How to Change the Text of a Div Using JavaScript
-
Change
div
Text Using theinnerHTML
Attribute -
Change the Contents of a Div With
textContent
Node Attribute -
Change the
div
Text Using thecreateTextNode
andappendChild
Functions -
Change the
div
Text Using theinnerText
Property -
Change the
div
Text Using the jQuerytext
Method - Conclusion
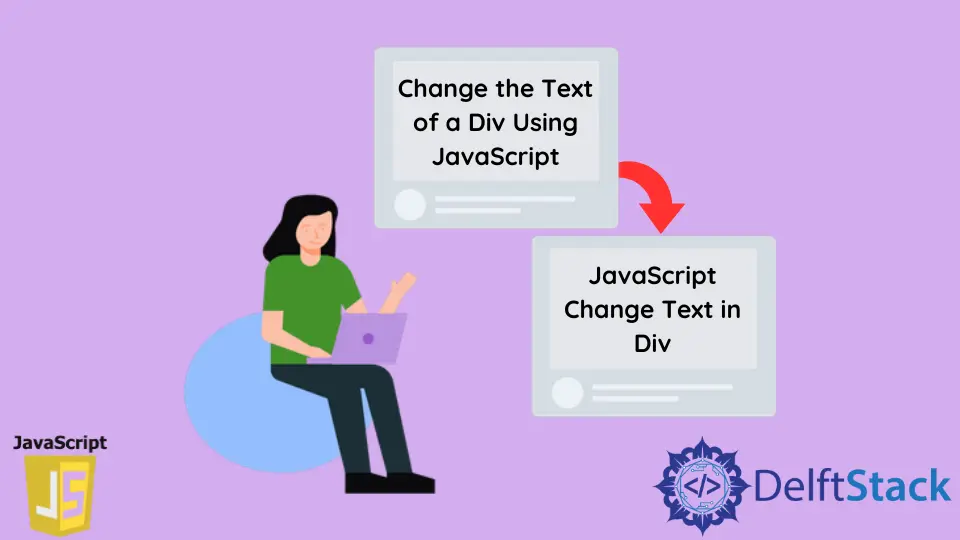
The div
elements are non-interactive by nature, and JavaScript infuses life into it by making it interactive.
Now, there might be a time when we may need to reword the text displayed by a div
. So, let us look at a few ways in which we can change the text of a div
using JavaScript.
Change div
Text Using the innerHTML
Attribute
Unlike other programming languages, we do not use getter and setter methods to set a text to HTML elements.
The HTML element object for a div
has an attribute called the innerHTML
. We can use it to get and set the content of the HTML object.
Here, the HTML object will be live. That is, it reflects any change to the element property in the browser on refresh.
The following is the syntax for using the innerHTML.
var text = htmlElement.innerHTML;
htmlElement.innerHTML = 'New Text';
In the above code snippet, we get the content of an HTML div
element using the htmlElement.innerHTML
. Here, the htmlElement
is the actual HTML object.
Similarly, we can set the content to a div
using the same attribute, the innerHTML
of the element. We just assign the text content to the innerHTML
.
Example:
<div id="div-element">Hey there!!</div>
window.onload = function() {
document.getElementById('div-element').innerHTML = 'Hello World!!';
console.log(document.getElementById('div-element').innerHTML);
var el = document.getElementById('div-element');
el.textContent = 'Hello bunny!!';
console.log(document.getElementById('div-element').innerHTML);
}
Output:
Hello World!!
Hello bunny!!
Before we apply the .innerHTML
, we need to select the HTML element. We can do it in various ways.
JavaScript has many functions that we can use to query for elements from the DOM. Following are a few of the most commonly used methods.
-
getElementById()
: This function of thedocument
interface is used to select an element from theDOM
using itsid
, specified in the HTML. The function returns the HTML object corresponding to the selected element. -
getElementsByClassName
: We can query for elements based on thecss class name
with thegetElementsByClassName
function. More than one element is returned as an array of HTML objects by this JavaScript method. -
getElementsByName
: There is another way to select the elements with thename
attribute. We specify thename
attribute for the node in HTML.This method returns an array of
HTML
objects. We can use it, especially if we are interested in selecting multiple elements with a specific style or CSS class and planning on acting upon them all in one go.
-
getElementsByTagName
: We can also select an element based on their HTML tags using thegetElementsByTagName()
function. For instance, we can perform some operations on all thediv
elements in a section ofDOM
, etc. As the name suggests, the function returns an array ofHTML
objects. -
querySelector
: JavaScriptquerySelector
function is a bit more generic and can be used to selectHTML
elements with thecss query
. The function returns the first element that satisfies the condition passed in its parameter. -
querySelectorAll
: This one is similar to thequerySelector
, with the only difference being that it will return more than one element that satisfies thecss query
format passed as a parameter to it.
Change the Contents of a Div With textContent
Node Attribute
Even though we use the innerHTML
quite often, there is a security risk involved in using it. It is related to how the innerHTML
replaces the content of the HTML
element.
The innerHTML
attribute removes all the sub HTML nodes in a single shot and adds the new content as assigned in it. The security risk comes as the innerHTML
attribute allows us to insert any piece of HTML code, even if it is a harmful code.
MDN recommends using node.textContent
for changing the text of an element. It replaces the child nodes inside the HTML with the text
we assign in the textContent
parameter. Refer to the following code to understand the usage.
<div id="div-element">Hey there!!</div>
window.onload = function() {
var el = document.getElementById('div-element');
el.textContent = 'Hello bunny!!'
}
Output:
Hello bunny!!
Here, we select the HTML element, div
, before changing the text. We use the getElementById()
function to query the element from the DOM.
We then use the textContent
to change the text of the div
. This change will not be distinguished when the screen loads.
As soon as the browser loads the webpage, the window.onload
function gets executed, and the text change will happen. So, the end-user sees the newer text always.
The change of the old text to the new is not evident on the screen load. We can also play around with the textContent
inside the setTimeOut
method to see the change.
The innerHTML
attribute differs from the textContent
. Both the attributes take text
as input, but in the innerHTML
, it is possible to add malicious code. Refer to the following code snippet.
Here, the change reflects, and the innerHTML
code will get executed. As shown in the following code snippet, on clicking the light green HTML div
element, an undesirable alert
pops up on the screen.
<div id="div-element"></div>
window.onload = function() {
var el = document.getElementById('div-element');
el.innerHTML =
`<div style="height: 20px; background: lightgreen" onclick="alert('Danger!!!')">`;
}
If we assign the same content in textContent
for the div
element, it will not render it as an HTML element. JavaScript renders the content as text on the screen.
So we can literally see the <div style="height: 20px; background: lightgreen" onclick="alert('Danger!!!')">
displayed as text on the webpage. This aspect makes code more secure and reliable.
Hence, if we intend to change the text of an HTML element, the JavaScript best practices (as supported by MDN) recommend using the textContent
instead of the innerHTML
attribute.
<div id="div-element"></div>
window.onload = function() {
var el = document.getElementById('div-element');
el.textContent =
`<div style="height: 20px; background: lightgreen" onclick="alert('Danger!!!')">`;
}
Change the div
Text Using the createTextNode
and appendChild
Functions
The createTextNode
method is a function in JavaScript that helps you create a piece of text that can be used on your web page.
Imagine you have a paragraph on your webpage, and you want to change the text inside that paragraph using JavaScript. The createTextNode
method allows you to make a new piece of text.
Here’s how it works:
-
Create a New Text Node
The
createTextNode
method is like a text factory. It helps make a new text node, which is basically a container for plain text. -
Set the Text Content
When you use
createTextNode
, you provide the actual text you want to show in yourdiv
. This could be anything from a single word to a whole sentence. -
Update the
div
ContentOnce you have this text node, you can use it to change the text inside a
div
. This is useful when you want to update what is written on your webpage without reloading the entire page.
To get a better idea of how you can use createTextNode
, a simple example can be seen below.
<div id="myDiv">Original Text</div>
function changeText() {
// Select the div element by its ID
var myDiv = document.getElementById('myDiv');
// Create a new text node with the desired text
var newText = document.createTextNode('New Text');
// Remove existing content from the div
myDiv.innerHTML = '';
// Append the new text node to the div
myDiv.appendChild(newText);
}
changeText();
In this example, the text inside the div
with the ID myDiv
would be replaced with the New Text Here!
text using the createTextNode
method. This is particularly handy when you want to dynamically update the content of a div
based on user interactions or other events in your web application.
Change the div
Text Using the innerText
Property
Another straightforward way of changing the text of the div
element is by using the innerText
property.
innerText
is a property in JavaScript that allows you to get or set the text content of an HTML element. It’s commonly used to manipulate the visible text within an element, excluding any HTML or XML tags that might be present.
When you have a div
, it may contain both text content and HTML tags. The innerText
property provides a way to access and modify only the text content, disregarding any HTML tags.
Here’s a simple example of how to use the innerText
property to change the text inside a div
:
<div id="myDiv">Original Text</div>
function changeText() {
var myDivContent = document.getElementById('myDiv').innerText;
console.log(myDivContent); // Output: Original Text
document.getElementById('myDiv').innerText = 'New Text';
}
changeText();
In the program above, the changeText
function uses document.getElementById('myDiv').innerText
to select the div with the ID myDiv
and change its text content to 'New Text'
.
When the page loads, the changeText
function is called, and the visible text inside the div
is updated to 'New Text'
.
In summary, innerText
is a handy property in JavaScript when you specifically want to work with and manipulate the plain text content of an element, making it a popular choice for tasks like changing the text of a div
on a webpage.
Using Template Literals
Changing the div
text using the innerText
property is already a great approach, but it can be enhanced further by incorporating template literals for a more dynamic and readable code structure. Below is an example of doing this.
<div id="myDiv">Original Text</div>
function changeText() {
// Select the div element by its ID
var myDiv = document.getElementById('myDiv');
// Use template literals to create dynamic text
var newText = `New Text with ${new Date().toLocaleString()}`;
// Update the div's text content using innerText and the dynamic template literal
myDiv.innerText = newText;
}
changeText();
Inside the changeText
function, we use document.getElementById('myDiv')
to select the div
element with the ID 'myDiv'
.
We employ template literals (enclosed by backticks) to create a dynamic text string. In this example, we include the text "New Text with"
and append the current date and time using new Date().toLocaleString()
.
The innerText
property is then used to update the text content of the div
to the dynamically generated string created with template literals. To showcase the text change, we call the changeText
function immediately, ensuring the update occurs as soon as the page loads.
Combining the innerText
property with template literals provides a powerful and flexible way to dynamically change the text content of a div
in JavaScript. This approach enhances code readability and allows for the easy inclusion of dynamic content using the expressive syntax of template literals.
Change the div
Text Using the jQuery text
Method
One of the methods jQuery offers for altering text content is the text
method. Changing the text of a div
using jQuery’s text
method is like using a special tool to easily swap out the words inside a box on your webpage.
Below is a simple example using the jQuery text
method that changes the text inside a div
.
<div id="myDiv">Original Text</div>
function changeText() {
// Select the div element by its ID and use the text method to change its content
$("#myDiv").text("New Text");
}
changeText();
In this example, $("#myDiv")
is pointing to the box with the ID "myDiv"
, and .text("New Text")
is the command to change the words inside that box to "New Text"
. It’s a quick and efficient way to update what’s displayed on your webpage without having to deal with a lot of complicated code.
Using jQuery’s text
method simplifies the process of changing text content in comparison to vanilla JavaScript. It provides a clean and concise way to select elements and modify their text, making it a popular choice for developers aiming for brevity and efficiency in their code.
Conclusion
We’ve explored the various methods of changing the text in a div
using JavaScript. Whether you’re just starting out or you’ve been around the coding block, being able to tweak text dynamically is a great skill to have.
From the basics like innerHTML
to the safety-conscious textContent
, we’ve covered a range of tools. We even spiced things up with template literals for a touch of creativity.
And don’t forget jQuery – it offers a sleek method called text
that makes text changes a breeze, especially if you’re into simplicity.
Now armed with these techniques, go ahead and play around with the text inside the div
on your web pages.