JavaScript console.error
- Understanding console.error
- Best Practices for Using console.error
- Common Use Cases for console.error
- Conclusion
- FAQ
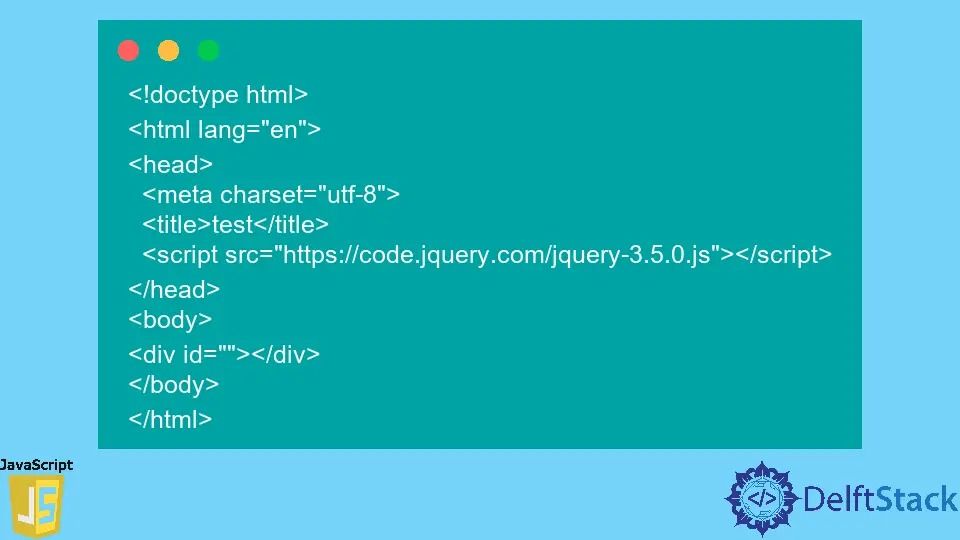
JavaScript is a versatile programming language that powers the web, allowing developers to create interactive and dynamic web applications. One of the essential tools in a JavaScript developer’s toolkit is the console, specifically the console.error
method. This function is invaluable for debugging, as it provides a way to output error messages directly to the console, helping developers quickly identify issues in their code.
In this article, we will explore the console.error
method in detail, discussing its syntax, use cases, and best practices. Whether you are a beginner or an experienced developer, understanding how to effectively use console.error
can significantly enhance your debugging process.
Understanding console.error
The console.error
method is part of the Console API in JavaScript, which provides various methods for logging messages to the web console. While console.log
is commonly used for general output, console.error
is specifically designed to highlight error messages. This distinction is crucial because it allows developers to separate regular logs from error messages, making it easier to spot issues during development.
The syntax for using console.error
is straightforward:
console.error(message);
Here, message
can be a string or any JavaScript object. When invoked, console.error
will display the message in red text in the console, making it stand out from regular log messages. This visual cue is particularly helpful when scanning through logs to identify errors.
For example, consider the following code snippet:
const userInput = null;
if (!userInput) {
console.error("User input is required.");
}
Output:
User input is required.
In this example, if the userInput
variable is null, the error message will be displayed in the console, alerting the developer to the issue.
Best Practices for Using console.error
When using console.error
, following best practices can help ensure that your debugging process is efficient and effective. Here are some tips:
-
Be Descriptive: Always provide a clear and descriptive message when using
console.error
. This will make it easier to understand the context of the error when reviewing logs later. -
Use Objects for Context: You can pass objects to
console.error
to provide additional context about the error. This is especially useful when dealing with complex data structures. -
Limit Usage in Production: While
console.error
is excellent for debugging during development, consider removing or limiting its use in production code. Excessive logging can clutter the console and impact performance. -
Combine with Other Console Methods: Use
console.error
alongside other console methods likeconsole.warn
andconsole.info
to categorize your logs effectively.
Here’s an example demonstrating these best practices:
function fetchData(url) {
fetch(url)
.then(response => {
if (!response.ok) {
console.error("Error fetching data:", { status: response.status, url });
}
return response.json();
})
.catch(error => console.error("Network error:", error));
}
Output:
Error fetching data: { status: 404, url: "https://api.example.com/data" }
In this code, if the fetch request fails, the error message includes both the status code and the URL, providing valuable context for debugging.
Common Use Cases for console.error
The console.error
method can be used in various scenarios to improve error handling and debugging. Here are some common use cases:
-
API Error Handling: When making API calls, it’s crucial to handle errors gracefully. Using
console.error
allows you to log any issues with the request, such as network errors or unsuccessful responses. -
Input Validation: When validating user input, you can use
console.error
to log any validation errors. This can help you identify issues with user data before processing it further. -
Debugging Asynchronous Code: Asynchronous operations can be tricky to debug. By logging errors with
console.error
, you can track down issues in callbacks or promises more easily.
Here’s an example of using console.error
in an API error handling scenario:
async function getUserData(userId) {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
if (!response.ok) {
console.error("Failed to fetch user data:", { userId, status: response.status });
return null;
}
return await response.json();
} catch (error) {
console.error("Network error while fetching user data:", error);
}
}
Output:
Failed to fetch user data: { userId: 123, status: 404 }
In this example, if the API call fails, the error message clearly indicates the user ID and the status code, making it easier to debug.
Conclusion
In conclusion, the console.error
method is an invaluable tool for JavaScript developers, providing a straightforward way to log error messages in the console. By using this method effectively, you can enhance your debugging process, making it easier to identify and resolve issues in your code. Remember to follow best practices, such as being descriptive and providing context, to maximize the benefits of using console.error
. As you continue your journey in JavaScript development, mastering this tool will undoubtedly improve your coding experience.
FAQ
-
What is the difference between console.log and console.error?
console.log is used for general logging, while console.error is specifically for logging error messages, which are displayed in red text in the console. -
Can I pass multiple arguments to console.error?
Yes, you can pass multiple arguments to console.error, and they will be concatenated into a single error message. -
Is console.error suitable for production code?
While console.error is great for debugging, excessive use in production code can clutter the console. It’s best to limit its use or remove it before deploying. -
How can I format error messages in console.error?
You can use template literals or string concatenation to format error messages, making them more informative and easier to read.
- Can I use console.error with objects?
Yes, you can pass objects to console.error, which can help provide additional context about the error.