How to Create Counters in JavaScript
- Implement Counters Using Variables in JavaScript
- Implement Counters Using Session Storage in JavaScript
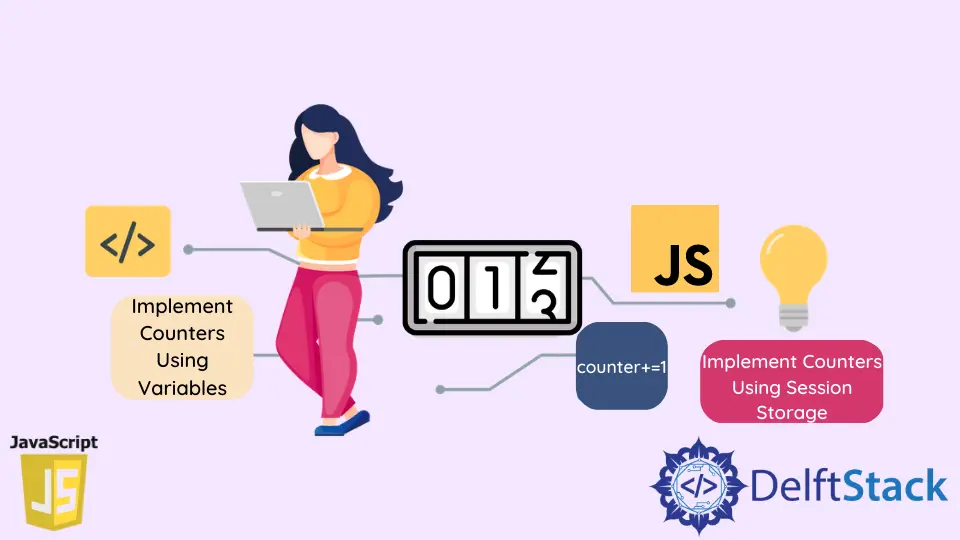
A Counter is a number that we use to count the occurrences of a particular thing. There are lots of situations where the counters can come in handy. We can implement a counter using a normal variable inside our code or using session storage.
Implement Counters Using Variables in JavaScript
The first way of implementing counters is by using variables. For example, In the below example, we have a function named value()
. Inside that function, we have a counter
variable initially set to 0
. Then we are generating a random number using the Math.random()
function. As we know that the Math.random()
function returns a floating-point value; therefore, we will be converting this floating-point value into an integer value with the help of the Math.floor()
function. After that, we will be storing the generated number inside the randomNo
variable.
function value() {
let counter = 0;
let randomNo = Math.floor(Math.random() * (10 - 0 + 1) + 0);
while (randomNo != 5) {
randomNo = Math.floor(Math.random() * (10 - 0 + 1) + 0);
counter += 1;
}
return counter;
}
console.log('Counter value is', value());
Inside the while
loop, we have a condition where we check whether the random number generated is equal to 5
or not. If that’s not the case, we will increment the counter so that the while
loop will continue to run. If the condition is false i.e the random number generated becomes equal to 5
, then the value()
function will return the value of the counter variable.
Finally, we are just printing the counter variable’s value which is returned by the value()
function as an output shown below.
Output:
Counter value is 23
Note that the above output value of the counter will vary depending upon how many iterations the number 5
is generated.
Implement Counters Using Session Storage in JavaScript
There might be a situation where we need to retain the value of a counter variable throughout the session is running in the browser or till the browser window is open. In such cases, we can make use of session storage. The session storage is used to retain the value of the counter variable for a longer time. Let’s understand this with an example.
In this example, we have a for
loop, which runs five times. And every time it runs, it calls the value()
function. Inside the value()
function, we have a counter
variable set to 0
, and then we are incrementing the value of the variable by 1
and then printing the value.
for (let i = 0; i < 5; i++) {
value();
}
function value() {
let counter = 0;
console.log('Counter Value:', counter + 1);
}
Output:
Counter Value: 1
Counter Value: 1
Counter Value: 1
Counter Value: 1
Counter Value: 1
The problem here is that every time we call the value()
function, we set the counter’s value as 0
, which we don’t want. We want to keep on incrementing its value till the loop gets executes. But because of this line of code, let counter = 0;
the value always becomes zero. Therefore, we get the output as 1 every time.
We can solve this issue by using session storage. For that, we first have to remove the counter
variable from the code and then create the same variable named counter
inside the session storage using the sessionStorage.setItem()
method as shown in the below code example.
The setItem()
method takes two parameters, key and value. Both of these parameters are strings. The key, in this case, will be counter
(you can provide any name to the key), and the value will be set to 0
.
We will also use an if
statement to check whether the counter
variable is already created inside the session storage or not. If not, then only we will create that and set its value to zero. If you don’t check prior whether the counter
variable is already created, then every time you call setItem()
it will make the counter
variable’s value to 0
, which we don’t want.
If you open the developers tools
and then click on the Application
tab, it will initially look like below.
After that, we will get the value from the session storage using the getItem()
method, which is a string. Using the Number()
method, we will convert this value into a number using the Number()
method and store it inside the counterValue
variable.
Then we will increment the value of the counterValue
variable and again store it inside the session storage. And finally, we will print the counter
value to the console window.
One thing you always have to take care of is to clear the session storage whenever the page is refreshed. To check if the page is refreshed or not, we will use the window method onunload
, and then we can use sessionStorage.clear();
to clear the values present inside session storage.
window.onunload = () => sessionStorage.clear();
for (let i = 0; i < 5; i++) {
value();
}
function value() {
if (sessionStorage.getItem('counter') == null)
sessionStorage.setItem('counter', '0');
let counterValue = Number(sessionStorage.getItem('counter'))
sessionStorage.setItem('counter', counterValue + 1);
console.log('Counter Value:', sessionStorage.getItem('counter'));
}
Output:
Counter Value: 1
Counter Value: 2
Counter Value: 3
Counter Value: 4
Counter Value: 5
If you run the above code, you will get the counter
variable’s values as expected.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn