How to Change the Mouse Pointer Using JavaScript
-
Use
getElementsByTagName()
to Change Cursor Pointer in JavaScript -
Use
querySelectorAll()
to Change Cursor Pointer in JavaScript
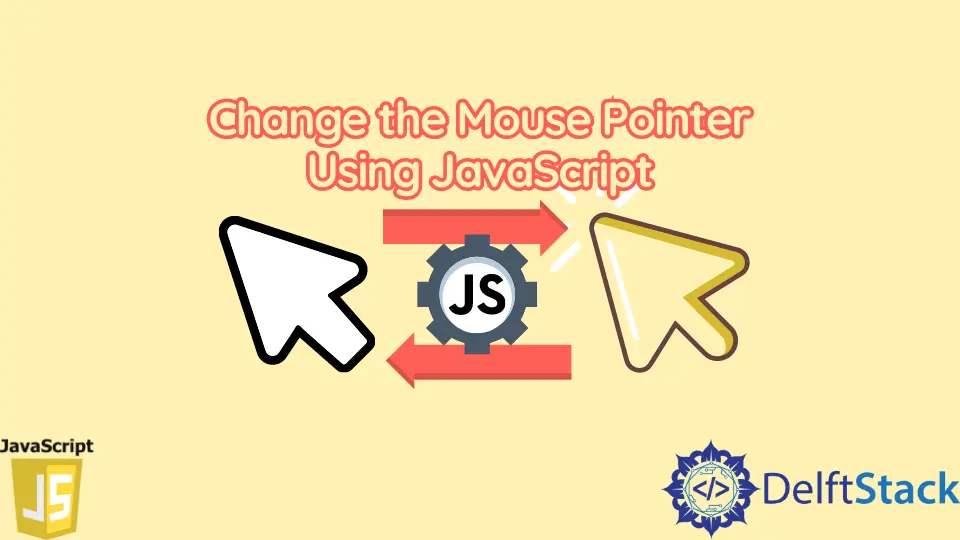
The Document Object Model (DOM) is a programming interface for Markup Language documents (HTML and XML). It defines the logical structure of documents and how to access and modify a document.
DOM presents the web page in a structured and hierarchical way to make it easier for developers and users to navigate the document.
With the DOM, we can easily access and manipulate tags, ids, classes, attributes, or elements using methods or commands provided by the Document
object.
Today’s article will teach how to change the mouse pointer using JavaScript. There are two ways to change the mouse pointer using the query selector or accessing the specific element through tags.
Use getElementsByTagName()
to Change Cursor Pointer in JavaScript
The getElementsByTagName()
is JavaScript’s built-in document
method and returns the NodeList
objects/element whose tag matches the specified tag
name.
Active HTML collections are returned, including the root node in the search.
Syntax:
document.getElementsByTagName(name);
The getElementsByTagName()
takes its name
as an input parameter. It’s a required parameter that specifies the tagName
of an HTML attribute that must match.
Returns the matching DOM element object if any matching elements are found; otherwise, it returns null
.
The only difference between getElementsByTagName
and querySelectorAll
is that it first selects only those elements whose specified tag name matches the specified tag name. In contrast, querySelectorAll
can use any selector with more flexibility and power.
The getElementsByTagName
also returns the list of active nodes, while querySelectorAll
returns the list of static nodes. The list of live nodes allows the result to be updated as soon as the DOM changes, which does not require the query to be activated.
Example:
<p>Hello World</p>
<br/>
<p>Welcome to my article</p>
const pElement = document.getElementsByTagName('p')[0];
pElement.style.cursor =
'url(\'http://wiki-devel.sugarlabs.org/images/e/e2/Arrow.cur\'), auto';
console.log(pElement.style.cursor);
We have defined two elements with a p
tag in the code above.
When we access the document with getElementsByTagName()
, it finds all nodes whose tag matches the given tag and returns NodeList
. And then set the cursor style as the requested URL.
Use querySelectorAll()
to Change Cursor Pointer in JavaScript
The querySelectorAll()
is a built-in document method provided by JavaScript that returns Element/NodeList objects whose selectors match the specified selectors.
You can change even more selectors. A syntax error is raised if an invalid selector is passed.
Syntax:
document.querySelectorAll(selectors);
See the querySelectorAll
method documentation for more information.
Example:
<p>Hello World</p>
<br/>
<p>Welcome to my article</p>
const pElement = document.querySelectorAll('p')[0];
pElement.style.cursor =
'url(\'http://wiki-devel.sugarlabs.org/images/e/e2/Arrow.cur\'), auto';
console.log(pElement.style.cursor);
We have defined two elements with a p
tag in the code above. When we access the document with querySelectorAll()
, it finds all nodes whose tag matches the given tag and returns NodeList
.
Since querySelectorAll
returns an array, we can extract the first matching element [0]
. If more than one random element is found, you can iterate on the matrix and change the cursor style for all values.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn