How to Hide/Show Elements in JavaScript
-
Use the
style.visibility
Property to Hide/Show HTML Elements -
Use the
style.display
Property to Hide/Show HTML Elements -
Use jQuery’s
hide()
/show()
to Hide/Show HTML Elements -
Use jQuery
toggle()
to Hide/Show HTML Elements -
Use
addClass()
/removeClass()
to Hide/Show HTML Elements
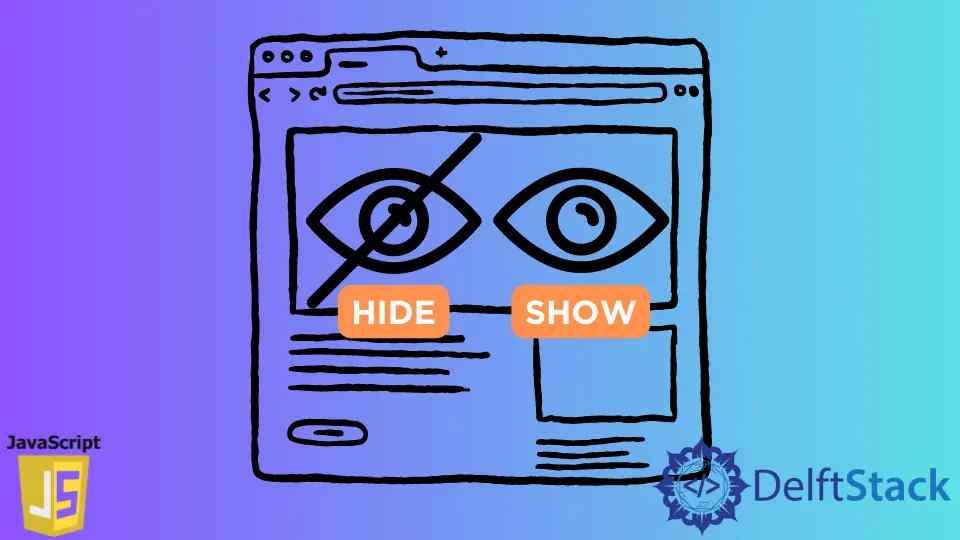
We often come across situations where we want to toggle between displaying and hiding an element.
This tutorial introduces how to hide/show an element in JavaScript.
Use the style.visibility
Property to Hide/Show HTML Elements
The style.visibility
property, when set to hidden, makes the target element hidden, but it does not remove it from the flow. So, the target element is rendered but not visible. It does not affect the layout and allows other elements to occupy their natural space. We can make the target element visible again by setting the property back to visible
.
document.getElementById(id).style.visibility = 'visible'; // show
document.getElementById(id).style.visibility = 'hidden'; // hide
Use the style.display
Property to Hide/Show HTML Elements
The style.display
property, when set to none
, removes the target element from the normal flow of the page and allows the rest of the elements to occupy its space. Although the target element is not visible on the page, we can still interact with it through DOM. All the descendants are affected and are not displayed just like the parent element. We can make the target element visible again by setting the property to block
. It is advisable to set display
as ''
because block
adds a margin to an element.
document.getElementById(id).style.display = 'none'; // hide
document.getElementById(id).style.display = ''; // show
Use jQuery’s hide()
/ show()
to Hide/Show HTML Elements
The show()
method helps us to display elements hidden through display:none
or jQuery hide()
method. It is not able to display elements that have their visibility hidden. It accepts the following three parameters:
Speed
: It specifies the speed of delay of fading effect.Easing
: It specifies the easing function used for transitioning to a visible/hidden state. It takes two different values:swing
andlinear
.Callback
: It is a function executed after the execution of theshow()
method is completed.
Similarly, the jQuery hide()
method helps to hide the selected elements. It takes the same 3
parameters as show()
.
$('#element').hide(); // hide
$('#element').show(); // show
Use jQuery toggle()
to Hide/Show HTML Elements
The jQuery toggle()
is a special method that allows us to toggle between hide()
and show()
method. It helps to make hidden elements visible and visible elements hidden. It also takes the same three parameters as jQuery’s hide()
and show()
methods. It also takes a 4th parameter display which helps to toggle the hide/show effect. It is a boolean parameter that, when set to false, hides the element.
$('div.d1').toggle(500, swing); // toggle hide and show
Use addClass()
/removeClass()
to Hide/Show HTML Elements
The addClass()
function helps us to add a class to the existing class list of an element, and removeClass()
helps us to remove it. We can use these functions to toggle hide/show by writing a custom class that hides element and then adding and removing it from the class list.
.hidden{display: none} $('div').addClass('hidden'); // hide
$('div').removeClass('hidden'); // show
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn