How to Get href in JavaScript
- Get Href or Location in JavaScript
-
Use
getAttribute()
to Get Href in JavaScript -
Use
href
Attribute to Get Href in JavaScript
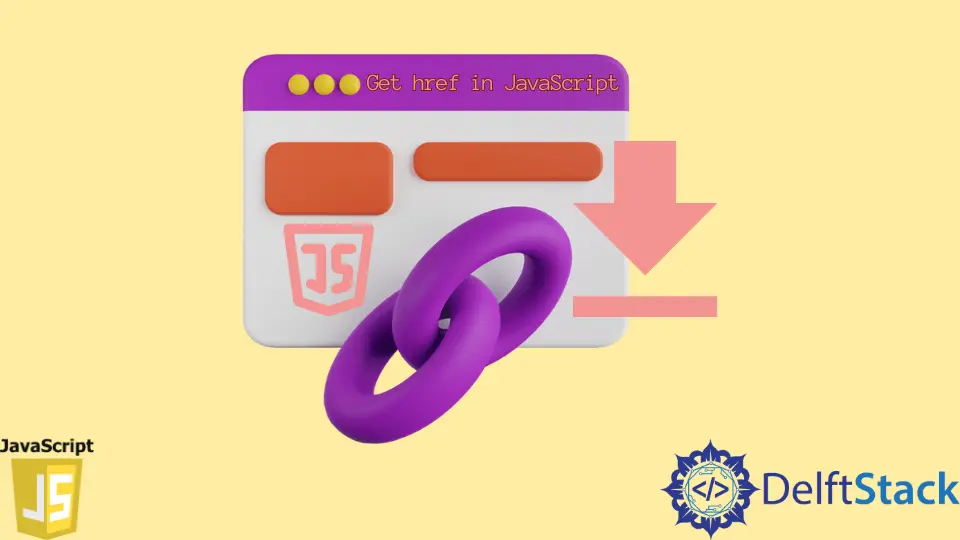
The article will teach how to get the href/location in JavaScript. The JavaScript widget offers two choices; the first uses the location attribute, and the second uses the open method.
Get Href or Location in JavaScript
The location
interface represents the location (URL) of the object to which it is linked. The Document
and Windows
interfaces have an associated location that can be accessed via document.location
or window.location
.
The biggest difference between them is their browser compatibility. The window.location
is read/written by all supported browsers.
The document.location
is read-only in IE but read/write in Gecko-based browsers like Firefox.
To communicate with the browser, JavaScript provides the main window object. Represents the browser window.
All global variables and functions become members of the widget. The window position object is used to get the URL of the current page and also change the redirect URL.
Use getAttribute()
to Get Href in JavaScript
The Element
interface’s getAttribute()
method returns the value of a specified attribute for the element. If the specified attribute does not exist, the return value is null
or " "
(the empty string).
Syntax:
const attributeOutput = element.getAttribute(attributeName);
The attributeName
is the attribute’s name whose value you want to retrieve. It returns the string containing the value of attributeName
.
Further information on the getAttribute
function can be found in the documentation for the getAttribute
.
<a id="google" href="https://www.google.com"></a>
<a id="local" href="aboutUs"></a>
const value1 = document.getElementById('google').getAttribute('href');
const value2 = document.getElementById('local').getAttribute('href');
console.log(value1);
console.log(value2);
In the above code, we use the element’s getAttribute
method to fetch the value of a select
attribute associated with the requested element. Once you run the above code in any browser, it’ll print something like this.
Output:
"https://www.google.com"
"aboutUs"
Use href
Attribute to Get Href in JavaScript
The HTMLAnchorElement.href
property is a stringifier that returns a USVString
containing the full URL and allows the href to be updated.
The sole difference between the getAttribute()
and href
attribute is that prior returns the value of the anchor
element. In contrast, the latter returns the full path where the anchor
element points.
Syntax:
// Getting href
string = anchorElement.href;
// Setting href
anchorElement.href = string;
Example:
<a id="google" href="https://www.google.com"></a>
<a id="local" href="aboutUs"></a>
const value1 = document.getElementById('google').href;
const value2 = document.getElementById('local').href;
console.log(value1);
console.log(value2);
In the above code, we use the href
attribute of the anchor
element, which will give the full path where the anchor
element points. Once you run the above code in any browser, it’ll print something like this.
Output:
"https://www.google.com/"
"https://fiddle.jshell.net/_display/aboutUs"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn