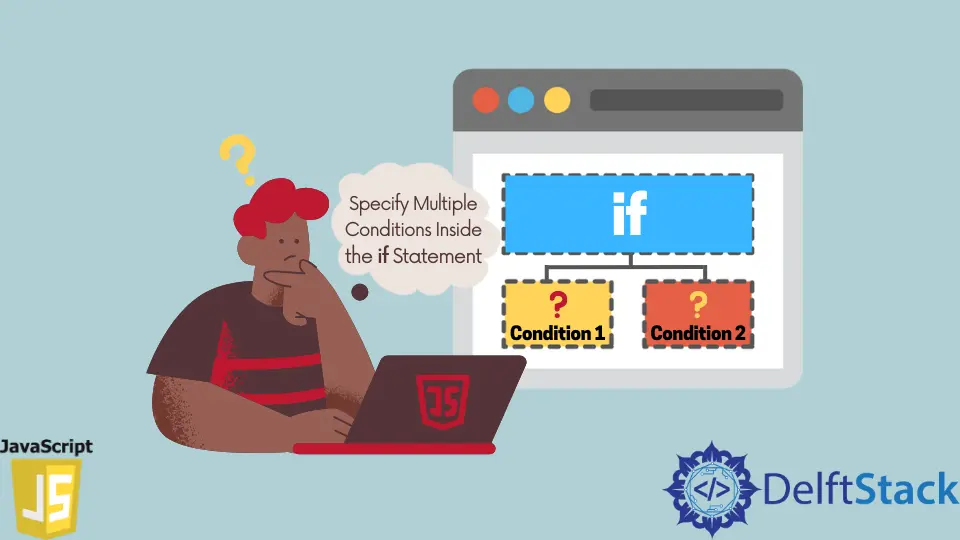
In programming, if we want to execute a code when a particular condition or conditions are satisfied, then in such cases, we make use of something called if
statements. Let’s say we have an array of integers, and we want to check whether the number say 20
is present inside the array or not. We do this by adding a condition inside the if
statement as follows.
var arr = [5, 25, 70, 62, 20, 89];
arr.forEach(num => {
if (num == 20) {
// do something
}
})
This is an example where we have only added a single condition inside the if
statement. But there are situations while writing production-ready software where we have to pass multiple conditions to the if
statements. And based on the result of the condition (true or false), we can execute the appropriate code. Now let us understand how to write an if
statement that contains multiple conditions.
Using Logical Operators to Add Multiply Conditions Inside the if
Statement in JavaScript
To add various conditions inside the if
statement, we make use of logical operators. There are four logical operators in JavaScript, out of which we will use two logical operators AND (&&
) and OR (||
). These two operators will help us to separate the different conditions inside the if
statement. Let’s see how these operators work and how to use them.
- AND (
&&
)
The AND (&&
) operator works in this way if you have many conditions, and if any one of the conditions is false
, the overall result will be false
. For more information, please see the truth table below.
Truth table for AND operator:
A |
B |
A && B |
---|---|---|
True |
True |
True |
True |
False |
False |
False |
True |
False |
False |
False |
False |
Example:
var arr = [5, 25, 70, 62, 20, 89];
arr.forEach(num => {
if (num == 20 && num % 2 == 0) {
console.log(num);
}
})
Output:
20
In this example, we have two conditions inside the if
statement. The first condition checks whether the current number of the array is 20
or not. If the condition holds, then it will return true
and false
otherwise. The second condition checks whether the current number of the array is even or not. And if that’s correct, then it will be true
and false
otherwise.
In this case, since we are using the &&
operator, both conditions inside the if
statements must hold to enter inside the if
statement. Here, only for number 20
of the array, both of these conditions will be true because the number 20
equals 20
, and also 20%2
will give us 0
as the output.
- OR (
||
)
The OR (||
) operator works in this way if you have many conditions, and if any one of the conditions is true
, the overall result will be true
.
The truth table for OR operator:
| A
| B
| A || B
|
| ——- | ——- | :—–: |
| True
| True
| True
|
| True
| False
| True
|
| False
| True
| True
|
| False
| False
| False
|
Example:
var arr = [5, 25, 70, 62, 20, 89];
arr.forEach(num => {
if (num == 20 || num % 2 == 0) {
console.log(num);
}
})
Output:
70
62
20
For OR operator, we have taken the same example we have seen for the AND operator, but instead of the &&
, we have used the ||
operator. Notice how the output has changed just by changing the operator. This is because the second operator is becoming true
three times when the number is 70
, 62
, and 20
.
Using AND and OR Operators in Combination Inside the if
Statement in JavaScript
There are some situations where we have to use these operators in combination inside the if
statement. The below example illustrates the same.
var arr = [5, 25, 70, 62, 20, 89];
arr.forEach(num => {
if ((num != 20 && num % 2 == 0) || (num > 50) && (num < 100)) {
console.log(num);
}
})
Output:
70
62
89
Here, we have three conditions inside the if
statement. The first condition is a combination of two conditions in itself. In order for the first condition to be true
the inner two conditions num != 20
and num%2 == 0
must also be true since there is an &&
operator. Then we have the second condition to check whether the number is greater than 50
or not. And the third condition is checking whether the number is less than 100
or not.
Notice that there is a ||
operator between the first and second condition and a &&
operator between the second and third. According to the precedence of the operators, the &&
operator has higher precedence than the ||
operator. Therefore, the second and the third conditions will be evaluated first, and then the result of this will be evaluated with the first condition. To learn more about operator precedence, you can visit this MDN docs.
Apart from just using a single if
statement, we can also make use of something called an if and else if
statement. This allows us to add multiple if
statements, each with different conditions, and each of these code blocks can execute different code. The code snippet below shows this.
var arr = [5, 25, 70, 62, 20, 89];
arr.forEach(num => {
if ((num > 50) && (num < 100)) {
console.log('The number is in the range of 20 to 100.');
} else if (num != 20 && num % 2 == 0) {
console.log('The number 20 is divisible by 2.')
} else if ((num < 50) || (num == 5)) {
console.log('The number is less than 50.')
}
})
Output:
The number is less than 50.
The number is less than 50.
The number is in the range of 20 to 100.
The number is in the range of 20 to 100.
The number is less than 50.
The number is in the range of 20 to 100.
Here, we have added if
and various else if
statements, each containing multiple conditions. Now depending upon the number present inside the array, any one of the three conditions will be satisfied, and the code inside that particular code block will be executed.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn