How to Check if Cookie Exists in JavaScript
- the Different Types of JavaScript Cookies
-
Create a
cookie
Using JavaScript -
Read or Check if
cookies
Exist Using JavaScript
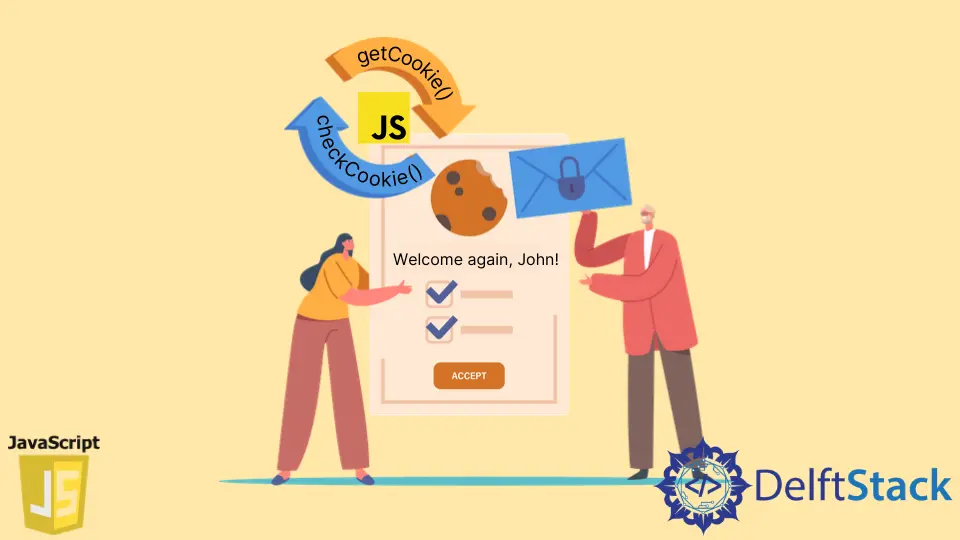
A cookie
may be a short document that stores some data on a pc approximately about 4KB. Using JavaScript, cookies will be created, retrieved, and changed and the value, name, and length of the cookie can be restricted.
This article discusses the concept of cookies
and how to check if a cookie
exists or not using JavaScript.
the Different Types of JavaScript Cookies
There are three types of cookies:
- Session cookies are stored in your browser. They are deleted once the browser is closed.
- First-party cookies are created by the website and may solely be browsed by your website.
- Third-party cookies are generated by third-party advertising on your website.
Create a cookie
Using JavaScript
You can create a cookie
of the form name=value
using the document.cookie
property provided by JavaScript that will allow you to set only one cookie at a time. With this document.cookie
property, cookies
can be set, read, and deleted.
Syntax:
document.cookie = 'UserName = HelloWorld';
Suppose you want to use special characters when creating cookies
. In that case, you need to use a Javascript-provided built-in function called encodeURIComponent()
, which encodes special characters like semicolons, spaces, and others.
Syntax - encodeURIComponent()
:
document.cookie = 'UserName=' + encodeURIComponent('Hello World!');
The default cookie lifetime is limited to the duration of the current browser session. Once the user exits the browser, it is removed.
With the max-age
attribute, you can specify in seconds how long a cookie can be stored before it is deleted from your system.
Syntax - max-age
:
document.cookie = 'Username = HelloWorld; max-age =' + 15 * 24 * 60 * 60;
This cookie
has an expiry date of 15 days.
Further information on the create cookie can be found in the documentation for the document.cookie
.
Read or Check if cookies
Exist Using JavaScript
The document.cookie
attribute returns a string containing a semicolon and a space-separated list of all cookies
. This string does not contain any cookie properties such as expiration, path, domain, etc.
To get a single cookie
from this list, use the split()
method to split it into individual name=value
pairs and then search the desired name, as shown in the following example:
function getCookie(user) {
var cookieArr = document.cookie.split(';');
for (var i = 0; i < cookieArr.length; i++) {
var cookiePair = cookieArr[i].split('=');
if (user == cookiePair[0].trim()) {
return decodeURIComponent(cookiePair[1]);
}
}
return null;
}
function checkCookie() {
var user = getCookie('username');
if (user != '') {
alert('Welcome again ' + user);
} else {
user = prompt('Please enter your name:', '');
if (user != '' && user != null) {
setCookie('username', user, 365);
}
}
}
checkCookie();
Output:
// If cookie is set
Welcome again, shraddha
In the code above, we create two functions:
getCookie()
function reads the value of a cookie.checkCookie()
function usesgetCookie()
to check whether the user name is set or not.
If configured, a welcome message is displayed. If not set, you can prompt the user name and store it in cookies.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn