Href # vs JavaScript Void
-
When to Use
Href
#
for JavaScript Links -
When to Use
Href
javascript:void(0)
for JavaScript Links -
a Better Approach to
Href
#
andjavascript:void()
in JavaScript - Conclusion
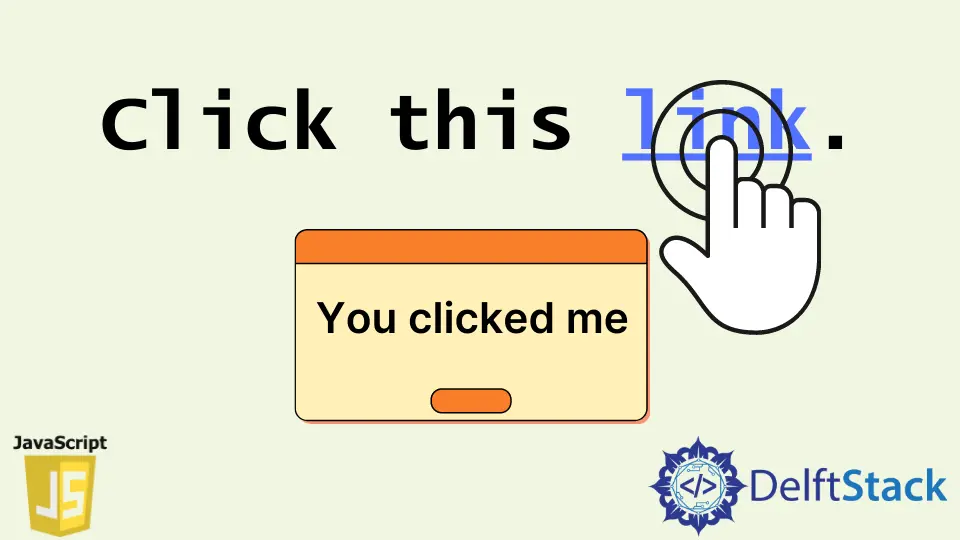
This article will explain when to use the #
and javascript:void(0)
for JavaScript links in href
. Therefore, you’ll be in a better position to decide which one is best for your use case.
At the end of this article, we’ll explain a better approach to using JavaScript links.
When to Use Href
#
for JavaScript Links
You can use href
#
for JavaScript links if you do the following.
Return false
in theonclick
event handler.- Ensure there is no error in the function that
onclick
invokes.
Return False
in the Onclick
Event Handler in JavaScript
If there is no return false
statement in onclick
, the link will take the user to the top of the page. This happens after the function execution in onclick
.
We have an HTML link with its href
value set to #
in the following code. Meanwhile, the link has an onclick
event attribute that calls on the clickMe
function.
The code will run when you click on the link to execute the clickMe
function. However, the page will jump to the top afterward.
<body>
<main style="font-size: 5em;">
<p>This is a large dummy text.</p>
<a href="#" onclick="clickMe();">Run some JS code.</a>
</main>
<script type="text/javascript">
function clickMe() {
alert("You clicked me");
}
</script>
</body>
Output:
You can fix this behavior by writing return false
in the onclick
event attribute. Therefore, after the function execution, the link will not jump to the top of the page.
The following is the updated code.
<body>
<main style="font-size: 5em;">
<p>This is a large dummy text.</p>
<a href="#" onclick="clickMe(); return false;">Run some JS code.</a>
</main>
<script type="text/javascript">
function clickMe() {
alert("You clicked me");
}
</script>
</body>
Output:
Ensure There Is No Error in the Function That Onclick
Invokes in JavaScript
If the function in the onclick
event attribute contains an error, it will not execute. As a result, the link will jump to the top of the page.
This also happens if you have a return false
statement in onclick
, but there is an error in the called function.
We’ve introduced a non-existence variable into the clickMe
function in the following code. So, when you click the link, the function will not execute; rather, the link will take the user to the top of the page.
That is because the non-existence variable leads to a ReferenceError
.
<body>
<main style="font-size: 5em;">
<p>This is a large dummy text.</p>
<a href="#" onclick="clickMe(); return false;">Run some JS code.</a>
</main>
<script type="text/javascript">
function clickMe() {
// We've introduced an undefined variable, so, this will lead to a reference error
alert("You clicked me" + t);
}
</script>
</body>
Output:
When to Use Href
javascript:void(0)
for JavaScript Links
You can use javascript:void(0)
for JavaScript links if you’d rather not use #
due to its limitations. However, javascript:void(0)
violates Content Security Policy on CSP-enabled HTTPS pages.
Meanwhile, with javascript:void(0)
, you do not need return false
in the onclick
event attribute. As a result, the link will not move the user to the top of the page after the function execution.
In the code below, we’ve used javascript:void(0)
as the value of the href
. When you run the code, you’ll observe that the link does not jump to the top of the page after the function execution.
<main style="font-size: 5em;">
<p>This is a large dummy text.</p>
<a href="JavaScript:Void(0)";" onclick="clickMe();">Run some JS code.</a>
</main>
<script type="text/javascript">
function clickMe() {
alert("You clicked me");
}
</script>
Output:
a Better Approach to Href
#
and javascript:void()
in JavaScript
When you find yourself in a situation where you’d want to use #
or javascript:void(0)
in href
, it’s best to use an HTML button. That is because links should navigate you to a location and not fire some JavaScript code.
Also, using a button eliminates the need for a link with its href
attribute set to #
or javascript:void(0)
. What’s more, you can make the button appear like an HTML link with little CSS.
We’ve styled the HTML button to resemble a link in the following code. Also, we’ve attached a click event via JavaScript.
So, when you click the link
, you’ll get a JavaScript alert
window.
<head>
<style type="text/css">
.btn-link {
cursor: pointer;
color: #00f;
padding: 0;
border: 0;
font: inherit;
text-decoration: underline;
background-color: transparent;
}
</style>
</head>
<body>
<main style="font-size: 2em;">
<p>This button looks like a <button type="button" class="btn-link">link.</button></p>
</main>
<script type="text/javascript">
let allBtn = document.querySelectorAll('.btn-link');
for (let btnLink of allBtn) {
btnLink.addEventListener('click', function() {
alert("You clicked me");
}, false);
}
</script>
</body>
Output:
Conclusion
You should favor javascript:void(0)
over #
due to the unwanted navigation. The unwanted navigation happens when there is no return false
in onclick
.
Alternatively, you can use a button and make it look like an HTML link.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn