How to Copy Text to Clipboard in JavaScript
-
Copy Text to Clipboard Using
Document.execCommand()
Method in JavaScript -
Copy Text to Clipboard Using
Clipboard API
in JavaScript
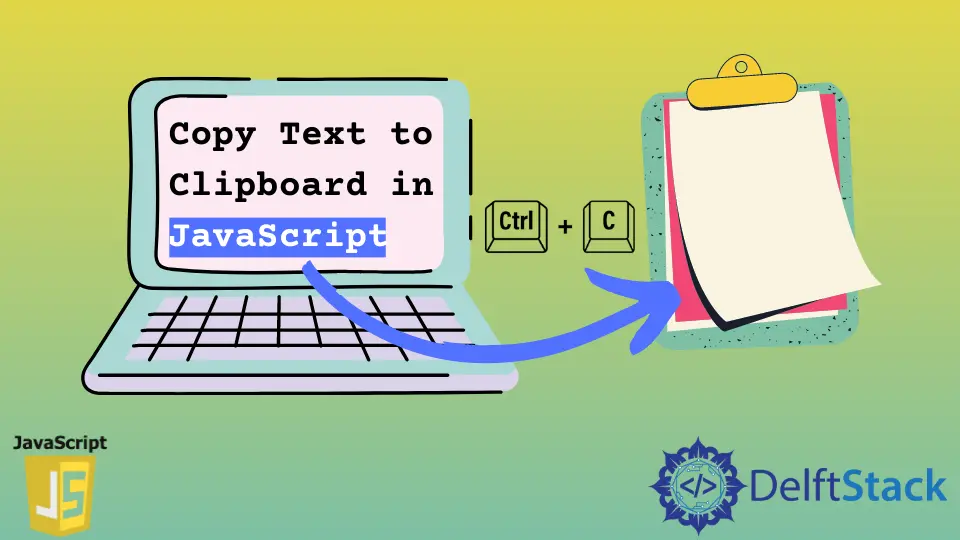
Nowadays, Many web developers want to implement a functionality on their websites that will allow their users to directly copy a bunch of text from their website with just a single click. Some of the most commonly used examples of this would be copying a terminal command or entire code snippets from any technical website.
Let’s see what do we even mean by a clipboard and how this entire process even works. After that, we will implement this copy to clipboard functionality in JavaScript.
Everything present inside our computers, like applications, images, text, files, etc., is called data. So, Whenever we try to copy anything present inside our computer, our computer will allocate a small portion of the memory to our data that we have copied to store that data inside that memory space. This is called a clipboard, and this is how the computer remembers the information which you have copied. Now that we have the data stored inside the memory, we can take this data and then paste it or use it as many times as we want.
Theoretically, it sounds simple, but practically it is difficult to implement because there are lots of complexities involved. But don’t worry, using JavaScript, we can do this thing in a much simpler way with the help of Document.execCommand()
method and Clipboard API
.
Copy Text to Clipboard Using Document.execCommand()
Method in JavaScript
This is the most popularly used method of interacting with the clipboard. Using this method, you can perform the below 3 operations.
- Copy the text to the clipboard using
Document.execCommand('copy')
- Cut text and add it to the clipboard using
Document.execCommand('cut')
- Paste the content which has already been present on the clipboard using
Document.execCommand('paste')
Notice that we have to pass in the parameter of which operation we want to perform. In this article, we will only be focusing on the copying operation and not on the other ones. Let’s take an example and understand this copy operation.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<textarea id="textArea">We are learning to copy text to clipboard in JavaScript.</textarea>
<button onclick="copyToClipBoard()">Copy</button>
<script src="link to your JS file"></script>
</body>
</html>
function copyToClipBoard() {
var content = document.getElementById('textArea');
content.select();
document.execCommand('copy');
alert('Copied!');
}
First, we have the HTML file with a textarea
containing a string and a button
. Our aim here is to copy the text present inside the textarea
when we click on the Copy
button. Secondly, we have a JS file inside which we have a function called copyToClipBoard()
. This function will be responsible for copying the text to the clipboard.
Initially, we have to get the textarea
present inside our DOM using document.getElementById
, and we will store this element inside the content
variable. Now we have the content of the textarea
inside our content
variable. To copy the text, we first have to select the entire text using the select()
method. This is similar to how to select text by using our mouse.
Using the document.execCommand('copy')
method, we will copy the selected text, and we will show an alert to the user to let him know that the text has been copied to the clipboard.
At last, we have to called the copyToClipBoard()
function on our Copy
button as an onClick
event. Now, if you open this HTML page on your browser, you will see the final results.
Copy Text to Clipboard Using Clipboard API
in JavaScript
The Clipboard API provides asynchronous read and write operations using which you can copy and paste contents to and from the clipboard. The Clipboard API is available inside the navigator.clipboard
object.
This API is a new addition to the JavaScript language, and not all browsers will be able to support it, especially the old ones. If you want to check if your browser supports this API or not, you can use the below code.
if (!navigator.clipboard) {
// Clipboard API not available
return
}
Let’s see how to use the Clipboard API to copy text to the clipboard.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<textarea id="textArea">We are learning to copy text to clipboard in JavaScript.</textarea>
<button id="btn" onclick="copyToClickBoard()">Copy</button>
<script src="link to your JS file"></script>
</body>
</html>
function copyToClickBoard() {
var content = document.getElementById('textArea').innerHTML;
navigator.clipboard.writeText(content)
.then(() => {console.log('Text copied to clipboard...')})
.catch(err => {
console.log('Something went wrong', err);
})
}
The above code is almost similar to the code which we have seen before. The only difference here is we have modified the copyToClickBoard()
function. This method will be called when we will press the Copy
button. Inside this function, we first get the text present inside the textarea
using the innerHTML
property and storing that text inside the content
variable.
The navigator.clipboard
object has two methods. One is the writeText()
method used for copying the text to the clipboard, and another is the readText()
method that will be used for reading the text present inside the clipboard pasting it. Here, we are only concerned about the writeText()
method.
Inside this method, we have to pass the variable content
, which currently holds the entire text in string format. After that, this method will take the text and will copy it onto the clipboard. If this is successful, we will show a message Text copied to clipboard...
else we will throw an error message depending upon the type of error that occurred.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn