Hashmap Eqivalent in JavaScript
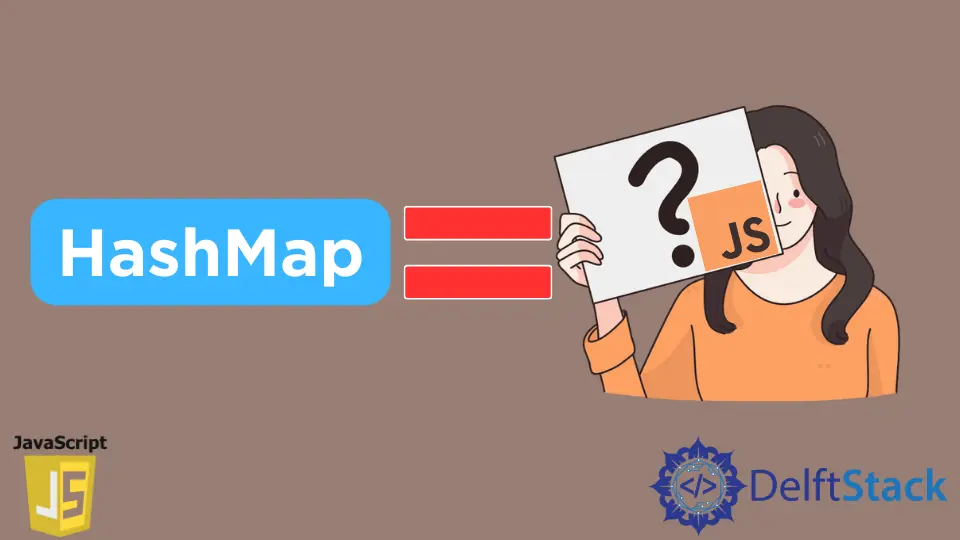
Hashmap, also known as Hash Table, is a collection of elements where elements are stored in the form of a key-value pair. There was no predefined data structure available for hashmap implemented in the JavaScript programming language back in the day. Not like other programming languages like C++ or Java, where this data structure is already implementing. Because of which people used to search for various alternatives of implementing hashmap, and most of the time, they were used to use objects because they also have the structure of key-value.
In the ES6 version of JavaScript, the JavaScript community introduces a new data structure for implementing a hashmap called a Map
object, which is a key-value pair. Let’s see how to implement a hashmap using the Map
object and perform various operations like insert, delete, and update using some predefined methods.
Implementing Hashmap Using the Map
Object in JavaScript
The Map
object, as we have already seen, is a key-value pair. Initially, the Map will be empty; there will be no elements inside it. The key in a hashmap will be either of type string or symbol and the value of the hashmap can be of any type.
To create a Map
, you can create an object of it and store it inside a variable, in this case, hashmap
. Now to insert key-value pairs inside the hashmap, JavaScript provides a method called set()
. This method takes two parameters, the first is the key, and the second is the value. Inside the hashmap
which we have created, we will insert four elements, each of them will have a value of a different type like integer, array, string, and an entire function. Below is the code which demonstrates the same.
var hashmap = new Map();
hashmap.set('1', 700);
hashmap.set('2', [1, 2, 3]);
hashmap.set('3', 'This is a string');
hashmap.set('4', () => {console.log('Hello World')});
console.log(hashmap);
After you run the above code, this is how it will look like in the console.
As you can see, we have inserted various data types, including an entire function as a value to the hashmap.
We have created a hashmap; let’s try printing all the values present inside our hashmap. It can be done with the help of the forEach
loop.
hashmap.forEach(element => {
document.write('<br>' + element);
});
The output of this will be directly on the screen rather than inside the console using the document.write()
method.
Now let’s check if the key ‘3’ is present inside the hashmap or not using the has()
method. If the key is present inside the hashmap, we will change or replace the existing value of the key ‘3’ using the set()
method.
if (hashmap.has('3')) {
hashmap.set('3', 'We changed the value...')
}
console.log(hashmap);
Output:
Finally, to delete an element from the hashmap, you can use the delete()
method and pass the appropriate key which you want to delete. And if you want to remove all the elements from the hashmap at once and make it empty, you can clear()
method as follows.
// deletes 4th element
hashmap.delete('4');
console.log(hashmap);
// clears entire hashmap
hashmap.clear();
console.log(hashmap);
The below is the output for the above code.
This is an equivalent and the right way of creating a hashmap in JavaScript.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn