JavaScript Checkbox onChange
-
Use
onChange
as Attribute on Checkbox in JavaScript -
Use
onChange
on Checkbox as JavaScript Property -
Use
addEventListener
foronChange
in JavaScript
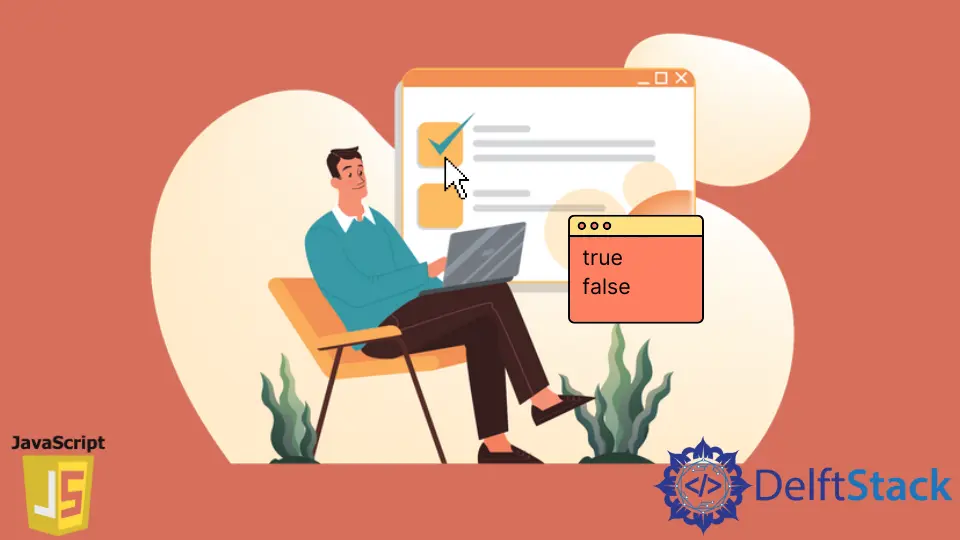
JavaScript’s onChange
event focuses on changing a value of an element. When a specific event is executed, it gets triggered and returns something of preference.
The onchange
event works just after the element loses its focus. It fires just after clicking, and the difference between onClick
and onChange
is subtle.
We will discuss the most used methods of onChange
as an HTML attribute, JavaScript property, and an event listener.
Use onChange
as Attribute on Checkbox in JavaScript
The onchange
attribute refers to a JavaScript function with a code body to trigger the system. The instance here has a checkbox, and when checked, it will run a function that will depict the change.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<label>
<input type="checkbox" onchange="Check(this)" /> CHECK as onchange attribute
</label>
<p id="verdict"></p>
<script>
function Check(value) {
document.getElementById('verdict').innerHTML = value.checked;
};
</script>
</body>
</html>
Output:
The keyword this
refers to a global object, and the onChange
attribute grabs and triggers the Check
function. The value.checked
returns a boolean output in the HTML body based on the toggle status.
Use onChange
on Checkbox as JavaScript Property
In this case, we declare an object that refers to the certain id = "check"
. After that, we will call the object.onchange
property to initialize a function that executes on toggles.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<label>
<input type="checkbox" id="check" /> CHECK for oncheck property
</label>
<p id="verdict"></p>
<script>
var y = document.getElementById('check');
y.onchange = function(value){
document.getElementById('verdict').innerHTML = y.checked;
};
</script>
</body>
</html>
Output:
Type the function(value)
for coding convenience; even if you don’t add value
as an argument, it has the same result.
Use addEventListener
for onChange
in JavaScript
The addEventListener
has a change
event that doesn’t fire until the focus is changed. The main operation calls the event listener and activates the change
event. The other function code results when the focus is lost.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<label>
<input type="checkbox" id="check" /> CHECK for addEventListener
</label>
<p id="verdict"></p>
<script>
var y = document.getElementById('check');
y.addEventListener('change', function(){
document.getElementById('verdict').innerHTML = y.checked;
});
</script>
</body>
</html>
Output:
The object is declared, and the addEventListener
is called to proceed with the other function body.