How to Check for a Hash in a URL in JavaScript
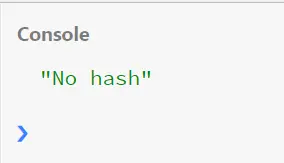
In JavaScript, we have the most straightforward way to check if the URL we are working on has a hash (#
), that is, by using window.location.hash
, but this is for a dynamic purpose. It draws out the current websites’ information.
Also, we have other ways to check if any given URL consists of a hash; we take the URL as a string and check its indexes to find the hash. Again, we can use the split()
method to check if our URL has a hash.
In the following examples, we will get a preview of these along with a jQuery solution. Let’s dig in!
Check for a Hash in Any URL in JavaScript
Here, we will see two instances that will cover how we can get the presence of a hash from any predefined URL. The following contains the indexOf()
and split()
methods for solving the case.
Use indexOf
to Check for a Hash in Any URL in JavaScript
The URL we will consider has to be set as a string as the indexOf
method plays in the case of strings. So, we will set a conditional statement here to give us our desired result, and we do get the proper fit.
Let’s check the code lines.
Code Snippet:
var url = 'http://www.google.sk/foo?boo=123baz';
if (url.indexOf('#') !== -1) {
console.log('got hash')
} else {
console.log('No hash');
}
Output:
Use the split()
Method to Check for a Hash in Any URL in JavaScript
In this case, we will again consider a URL as a string. Later, we will use the split()
method to get the token if we have a hash in our case, and according to conditions, we do get a hash.
The code fence will demonstrate clearly.
Code Snippet:
var url = 'https://mail.google.com/mail/u/0/#inbox';
var parts = url.split('#');
if (parts.length > 1) {
console.log('Found hash');
}
Output:
Check for a Hash in Current URL in JavaScript
We will focus on the current website and its address. By fetching the URL, we will check the hash portion, and if we find it, this will result in an affirmation word; else, a negation.
Use window.location.hash
to Check for a Hash in Current URL in JavaScript
The basic window.location.hash
grabs the current URL and checks if there are any findings of hash. Then, we set up a condition to bring us an answer.
Code Snippet:
var hash = window.location.hash;
if (hash) {
console.log('hash found');
} else {
console.log('hash not found')
}
Output:
Use the jQuery .prop()
Method to Check for a Hash in Current URL in JavaScript
Similarly, here we used $(location)
that by default fetches the current window we are working on. Then, the .prop()
method adds up the condition to look for any available hash in the URL.
Code Snippet:
var hash = $(location).prop('hash');
if (hash) {
console.log('hash found');
} else {
console.log('no hash')
}
Output: