How to Read Cookies in JavaScript
- Use Loop-Based Function to Read Cookies in JavaScript
- Use Regular Expression Based Function to Read Cookies in JavaScript
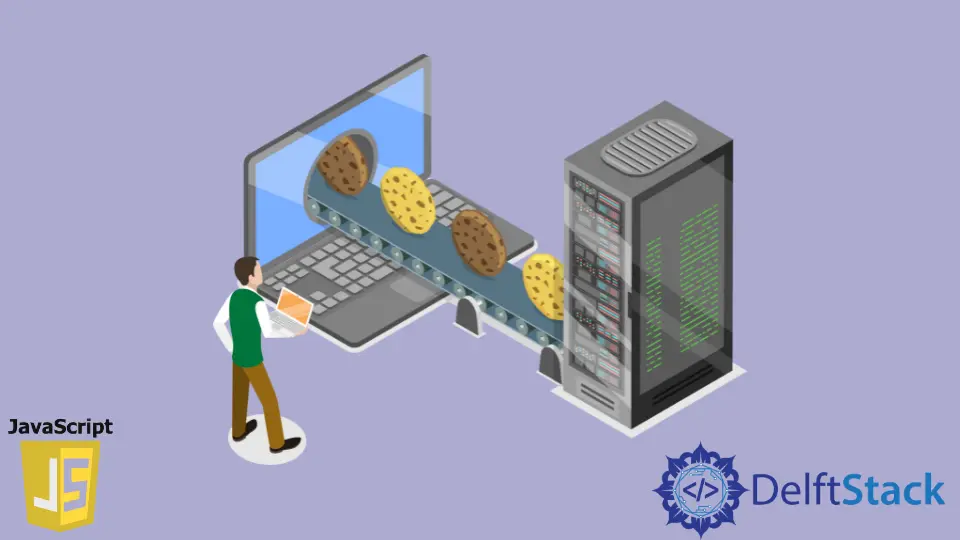
This article will tackle the function of reading a cookie in JavaScript.
Use Loop-Based Function to Read Cookies in JavaScript
The cookie is the document’s value; as a cookie object, reading a cookie is as simple as generating one. The document will keep a list of name = value
pairs separated by semicolons(;
) and the cookie
string, where name
is a cookie’s name and value
is its string value.
The split()
method for strings may separate a string into its key and values.
Example Code:
<script type="text/javascript">
function RdCookie() {
var forallcookies = document.cookie;
document.write("All Cookies : " + forallcookies);
cookiesarr = forallcookies.split(';');
for (var i = 0; i < cookiesarr.length; i++) {
name = cookiesarr[i].split('=')[0];
value = cookiesarr[i].split('=')[1];
document.write("Key is : " + name + " and Value is : " + value);
}
}
</script>
After executing this code on any online compiler, you have to save it so that it runs on the browser and you get all the cookies. Here, the Array class’s length function returns the length of an array.
Other cookies could already be installed on your computer. The code above will list every cookie placed on your computer.
Output:
All Cookies : csrftoken=qf2iNHP0TosxzZjQhSe25m9FEumZawCoL5y202yZUqkg4tRxi7Ze0sJD3u4iX1S6d0kQ%2FN4hN4ve4MuV63KdTw%3D%3DKey is : csrftoken and Value is : qf2iNHP0TosxzZjQhSe25m9FEumZawCoL5y202yZUqkg4tRxi7Ze0sJD3u4iX1S6d0kQ%2FN4hN4ve4MuV63KdTw%3D%3D
Use Regular Expression Based Function to Read Cookies in JavaScript
You may use this RegEx-based function to discover and extract the value of a cookie. If the cookie cannot be discovered (or does not have a value), an empty string " "
is returned.
Example Code:
document.cookie = 'username=hiva; SameSite=None; Secure';
document.cookie = 'favorite_food=tripe; SameSite=None; Secure';
function rdCookies(name) {
var output = document.cookie.match('(^|[^;]+)s*' + name + 's*=s*([^;]+)');
return output ? output.pop() : '';
}
console.log(rdCookies('username'));
We’re simply comparing our RegEx with the string document.cookie
and saving the result in the variable output. What is critical to understand here is the type of variables that .output()
yields.
If the RegEx matches, the output will be an array of variables. If the regex fails to match, the result will be null
.
The .output()
method will examine If the cookie we were looking for were not found within the document.cookie
(or had no value), our variable match would be null
in JavaScript.
Suppose that it was found; Our RegEx-based function will return an array variable.
Output:
"hiva"
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn